Understanding the Role of Call Stack in JavaScript
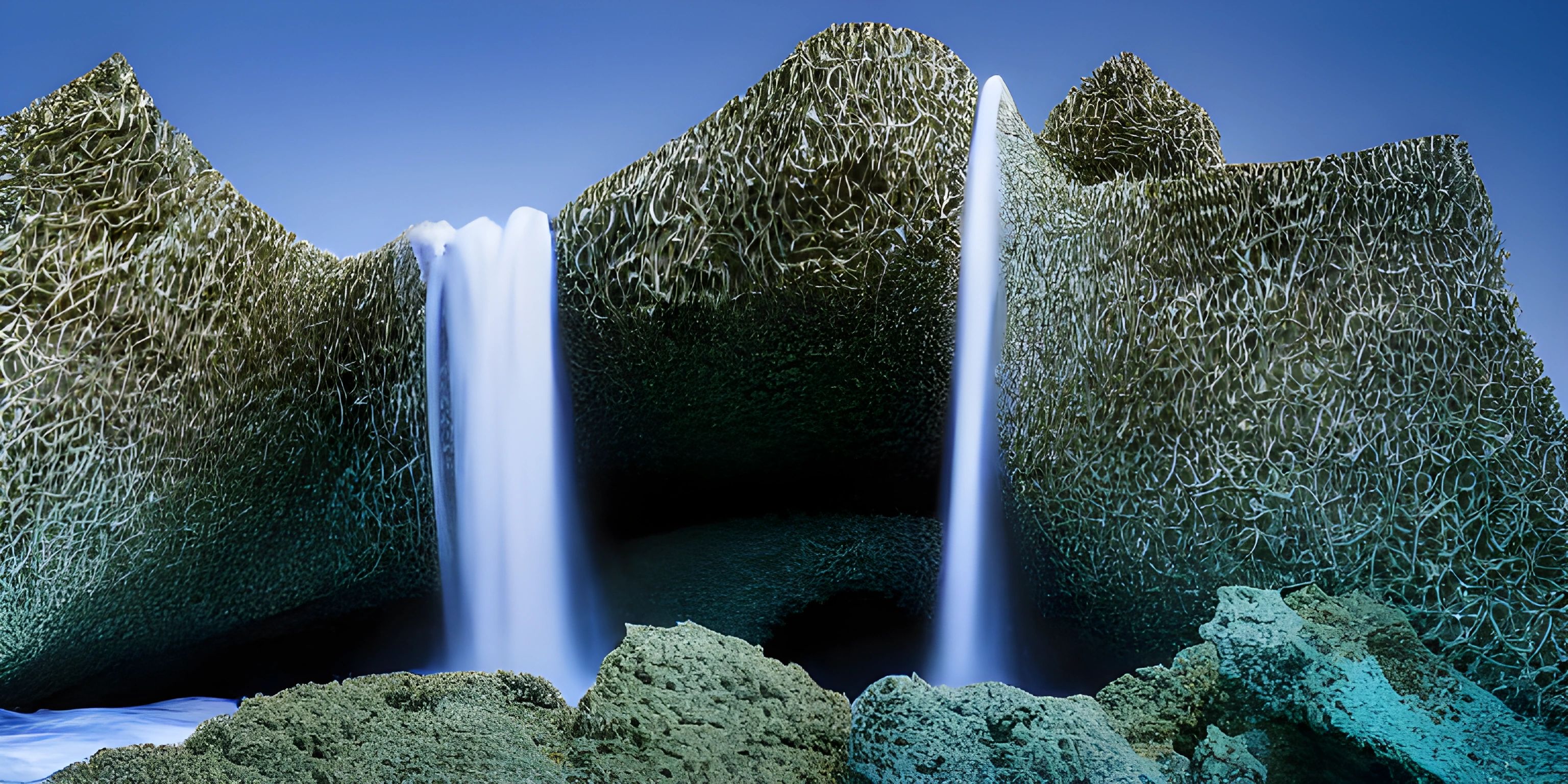
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
To brew a perfect cup of JavaScript, one must comprehend the crucial ingredients that make it so versatile and potent. One such ingredient is the call stack. It's like the barista of JavaScript, managing the execution of your function orders and ensuring they're served in the right sequence.
The Barista Analogy
Imagine walking into a café, where the barista (call stack) takes orders (function calls) from customers (code execution). The barista serves in the exact order the requests were made - the first in, first out. This is essentially how a JavaScript call stack functions. It operates on a simple principle: Last In, First Out (LIFO).
The Call Stack
The call stack, in the JavaScript context, is a data structure that records where in the program we are. If we step into a function, we put something on the top of the stack. If we return from a function, we pop off the top of the stack. That's all there is to the call stack. Here's a visual example:
function firstFunction() { secondFunction(); console.log("I am the first function!"); } function secondFunction() { thirdFunction(); console.log("I am the second function!"); } function thirdFunction() { console.log("I am the third function!"); } firstFunction();
When we run this code, our call stack will look something like this:
firstFunction
gets added to the stack.secondFunction
is called from withinfirstFunction
, so it gets added to the stack on top.thirdFunction
is called withinsecondFunction
, so it gets added to the top of the stack.thirdFunction
finishes executing and gets popped off the stack.secondFunction
then finishes and also gets popped off the stack.- Finally,
firstFunction
finishes and gets popped off.
As you can see, the call stack keeps track of function calls in our program, adding and removing them in a LIFO order.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is a call stack in JavaScript?
A call stack in JavaScript is a data structure that records where in the program we are. If we step into a function, we put something on the stack. If we return from a function, we pop off the top of the stack.
What is the principle of the call stack?
The call stack operates on a Last In, First Out (LIFO) principle. This means the last function that gets pushed onto the stack is the first one to be popped off when its execution is finished.
What happens when the call stack is full or empty?
When the call stack is empty, it signifies that the program has done executing. If the call stack gets full, it results in a Stack Overflow error. This typically happens when there is a recursive function (a function that keeps calling itself) without an exit condition.
How does the call stack handle function calls?
For every function call, it gets added (pushed) to the top of the stack. Once the function finishes execution, it is removed (popped) from the stack.
How does understanding the call stack benefit a JavaScript developer?
Understanding the call stack helps developers trace the execution order of function calls, which is crucial for debugging. It also helps in understanding concepts like recursion and closures better.