Understanding the Lerp Function in p5.js
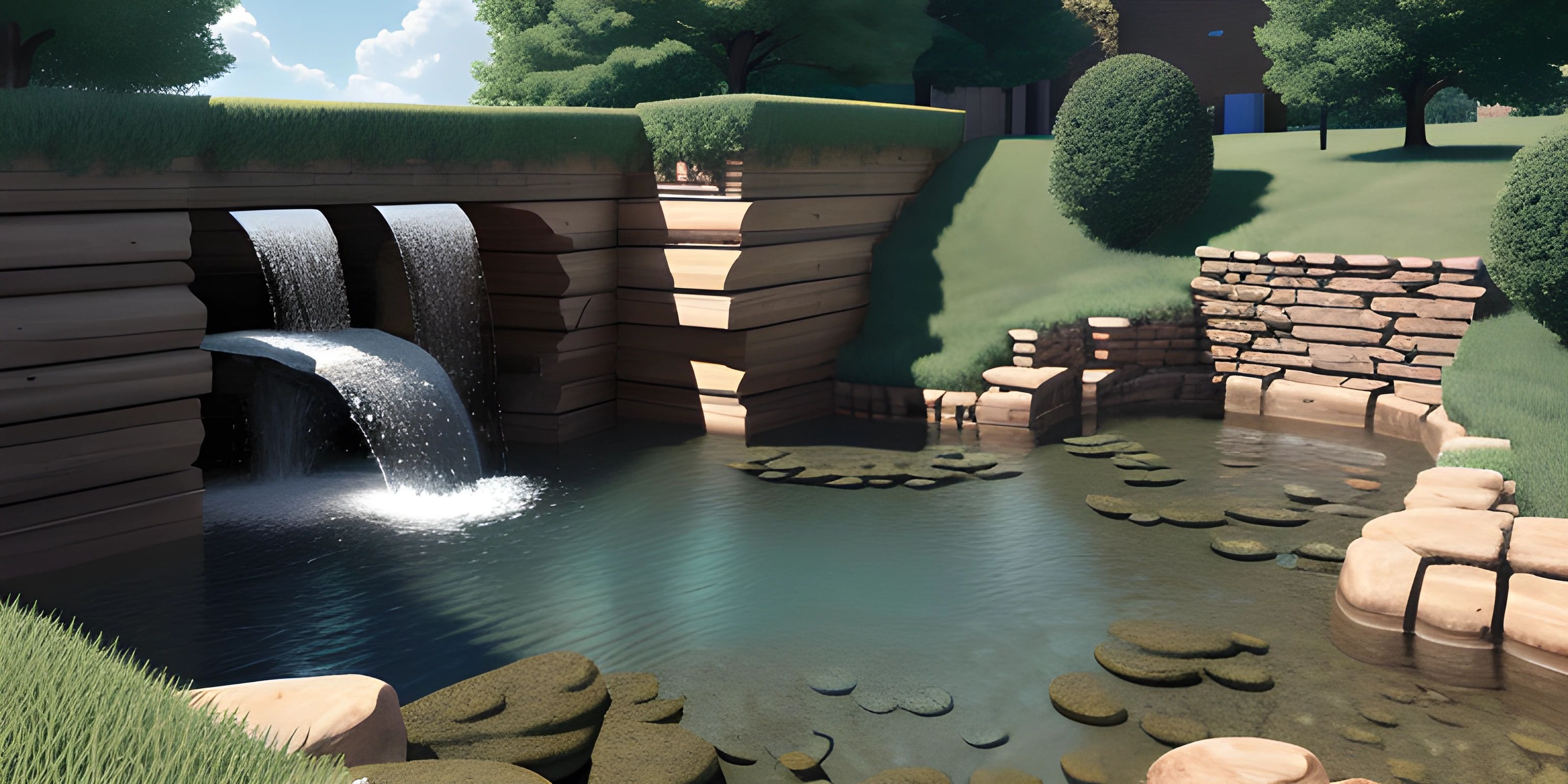
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine trying to walk a tightrope. You need to find a perfect balance between the two extremes. It's all about smooth transitions, right? Now, guess what? We have a similar concept in the world of programming – and it's called the linear interpolation, or lerp()
in p5.js lingo.
Lerp Function
The lerp function in p5.js is used to find a value between two numbers at a specific increment. It's like the bridge that takes you from one end to the other in a smooth, calculated manner.
var value = lerp(value1, value2, amount);
Here, value1
and value2
are the start and end values, and amount
is a number between 0.0 and 1.0 indicating the interpolation amount.
Using Lerp
So, how about we use this lerp() function in action? Let's say we want to animate a rectangle moving from the left side to the right side of the canvas.
var x = 0; function setup() { createCanvas(400, 400); } function draw() { background(200); x = lerp(x, mouseX, 0.1); rect(x, 200, 50, 50); }
In the above code, the rectangle starts from the left of the canvas (x = 0
). The lerp()
function then interpolates between the current x position and the mouse's x position (mouseX
), at 10% increments. This causes the rectangle to follow the mouse, but not instantly — it smoothly moves towards the mouse's position.
The p5.js lerp()
function is an incredibly useful tool in your programming arsenal, particularly when it comes to animation or gradual transitions.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Drawing Basic Shapes (psst, it's free!).
FAQ
What does the lerp function do in p5.js?
The lerp function in p5.js performs linear interpolation between two values. It calculates a new value that is a certain percentage between an initial and a final value. This can be used for smooth transitions and animations.
Can the 'amount' parameter in the lerp function be more than 1 or less than 0?
Even though the 'amount' parameter is typically between 0 and 1, it can technically be more than 1 or less than 0. This will extrapolate instead of interpolate, meaning the calculated value will be outside the range between the initial and final value.
How can the lerp function be used in animations?
In animations, the lerp function can be used to create smooth transitions. By gradually changing a value each frame, rather than jumping directly to a new value, you can create the illusion of fluid movement or gradual change, such as an object moving towards a target or a color gradually fading.
What does 'lerp' stand for?
'lerp' stands for 'linear interpolation'. It's a mathematical concept that involves calculating a new value that is a certain percentage between two other values.
Is the lerp function specific to p5.js?
No, the concept of linear interpolation and the term 'lerp' are used in many different programming languages and libraries. However, the specific implementation and usage may vary depending on the language or library.