JavaScript Recursion: Fibonacci Sequence
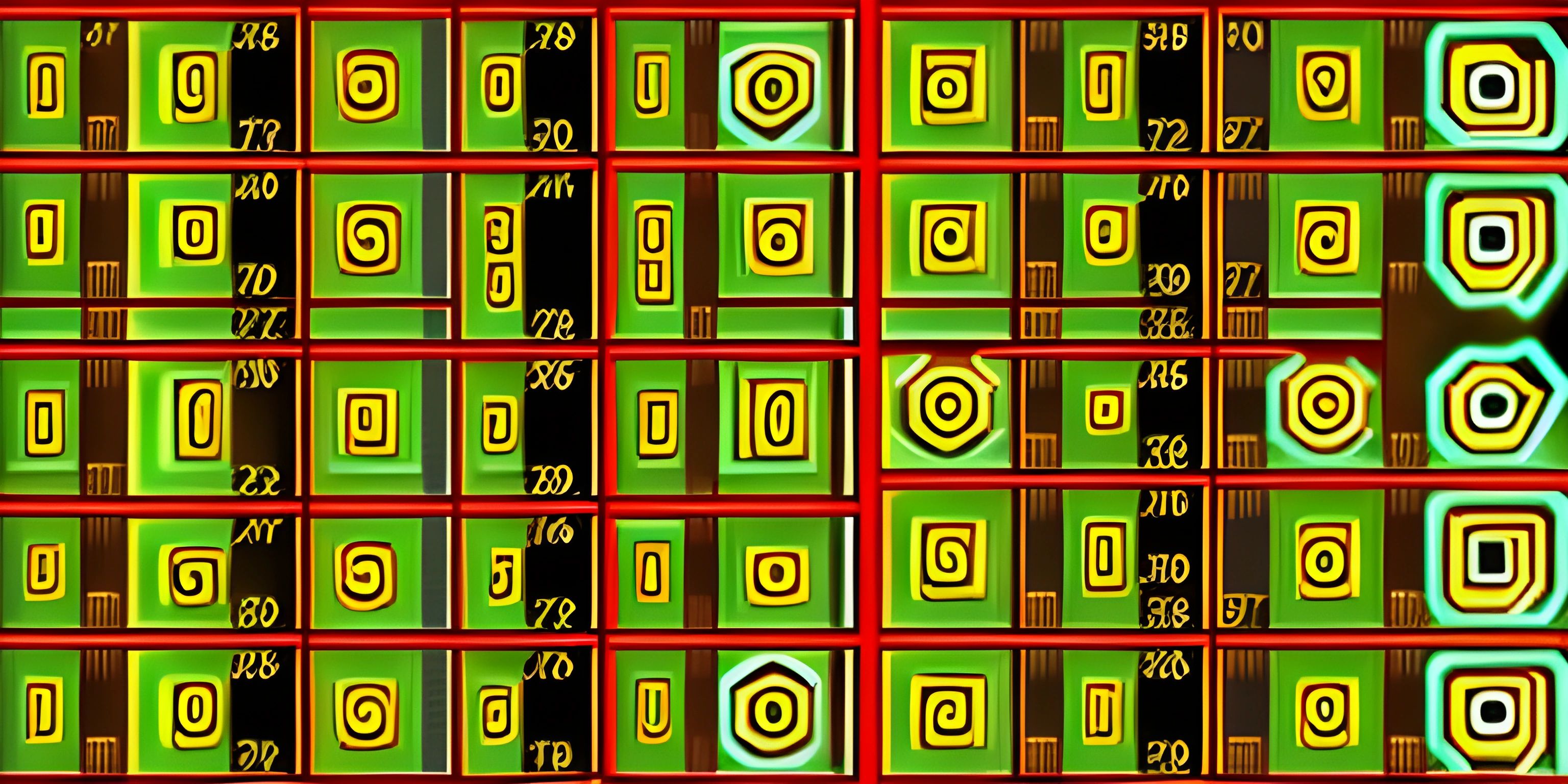
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
The Fibonacci sequence is one of those mathematical wonders that seems to pop up everywhere, from the branching of trees to the spiral of a galaxy. It's also a favorite among programmers as it provides a great opportunity to flex our recursive muscles. So, let's dive into the world of JavaScript and see how we can implement this fascinating sequence using recursive functions.
Recursive Functions
Recursive functions are functions that call themselves within their own definition. This may sound like a circular argument that could go on forever, but fear not, we can ensure that our function doesn't become the coding equivalent of a dog chasing its own tail by using a condition to halt the recursion. This is known as a base case.
For a quick introduction on recursion, you can check out this article.
Fibonacci Sequence
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones, usually starting with 0 and 1. The beginning of the sequence looks like this: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, and so on.
Now, let's implement this in JavaScript using recursion.
function fibonacci(n) { if (n <= 1) { return n; } else { return fibonacci(n - 1) + fibonacci(n - 2); } } console.log(fibonacci(10)); // Output: 55
In this code, the base case is when n <= 1
. In these cases, the function simply returns n
. For any larger values of n
, the function calls itself twice – once with the argument n - 1
and once with n - 2
– and adds these two values together. This corresponds exactly to the definition of the Fibonacci sequence!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Recursion Intro (psst, it's free!).
FAQ
Why do we need a base case in a recursive function?
A base case is a condition that halts the recursion. Without a base case, a recursive function would keep calling itself indefinitely, leading to a stack overflow error. In other words, your program would crash because it ran out of memory.
What is the Fibonacci sequence?
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones. It usually starts with 0 and 1.
How is the Fibonacci sequence implemented in the recursive function?
In the recursive function, if the input number n
is less than or equal to 1, the function simply returns n
. For larger values of n
, the function calls itself twice, once with n - 1
and once with n - 2
, and adds these two values together. This mirrors the definition of the Fibonacci sequence, where each number is the sum of the two preceding ones.
Can recursion be used to solve other problems?
Absolutely! Recursion is a powerful concept in programming and can be used to solve a wide range of problems, from sorting and searching algorithms to parsing complex data structures. However, it's important to remember that recursion isn't always the most efficient solution, and in some cases, a non-recursive (iterative) approach might be better.
I tried to generate a really large Fibonacci number and my program crashed. Why?
Recursive functions can be quite memory-intensive, especially when the depth of recursion is high. Each recursive call adds a new layer to the call stack, consuming more memory. If the Fibonacci number you're trying to generate requires a high level of recursion, you may run out of memory, causing the program to crash. This is known as a stack overflow error. In such cases, an iterative solution may be more appropriate.