Fibonacci Sequence Explained
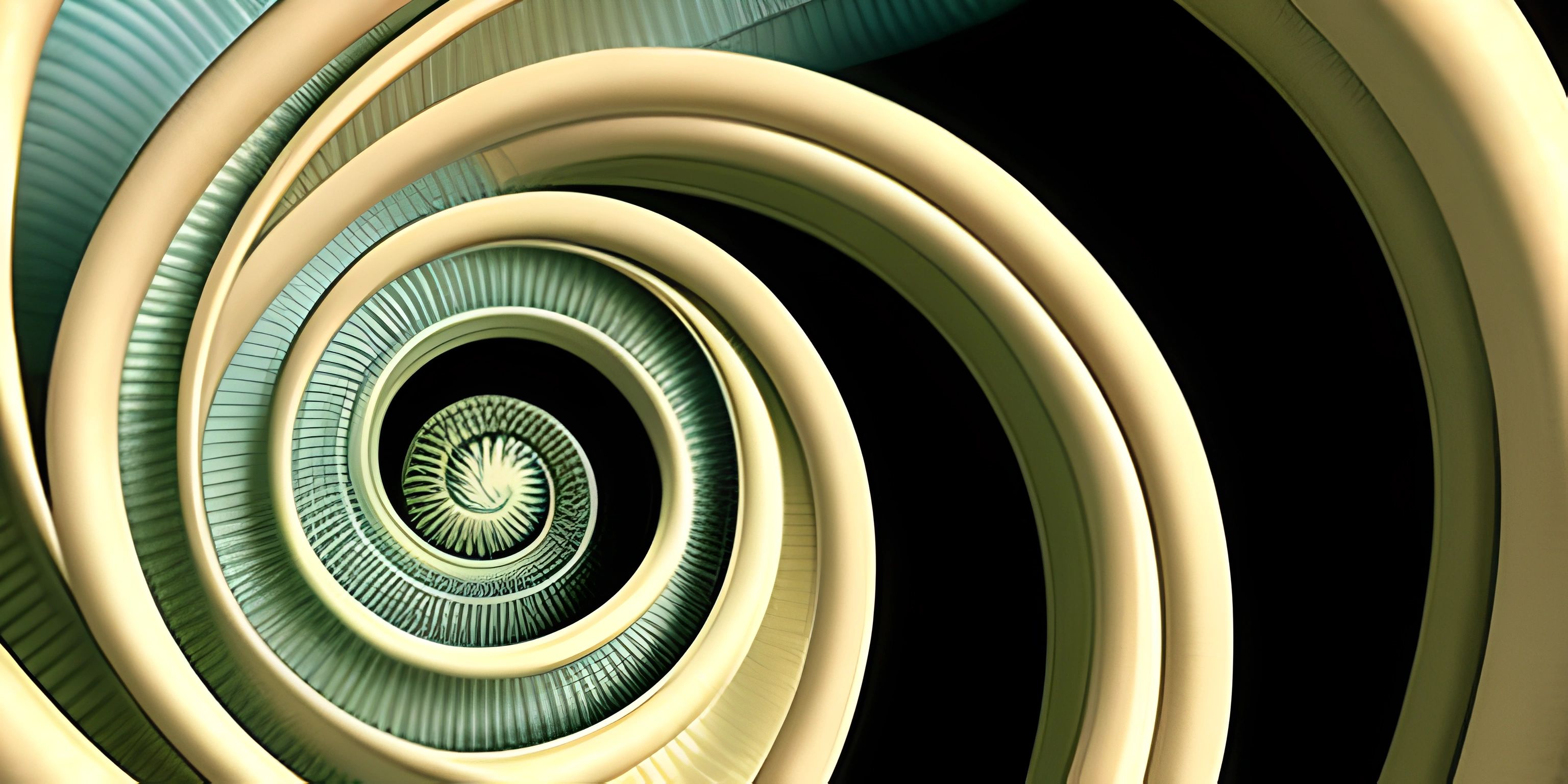
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
One of the most famous sequences in mathematics, and sometimes even in popular culture, is the Fibonacci Sequence. This seemingly simple sequence of numbers has a myriad of fascinating properties and applications in both mathematics and computing. Let's unravel the beauty of the Fibonacci Sequence and find out why it's so important.
What is the Fibonacci Sequence?
The Fibonacci Sequence is an infinite series of numbers, in which each number is the sum of the two preceding ones, starting from 0 and 1. In simpler terms, the sequence starts with 0 and 1, and each subsequent number is obtained by adding the previous two numbers. Here's how the sequence unfolds:
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, ...
As you can see, 0 + 1 = 1, 1 + 1 = 2, 1 + 2 = 3, and so on.
Why is the Fibonacci Sequence Important?
The Fibonacci Sequence has several fascinating properties, and it pops up in various fields, including mathematics, science, and nature. Some of these noteworthy occurrences are:
-
Golden Ratio: The ratio of consecutive Fibonacci numbers converges to the Golden Ratio (approximately 1.618), which is a number that has been revered for its aesthetic beauty in art, architecture, and nature.
-
Spirals in Nature: The Fibonacci Sequence is found in the arrangement of sunflower seeds, pinecone scales, and other natural phenomena. These spirals are efficient ways to pack seeds or scales, allowing the organism to maximize its growth and reproduction.
-
Fractals: The Fibonacci Sequence is used in the construction of some fractals like the Mandelbrot Set.
-
Algorithms and Computing: The Fibonacci Sequence is used in computer science as a basis for various algorithms, including search algorithms, optimization problems, and even as a classic example for recursion and dynamic programming.
How to Generate the Fibonacci Sequence
There are several ways to generate the Fibonacci Sequence in programming, and we'll look at three popular methods below: recursive, iterative, and matrix exponentiation.
Recursive Method
A recursive function is a function that calls itself to solve a problem. The Fibonacci Sequence can be generated using a simple recursive function:
def fibonacci_recursive(n): if n <= 1: return n else: return fibonacci_recursive(n - 1) + fibonacci_recursive(n - 2)
This method works, but it is highly inefficient due to the overlapping and repetitive nature of the calculations. Its time complexity is O(2^n), making it impractical for large values of n.
Iterative Method
An alternative to the recursive method is the iterative method, which uses a loop to calculate the Fibonacci numbers. This method is more efficient, with a time complexity of O(n):
def fibonacci_iterative(n): if n <= 1: return n a, b = 0, 1 for _ in range(n - 1): a, b = b, a + b return b
Matrix Exponentiation
Matrix exponentiation is a more advanced method for generating the Fibonacci Sequence, which takes advantage of linear algebra and has a time complexity of O(log n). The idea is to represent the Fibonacci numbers as the product of a matrix and its powers. This method is outside the scope of this article, but it's worth exploring for those interested in advanced algorithms.
In conclusion, the Fibonacci Sequence is a beautiful blend of mathematics and nature, with a multitude of fascinating properties and applications. Its elegance and simplicity make it a great example for teaching programming concepts such as recursion and dynamic programming. So the next time you come across a spiral in nature, remember the humble Fibonacci Sequence and its amazing connection to our world.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Recursion Intro (psst, it's free!).
FAQ
What is the Fibonacci Sequence?
The Fibonacci Sequence is a series of numbers in which each number is the sum of the two preceding ones, usually starting with 0 and 1. It is named after Leonardo Fibonacci, an Italian mathematician who introduced the sequence to Western mathematics. The sequence goes like this: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, and so on.
How is the Fibonacci Sequence used in mathematics and computing?
The Fibonacci Sequence has various applications in mathematics, computing, and even in nature. In mathematics, it is used to study patterns, sequences, and recursion. In computing, it is often used in algorithms, data structures, and problem-solving techniques. For instance, it can be found in the dynamic programming approach to optimize problems by breaking them down into smaller subproblems. It also appears in nature, with examples like the arrangement of leaves on a plant stem and the spiral pattern of sunflower seeds.
How can I generate the Fibonacci Sequence using a programming language?
Here's an example of generating the Fibonacci Sequence using Python:
def fibonacci(n): if n == 0: return 0 elif n == 1: return 1 else: return fibonacci(n-1) + fibonacci(n-2) for i in range(10): print(fibonacci(i))
This code defines a recursive function called fibonacci
that takes an integer n
as input and returns the nth number in the Fibonacci Sequence. It then prints the first 10 numbers in the sequence using a for loop.
What is the connection between the Fibonacci Sequence and the Golden Ratio?
The Golden Ratio, often denoted as φ (phi), is a mathematical constant approximately equal to 1.61803398875. It is found by dividing a line into two parts such that the ratio of the whole line to the longer part is equal to the ratio of the longer part to the smaller part. The Fibonacci Sequence is closely related to the Golden Ratio, as the ratio between consecutive Fibonacci numbers tends to the Golden Ratio as the numbers get larger. This relationship can be expressed as follows: lim (n → ∞) (F(n+1) / F(n)) = φ Where F(n) represents the nth Fibonacci number and φ is the Golden Ratio.
Are there any other sequences similar to the Fibonacci Sequence?
Yes, there are many other sequences similar to the Fibonacci Sequence, known as Lucas Sequences. These sequences follow the same recurrence relation as the Fibonacci Sequence, but they have different initial values. The most well-known is the Lucas Sequence, which starts with 2 and 1 instead of 0 and 1: 2, 1, 3, 4, 7, 11, 18, 29, 47, 76, and so on. Other Lucas Sequences can be formed by choosing different starting values, leading to a wide variety of related sequences with interesting properties and applications.