Dijkstra's Algorithm
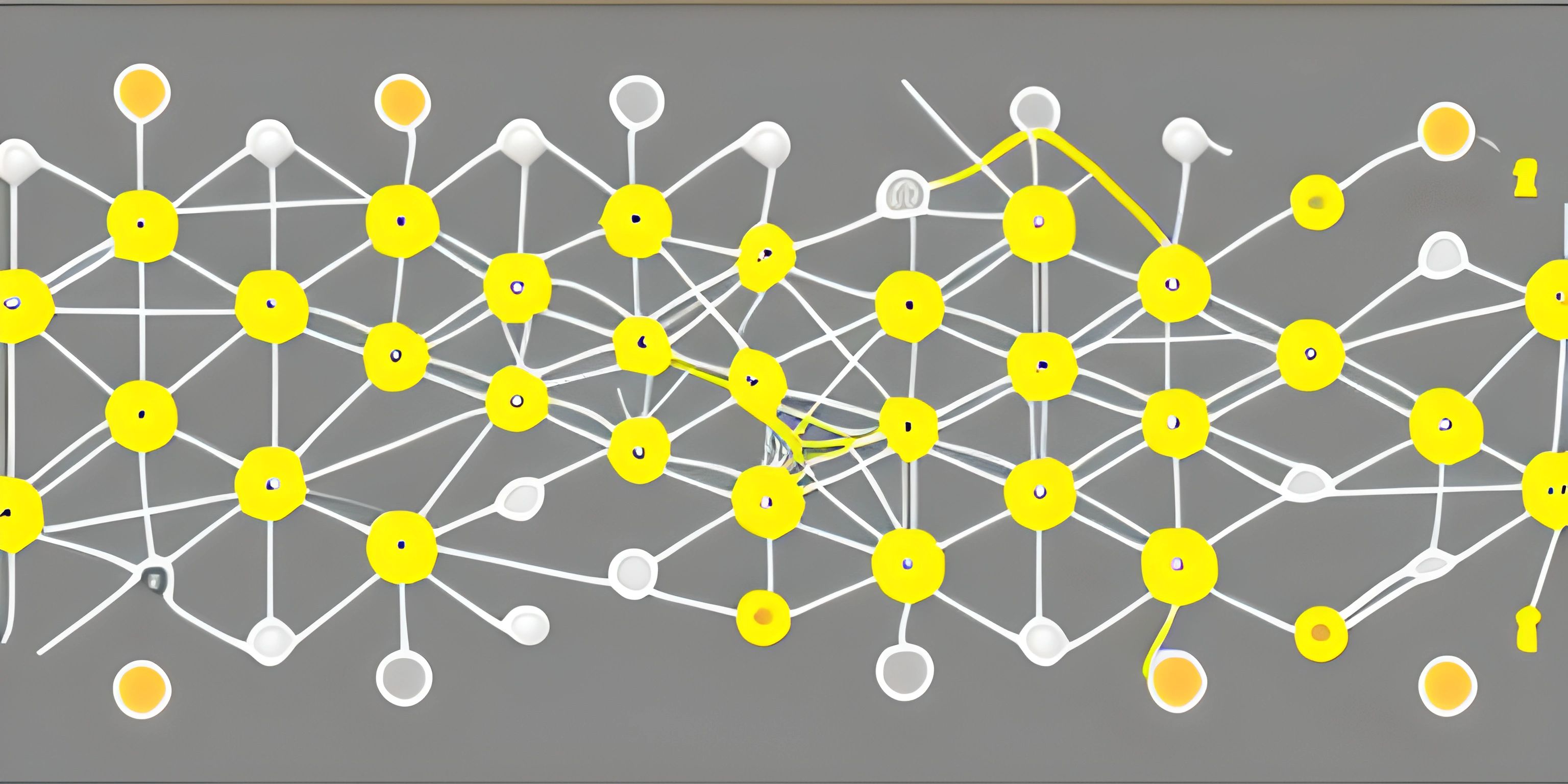
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Dijkstra's algorithm is like the GPS of programming – it helps us navigate through a network of nodes, finding the shortest path along the way. Named after its creator, Edsger Dijkstra, this powerful algorithm is used to solve a variety of problems that involve finding the quickest route or the least cost path in a graph.
Understanding Graphs
Imagine you're playing a game of Pac-Man, and you need to collect all the pellets as quickly as possible. In this scenario, the game board can be represented as a graph, with nodes (pellets) connected by edges (paths). The goal is to find the shortest path that touches each node exactly once. That's where Dijkstra's algorithm comes in handy.
A graph is a collection of nodes (also called vertices) and edges that connect them. Nodes can represent anything from cities in a map to web pages in the internet. Edges, on the other hand, symbolize the connections between these nodes, often with an associated weight or cost (like distance or time).
Dijkstra's Algorithm
Dijkstra's algorithm is a greedy algorithm that finds the shortest path from a starting node to all other nodes in a graph. The algorithm maintains a set of unvisited nodes and a current distance value for each node. Initially, the distance to the starting node is set to 0, while the distances to all other nodes are set to infinity. Dijkstra's algorithm follows these steps:
- Select the node with the smallest distance (initially the starting node) and mark it as visited.
- Update the distances of neighboring nodes by considering the weight of the edge connecting the current node to its neighbors.
- Repeat steps 1 and 2 for all unvisited nodes until all nodes have been visited or the target node has been visited.
Here's a high-level pseudocode representation of Dijkstra's algorithm:
function dijkstra(graph, start): distances = {} visited = set() # Initialize distances for node in graph.nodes: distances[node] = float("inf") distances[start] = 0 # Process the nodes while len(visited) < len(graph.nodes): # Find the node with the smallest distance that has not been visited min_node = None for node in graph.nodes: if node not in visited and (min_node is None or distances[node] < distances[min_node]): min_node = node # Update the distances of neighboring nodes for neighbor, weight in graph.edges[min_node]: new_distance = distances[min_node] + weight if new_distance < distances[neighbor]: distances[neighbor] = new_distance visited.add(min_node) return distances
Applications and Variations
Dijkstra's algorithm can be applied to a wide range of problems, such as network routing, traffic navigation, and even puzzle-solving. Its versatility makes it a popular choice in programming and computer science.
There are also numerous variations and optimizations of Dijkstra's algorithm. For example, the A* algorithm is a popular extension that uses heuristics to guide the search, often resulting in faster solutions. Another optimization involves using priority queues to efficiently find the node with the smallest distance in each iteration.
Now that you have an understanding of Dijkstra's algorithm, you're equipped to tackle a wide array of problems that require finding the shortest path through a graph. It's time to put your newfound knowledge to the test and blaze your trail through the world of programming.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Smiley Face (psst, it's free!).