Bubble Sort In-Depth
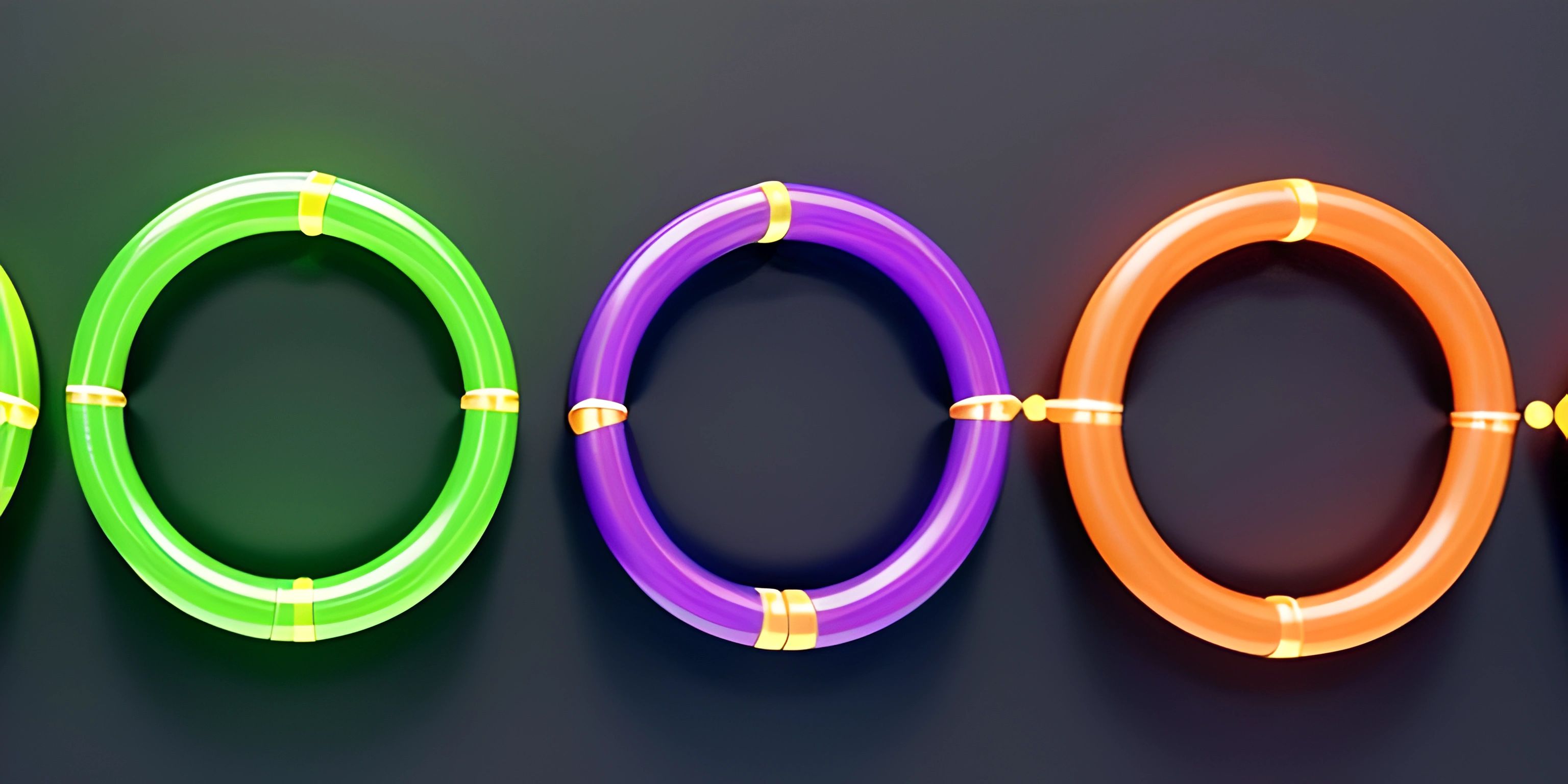
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Sorting is a common problem in computer science, and one of the simplest algorithms to solve it is the Bubble Sort. Bubble Sort may not be the fastest or the most efficient algorithm, but it's a great starting point for understanding the mechanics behind sorting. So let's dive into the world of bubbles and understand how they can help us sort our data!
How Bubble Sort Works
Bubble Sort works by repeatedly iterating through a list of items and comparing adjacent pairs. If the pair is out of order, it swaps them. This process is repeated until no more swaps are needed, which indicates that the list is sorted. Picture a row of bubbles floating on water, and imagine that the bubbles with higher values are heavier. As the heavier bubbles "sink", they swap places with the lighter bubbles, which "float" to the top.
Here's a step-by-step breakdown of the Bubble Sort process:
- Start at the beginning of the list.
- Compare the first pair of adjacent items.
- If the first item is greater than the second item, swap them.
- Move to the next pair and repeat steps 2-3 until the end of the list is reached.
- If any swaps were made during the iteration, go back to the beginning of the list and repeat steps 1-4.
- If no swaps were made during the iteration, the list is sorted, and the algorithm terminates.
Bubble Sort Implementation
The Bubble Sort algorithm can be implemented in most programming languages. Let's take a look at an example implementation in Python:
def bubble_sort(arr): n = len(arr) # Traverse through all elements in the array for i in range(n): # Last i elements are already in place, so no need to check them for j in range(0, n - i - 1): # Swap if the current element is greater than the next one if arr[j] > arr[j + 1]: arr[j], arr[j + 1] = arr[j + 1], arr[j] # Example usage my_list = [64, 34, 25, 12, 22, 11, 90] bubble_sort(my_list) print("Sorted array is:", my_list)
Time Complexity
The time complexity of Bubble Sort is O(n^2) in worst and average cases, where n is the number of items being sorted. This is because for each element in the list, we need to compare and potentially swap it with every other element. In the best case, when the list is already sorted, the time complexity can be reduced to O(n) by adding a flag that checks if any swaps were made in the previous iteration. However, even with this optimization, Bubble Sort is generally not suitable for large datasets.
Use Cases
Bubble Sort is best suited for small datasets or datasets that are already partially sorted. It is also useful for educational purposes, as it is easy to understand and implement. For larger datasets or cases where performance is crucial, other sorting algorithms, such as Quick Sort or Merge Sort, are more appropriate.
In conclusion, Bubble Sort is a simple and intuitive sorting algorithm that provides a solid foundation for understanding the basics of sorting. While it may not be the most efficient algorithm, it's a great starting point for anyone new to the world of computer science and algorithms.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).