Exploring Linked Lists
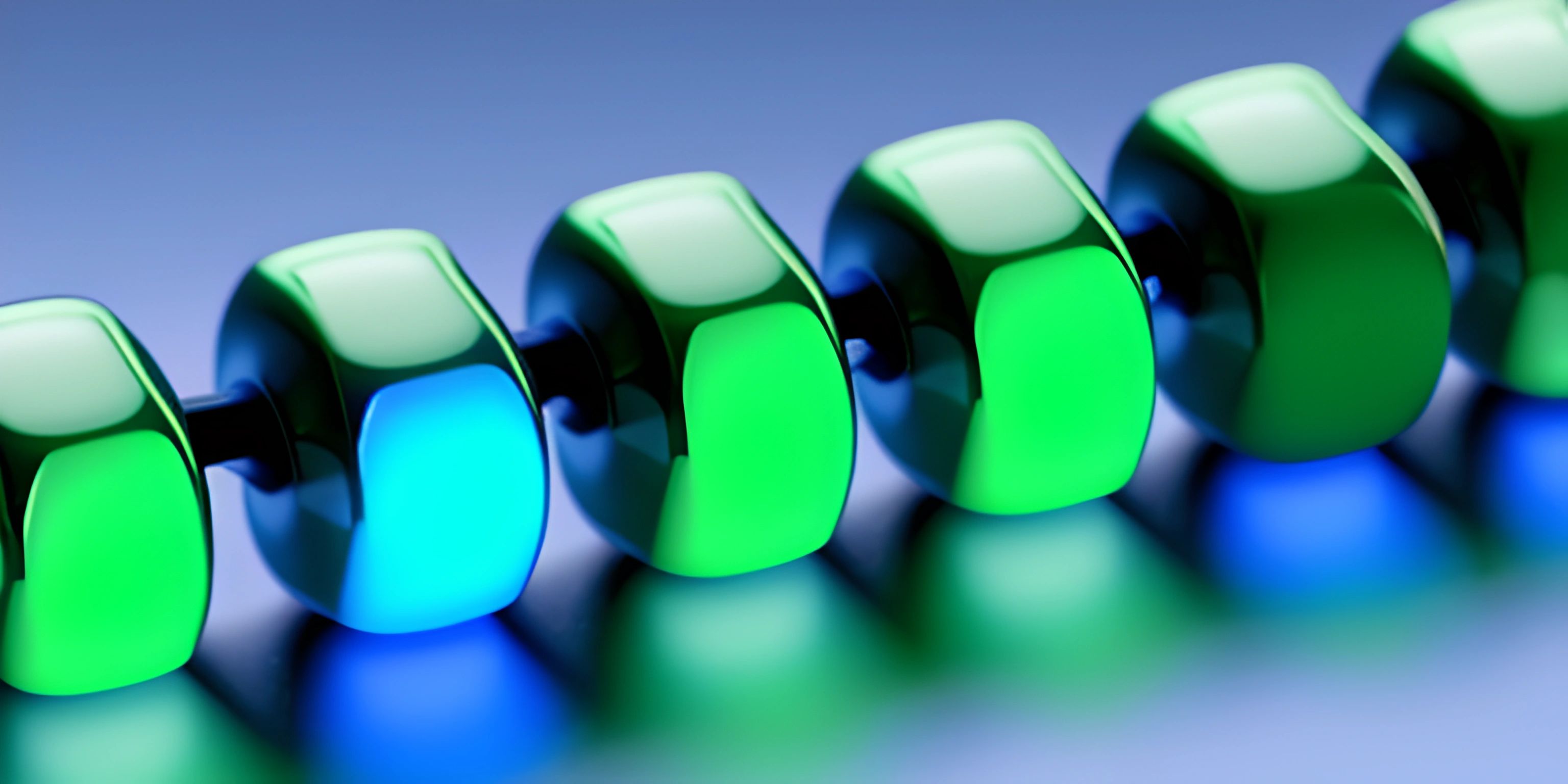
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ah, the humble linked list, a data structure that may not be as fancy as its more popular cousins, like arrays and hash tables, but it's got some tricks up its sleeve that make it an important tool in a programmer's arsenal. Let's dive in and explore this fascinating and useful data structure.
What is a Linked List?
A linked list is a linear data structure in which elements, called nodes, are stored in a sequence. Each node contains two parts: the data and a reference (or a link) to the next node in the sequence. Think of it like a chain of elements, where every element points to its successor. The first node in the list is called the head, and the last node is called the tail.
Here's a visual representation of a simple linked list:
head -> Node1 -> Node2 -> Node3 -> tail
Linked lists come in different flavors, such as singly-linked lists and doubly-linked lists. In a singly-linked list, each node only has a reference to its next neighbor, while in a doubly-linked list, each node has a reference to both its next and previous neighbors.
Advantages of Linked Lists
You might be wondering, "Why would I use a linked list when I have arrays or other fancy data structures?" Good question! Linked lists have some advantages that make them appealing in specific scenarios:
-
Dynamic Size: Unlike arrays, linked lists can grow or shrink in size as elements are added or removed. This makes them more memory-efficient for situations where the exact number of elements is unknown or constantly changing.
-
Ease of Insertion and Deletion: Adding or removing elements in a linked list is a fairly simple operation, as it only requires updating the references in the neighboring nodes. In contrast, inserting or deleting elements in an array often involves shifting multiple elements, which can be time-consuming.
-
No Memory Contiguity: Linked list nodes can be scattered throughout memory, not requiring a contiguous block like arrays. This can help prevent memory fragmentation and make better use of available memory.
Implementing a Linked List
Now that we know what a linked list is and why it's useful, let's see how to implement one. We'll start by defining a simple Node
class:
class Node: def __init__(self, data): self.data = data self.next = None
Next, we'll create a LinkedList
class to wrap our list and provide some useful methods for managing the nodes:
class LinkedList: def __init__(self): self.head = None def add(self, data): new_node = Node(data) new_node.next = self.head self.head = new_node def remove(self, data): # Code to remove a node with the specified data def display(self): # Code to display the data in the list
The add
method in our LinkedList
class creates a new node with the given data and inserts it at the beginning of the list by updating the head reference. You can now add methods to remove nodes and display the list as needed.
Conclusion
Linked lists might not be the flashiest data structure, but they have their place in the world of programming. They offer advantages like dynamic size, ease of insertion and deletion, and memory flexibility. Understanding how to implement and use linked lists can help you write more efficient and adaptable code. So remember, don't underestimate the power of the humble linked list!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Common Programming Pitfalls (psst, it's free!).
FAQ
What is a linked list and what are its advantages?
A linked list is a linear data structure where elements are stored in nodes, and each node points to the next node. Some advantages of linked lists include:
- Dynamic size: Linked lists can easily grow or shrink in size as needed.
- Efficient insertions and deletions: Elements can be inserted or removed from a linked list without the need for reorganizing the entire structure.
- Lower memory overhead: Linked lists don't require contiguous memory allocation, making them more memory-efficient in certain scenarios.
How do I create a simple linked list in Python?
In Python, you can create a simple linked list using classes. Here's an example implementation:
class Node: def __init__(self, data): self.data = data self.next = None class LinkedList: def __init__(self): self.head = None
How do I insert an element into a linked list?
Inserting an element into a linked list can be done in various ways, including at the beginning, end, or a specific position. Here's an example of inserting an element at the beginning of a linked list:
def insert_at_beginning(self, data): new_node = Node(data) new_node.next = self.head self.head = new_node
How do I delete an element from a linked list?
To delete an element from a linked list, you need to find the element, update the pointers, and then remove the element. Here's an example of deleting an element with a given key:
def delete_node(self, key): temp = self.head # If the head node holds the key to be deleted if temp is not None: if temp.data == key: self.head = temp.next temp = None return # Search for the key to be deleted while temp is not None: if temp.data == key: break prev = temp temp = temp.next # If the key is not found, return if temp == None: return # Unlink the node from the linked list prev.next = temp.next temp = None
How do I traverse and print elements in a linked list?
To traverse and print elements in a linked list, use a loop to visit each node until reaching the end of the list. Here's an example:
def print_list(self): temp = self.head while temp: print(temp.data, end=" -> ") temp = temp.next