Data Structures
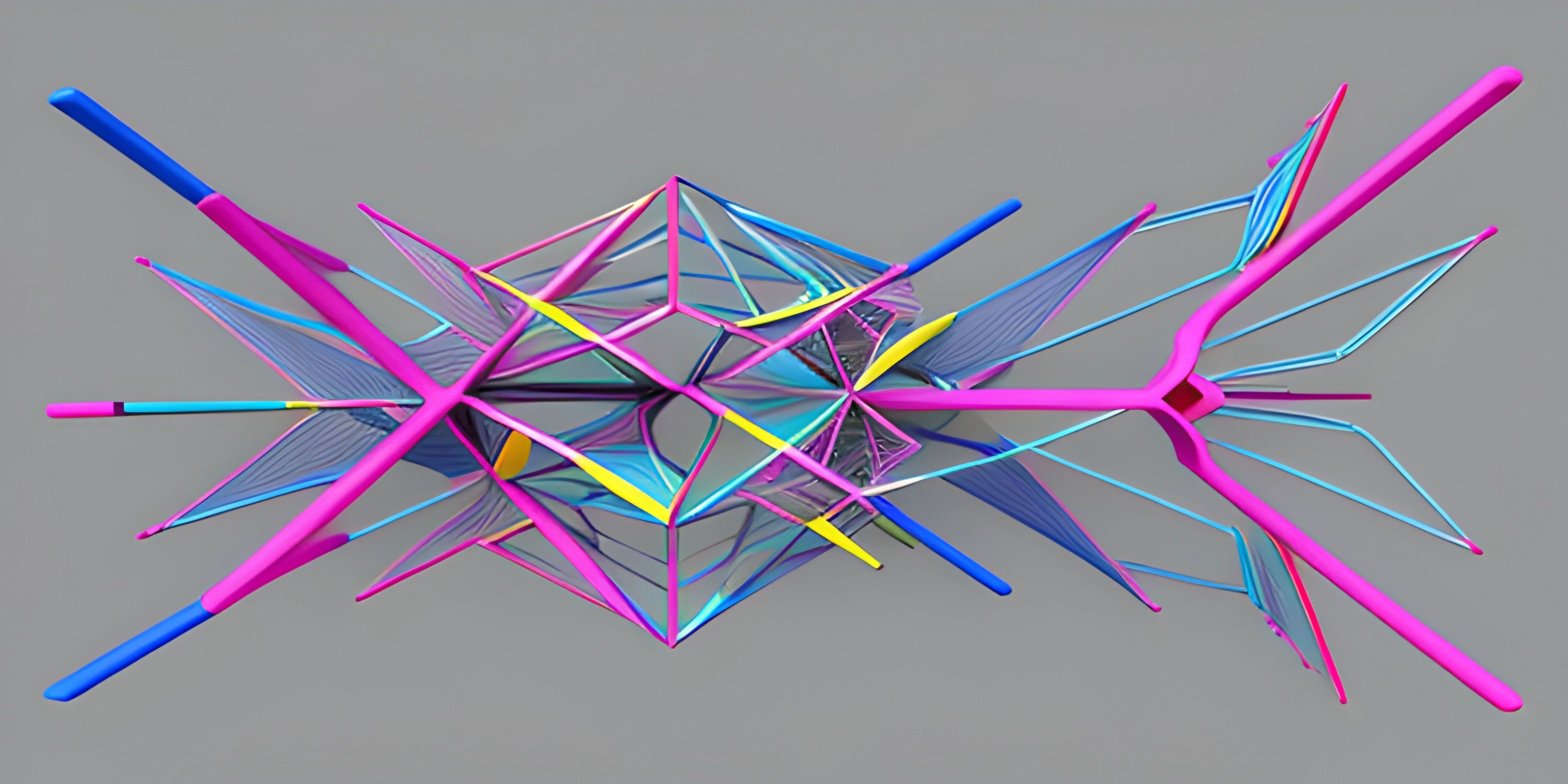
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Data structures are the backbone of programming, as they allow us to store and manipulate information in an organized manner. In this article, we'll discuss some of the most common data structures, like arrays and linked lists, and how they can be used effectively in your code.
Arrays
An array is a data structure that stores a fixed number of elements, all of the same type. Imagine an array like a row of lockers, where each locker has a unique number and can store one item. The size of the array is determined when it's created, and it cannot be changed afterward. Here's an example of creating an array in JavaScript:
let myArray = [1, 2, 3, 4, 5];
Arrays are typically accessed using an index, starting from 0. For example, myArray[0]
would give us the first element (1) and myArray[4]
would give us the last element (5). Keep in mind that trying to access an index outside the range of the array will result in an error or unexpected behavior.
Linked Lists
A linked list is another common data structure that consists of a chain of nodes. Each node contains two parts: the data and a reference to the next node in the chain. Unlike arrays, linked lists are dynamic, meaning they can grow or shrink in size as needed.
Imagine a linked list as a treasure hunt, where each clue (node) leads you to the next one. Here's a simple example of a linked list node in JavaScript:
class Node { constructor(data) { this.data = data; this.next = null; } }
One major advantage of linked lists over arrays is that they can be easily resized, as they don't require a contiguous block of memory. To insert or remove elements, we simply update the references to the next node. However, accessing elements in a linked list can take longer because we need to traverse the list from the beginning until we find the desired element.
Other Data Structures
Arrays and linked lists are just the tip of the iceberg when it comes to data structures. Some other popular data structures include:
- Stacks: A stack is a last-in, first-out (LIFO) data structure that allows you to add and remove elements only from the top.
- Queues: A queue is a first-in, first-out (FIFO) data structure that allows you to add elements to the end and remove elements from the front.
- Trees: A tree is a hierarchy of nodes, where each node has a parent and zero or more children.
- Hash Tables: A hash table is a data structure that allows you to store and retrieve values using a key, providing fast access times.
Understanding and utilizing the right data structure for your specific problem is crucial for efficient programming. As you continue to explore and learn about these structures, you'll become better equipped to tackle complex coding challenges.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Common Programming Pitfalls (psst, it's free!).
FAQ
What are data structures and why are they important in programming?
Data structures are specialized formats for organizing, storing, and manipulating data in a program. They are important because they enable efficient and effective handling of data, allowing developers to write better-performing and more maintainable code.
Can you provide examples of common data structures?
Sure! Some common data structures include:
- Arrays: A contiguous block of memory that stores a fixed-size sequence of elements of the same type.
- Linked Lists: A collection of elements (nodes) with each node containing a reference to the next node in the sequence.
- Stacks: A last-in, first-out (LIFO) data structure where elements are added and removed from one end called the "top."
- Queues: A first-in, first-out (FIFO) data structure where elements are added at the rear and removed from the front.
- Trees: A hierarchical structure consisting of nodes connected by edges, with a single root node and no cycles.
- Hash Tables: A data structure that stores key-value pairs and uses a hash function to map keys to their respective storage locations.
How do I choose the right data structure for my problem?
To choose the right data structure, consider the following factors:
- The type of data you need to store (e.g., numbers, strings, objects)
- The operations you will perform on the data (e.g., search, insert, delete, update)
- The time and space complexity of these operations for different data structures
- Any specific constraints or requirements of your problem domain It's helpful to understand the strengths and weaknesses of various data structures and their suitability for specific tasks.
How do I create an array in popular programming languages like Python and JavaScript?
Creating an array in Python and JavaScript can be done as follows: In Python:
my_array = [1, 2, 3, 4, 5]
In JavaScript:
let myArray = [1, 2, 3, 4, 5];
Can you briefly explain the difference between arrays and linked lists?
Arrays and linked lists are both linear data structures, but they have some key differences:
- Arrays store elements in contiguous memory locations, while linked lists use nodes with references to the next node in the sequence.
- Arrays have a fixed size, whereas linked lists can grow or shrink dynamically.
- Accessing elements in an array is faster due to its memory layout, while linked lists require traversal from the head node.
- Inserting and deleting elements is generally faster in linked lists, as it only requires updating node references, while arrays may involve shifting elements or reallocating memory.