Merge Sort Explained
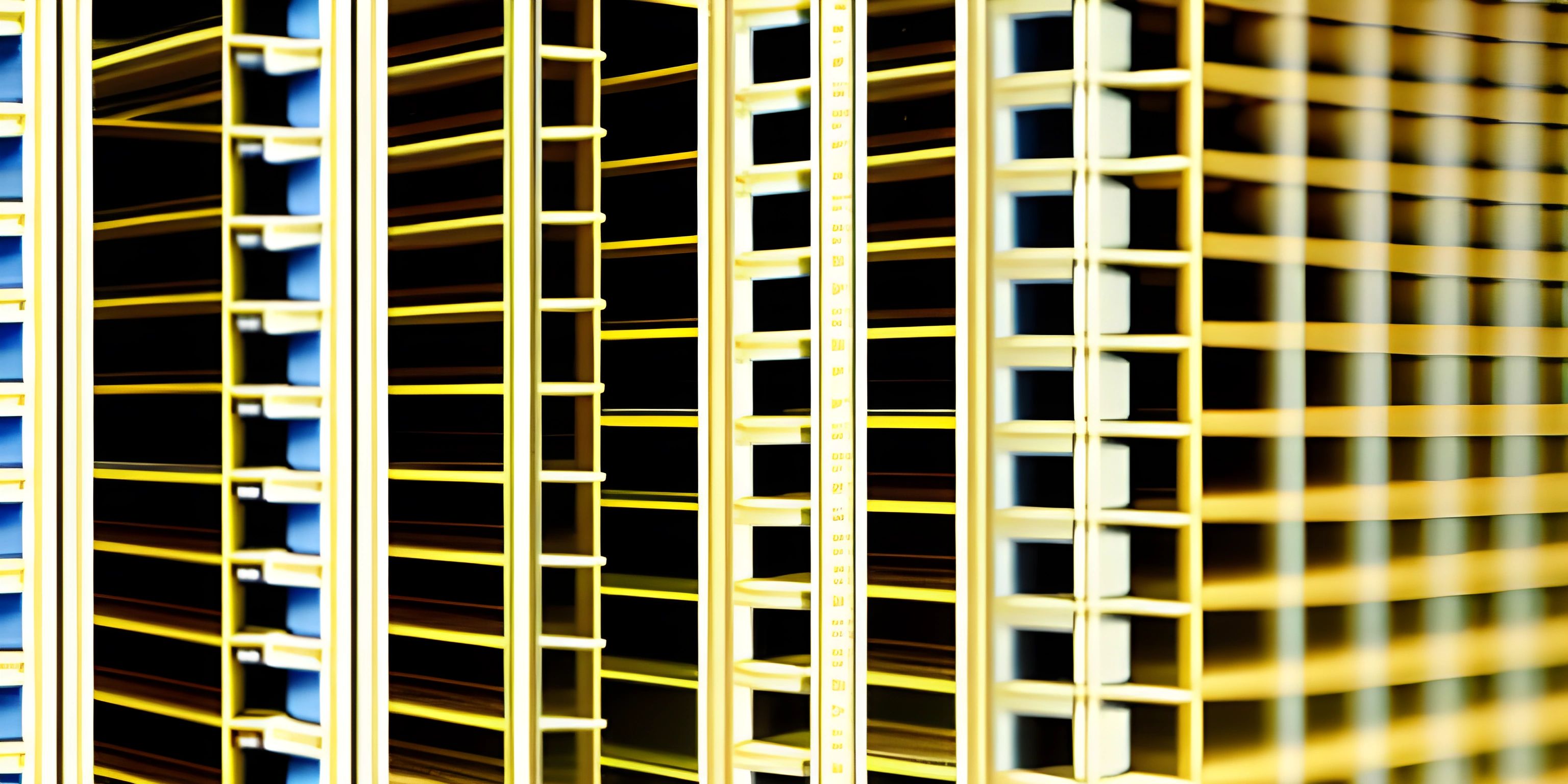
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine you're in charge of sorting a massive pile of books at a library. You could try to sort them all at once, but you'd probably end up overwhelmed and surrounded by a hurricane of novels, biographies, and dusty encyclopedias. Instead, what if you broke the task into smaller, more manageable parts? That's the idea behind merge sort, a powerful algorithm that helps us sort data efficiently by dividing and conquering.
What is Merge Sort?
Merge sort is a divide-and-conquer algorithm that works by splitting an array into smaller sub-arrays, sorting those sub-arrays, and then merging them back together. This method ensures efficiency, even with large datasets. It's a staple algorithm in computer science and is often used in various applications that require sorting large amounts of data.
To understand this better, let's break it down:
- Divide: Split the array into two halves.
- Conquer: Recursively sort each half.
- Combine: Merge the sorted halves to produce the final sorted array.
Why Use Merge Sort?
Merge sort is preferred for several reasons:
- Efficiency: With a time complexity of O(n log n), merge sort is faster than simple quadratic algorithms like bubble sort or insertion sort, especially for larger datasets.
- Stability: Merge sort maintains the relative order of equal elements, which can be important for certain applications.
- Predictability: Its time complexity is consistent, making it reliable for performance-critical applications.
Merge Sort Implementation in Python
Let's dive into the code and see how merge sort can be implemented in Python. Here's a simple and clear implementation:
def merge_sort(arr): # Base case: If the array is of length 1 or less, it's already sorted if len(arr) <= 1: return arr # Split the array into two halves mid = len(arr) // 2 left_half = arr[:mid] right_half = arr[mid:] # Recursively sort each half left_sorted = merge_sort(left_half) right_sorted = merge_sort(right_half) # Merge the sorted halves return merge(left_sorted, right_sorted) def merge(left, right): sorted_array = [] i = j = 0 # Merge the two sorted arrays while i < len(left) and j < len(right): if left[i] < right[j]: sorted_array.append(left[i]) i += 1 else: sorted_array.append(right[j]) j += 1 # Collect the remaining elements sorted_array.extend(left[i:]) sorted_array.extend(right[j:]) return sorted_array # Example usage arr = [38, 27, 43, 3, 9, 82, 10] sorted_arr = merge_sort(arr) print("Sorted array:", sorted_arr)
Breaking Down the Code
- Base Case:
- If the array has one or zero elements, it is already sorted.
- Dividing the Array:
- The array is split into two halves using Python's slicing.
- Recursively Sorting:
- We recursively call
merge_sort
on each half.
- We recursively call
- Merging:
- The
merge
function takes two sorted arrays and combines them into one sorted array. It compares elements of both arrays and appends the smaller element to thesorted_array
.
- The
Use Cases for Merge Sort
Merge sort is especially useful in the following scenarios:
- Large Datasets: It handles large datasets efficiently due to its O(n log n) time complexity.
- External Sorting: When dealing with large amounts of data that don’t fit into memory, merge sort's ability to work with chunks of data makes it a great choice.
- Stable Sorting: When maintaining the relative order of equal elements is important, merge sort's stability is advantageous.
Comparison with Other Sorting Algorithms
While merge sort is efficient and reliable, it's important to consider other algorithms depending on the context:
- Quick Sort: Generally faster for average cases but has a worst-case time complexity of O(n^2).
- Heap Sort: Offers O(n log n) time complexity but is not stable.
- Bubble Sort: Simple but inefficient with O(n^2) time complexity, making it impractical for large datasets.
Each sorting algorithm has its strengths and weaknesses, and the choice of which to use can depend on the specific requirements of the task at hand.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
What is the time complexity of merge sort?
The time complexity of merge sort is O(n log n), making it more efficient than quadratic algorithms like bubble sort or insertion sort for large datasets.
Is merge sort a stable sorting algorithm?
Yes, merge sort is a stable sorting algorithm, meaning it preserves the relative order of equal elements.
Can merge sort be used for external sorting?
Yes, merge sort is suitable for external sorting, where data is too large to fit into memory and needs to be sorted in chunks.
How does merge sort compare to quick sort?
Merge sort has a consistent O(n log n) time complexity while quick sort is generally faster for average cases but has a worst-case time complexity of O(n^2). Quick sort is also not stable, whereas merge sort is.
Why is merge sort preferred for large datasets?
Merge sort is preferred for large datasets because of its efficient O(n log n) time complexity and its ability to handle data that doesn't fit entirely into memory.