Merge Sort Overview
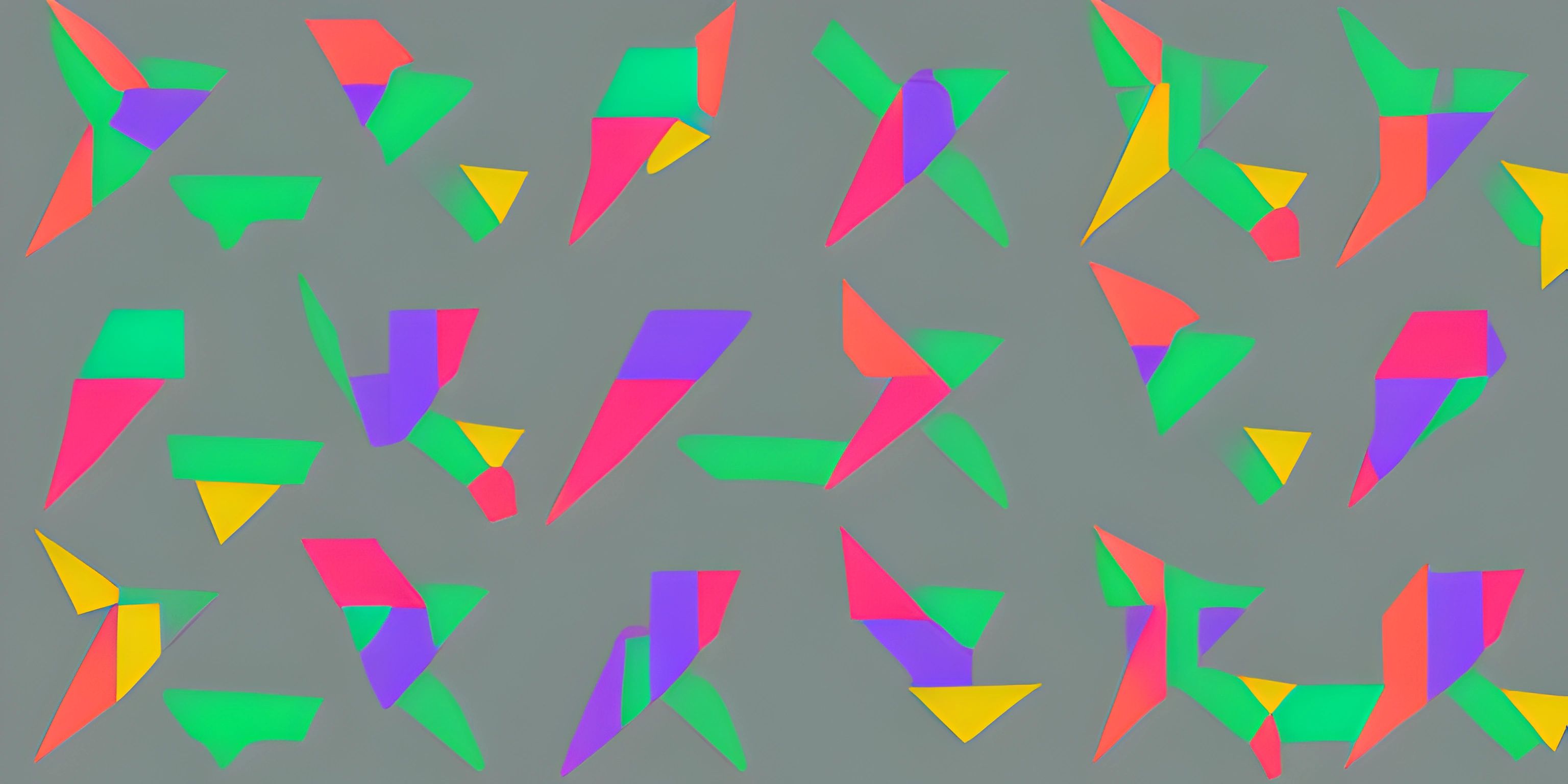
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Sorting is a crucial problem in computer science, and there are many algorithms designed to do it. One of the most efficient and widely used algorithms is the merge sort. It's a divide-and-conquer sorting algorithm that works by recursively dividing the input into smaller parts, sorting them, and then merging the sorted parts to produce the final sorted output.
How Merge Sort Works
Merge sort works on the principle of dividing the input array into smaller sub-arrays, sorting those sub-arrays, and then merging them back together to create a sorted array. The key step in merge sort is the merging of sorted sub-arrays.
Here's a high-level overview of how merge sort works, using a simple array of numbers as an example:
- Split the input array into two equal halves.
- Recursively apply merge sort on both halves.
- Merge the two sorted halves back together.
Merging Sorted Arrays
The process of merging two sorted arrays involves comparing the first elements of both arrays and selecting the smaller one, then moving to the next element in the selected array and repeating the process until one of the arrays is completely traversed. Finally, any remaining elements in the other array are simply appended to the result.
Here's an example of merging two sorted arrays [2, 4, 6]
and [1, 3, 5]
:
Result: [] [2, 4, 6] and [1, 3, 5] => Compare 2 and 1, select 1 Result: [1] [2, 4, 6] and [3, 5] => Compare 2 and 3, select 2 Result: [1, 2] [4, 6] and [3, 5] => Compare 4 and 3, select 3 Result: [1, 2, 3] [4, 6] and [5] => Compare 4 and 5, select 4 Result: [1, 2, 3, 4] [6] and [5] => Compare 6 and 5, select 5 Result: [1, 2, 3, 4, 5] [6] and [] => Append remaining elements from [6] Result: [1, 2, 3, 4, 5, 6]
Implementing Merge Sort
Merge sort can be implemented in various programming languages. For example, here's a simple implementation in Python:
def merge_sort(arr): if len(arr) <= 1: return arr mid = len(arr) // 2 left = merge_sort(arr[:mid]) right = merge_sort(arr[mid:]) return merge(left, right) def merge(left, right): result = [] i = j = 0 while i < len(left) and j < len(right): if left[i] < right[j]: result.append(left[i]) i += 1 else: result.append(right[j]) j += 1 result.extend(left[i:]) result.extend(right[j:]) return result
Performance of Merge Sort
Merge sort has a time complexity of O(n*log(n))
, where n
is the number of elements in the input array. This makes it efficient for sorting large data sets. However, it has a space complexity of O(n)
as it requires additional memory for the merging process.
Compared to other sorting algorithms like bubble sort and insertion sort, merge sort is faster and more efficient, especially for larger data sets. However, it might not be the best choice for smaller data sets or situations where memory usage is a concern.
In summary, merge sort is a powerful and efficient sorting algorithm that works well for most use cases. Understanding its implementation and performance characteristics can help you make informed decisions about which sorting algorithm to use in your projects.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).