Mastering Merge Sort in Python: A Step-by-Step Guide
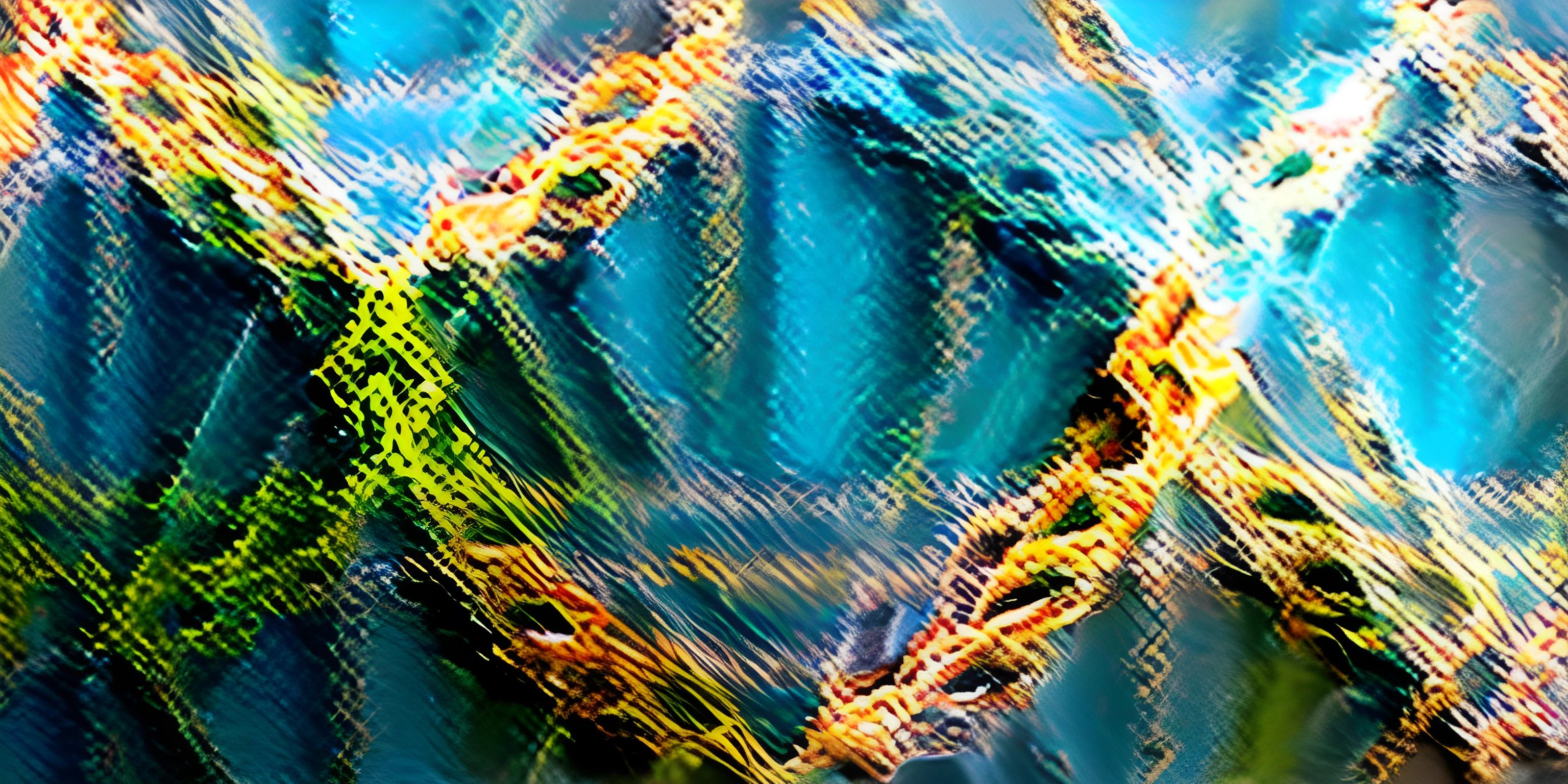
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Sorting algorithms are like the unsung heroes of the coding world. They quietly arrange our data, making it easier to search, analyze, and visualize. One such hero is merge sort. This algorithm is known for its efficiency and simplicity, and today, we're going to dive into its inner workings and learn how to implement it in Python.
What is Merge Sort?
Merge sort is a divide and conquer algorithm. It works by recursively splitting the list into smaller sublists until each sublist contains a single element. Then, it merges these sublists in a sorted manner to produce the final sorted list. Think of it as splitting a deck of cards into individual cards and then meticulously putting them back together in the right order.
The Steps of Merge Sort
Merge sort can be broken down into three main steps:
- Divide: Split the list into two halves.
- Conquer: Recursively sort the two halves.
- Merge: Merge the two sorted halves to produce the sorted list.
Let's take a closer look at each of these steps.
Step 1: Divide
The first step is to split the list into two halves. This is done until each sublist contains only one element. In Python, we can use slicing to achieve this.
def merge_sort(arr): if len(arr) > 1: mid = len(arr) // 2 # Find the middle point left_half = arr[:mid] # Split the array into two halves right_half = arr[mid:] # Recursively sort each half merge_sort(left_half) merge_sort(right_half)
Step 2: Conquer
In this step, we recursively sort each half. Since a single-element list is already sorted, the recursion will eventually bottom out, and we can start merging.
Step 3: Merge
The final step is to merge the two sorted halves. This is done by comparing the elements of each half and arranging them in order.
i = j = k = 0 # Initialize pointers for left_half, right_half, and arr # Copy data to temp arrays left_half[] and right_half[] while i < len(left_half) and j < len(right_half): if left_half[i] < right_half[j]: arr[k] = left_half[i] i += 1 else: arr[k] = right_half[j] j += 1 k += 1 # Checking if any element was left while i < len(left_half): arr[k] = left_half[i] i += 1 k += 1 while j < len(right_half): arr[k] = right_half[j] j += 1 k += 1
Putting It All Together
Now, let's put everything together into a complete function.
def merge_sort(arr): if len(arr) > 1: mid = len(arr) // 2 left_half = arr[:mid] right_half = arr[mid:] merge_sort(left_half) merge_sort(right_half) i = j = k = 0 while i < len(left_half) and j < len(right_half): if left_half[i] < right_half[j]: arr[k] = left_half[i] i += 1 else: arr[k] = right_half[j] j += 1 k += 1 while i < len(left_half): arr[k] = left_half[i] i += 1 k += 1 while j < len(right_half): arr[k] = right_half[j] j += 1 k += 1
And there you have it! You've successfully implemented merge sort in Python. To see it in action, let's create a list and sort it using our function.
arr = [12, 11, 13, 5, 6, 7] print("Given array:", arr) merge_sort(arr) print("Sorted array:", arr)
Running this code will sort the array [12, 11, 13, 5, 6, 7]
into [5, 6, 7, 11, 12, 13]
.
Time Complexity
Merge sort has a time complexity of O(n log n), making it a very efficient sorting algorithm, especially for large datasets. This efficiency comes from its divide and conquer approach, which splits the list log(n) times and performs n comparisons during the merging process.
Advantages and Disadvantages
Advantages
- Stable: Merge sort preserves the order of equal elements, which is important in certain applications.
- Predictable O(n log n) Time Complexity: Unlike some other sorting algorithms, merge sort consistently performs well, regardless of the input data.
Disadvantages
- Space Complexity: Merge sort requires additional space proportional to the size of the input list, which can be a drawback for large datasets.
- Not In-Place: The algorithm creates additional lists during the sorting process, which can lead to higher memory usage.
Conclusion
Merge sort is a powerful and efficient sorting algorithm that is worth adding to your programming toolkit. Its divide and conquer approach makes it both elegant and effective. Understanding and implementing merge sort will not only improve your sorting skills but also give you a deeper appreciation for algorithmic thinking.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What is merge sort?
Merge sort is a divide and conquer sorting algorithm that recursively splits a list into smaller sublists, sorts them, and then merges them back together in a sorted order.
How does merge sort work?
Merge sort works by dividing the list into two halves, recursively sorting each half, and then merging the sorted halves back together.
What is the time complexity of merge sort?
The time complexity of merge sort is O(n log n), making it very efficient for large datasets.
What are the advantages of merge sort?
Merge sort is stable, has a predictable O(n log n) time complexity, and works well with large datasets.
What are the disadvantages of merge sort?
Merge sort requires additional space proportional to the size of the input list and is not an in-place sorting algorithm, leading to higher memory usage.