Introduction to NUnit
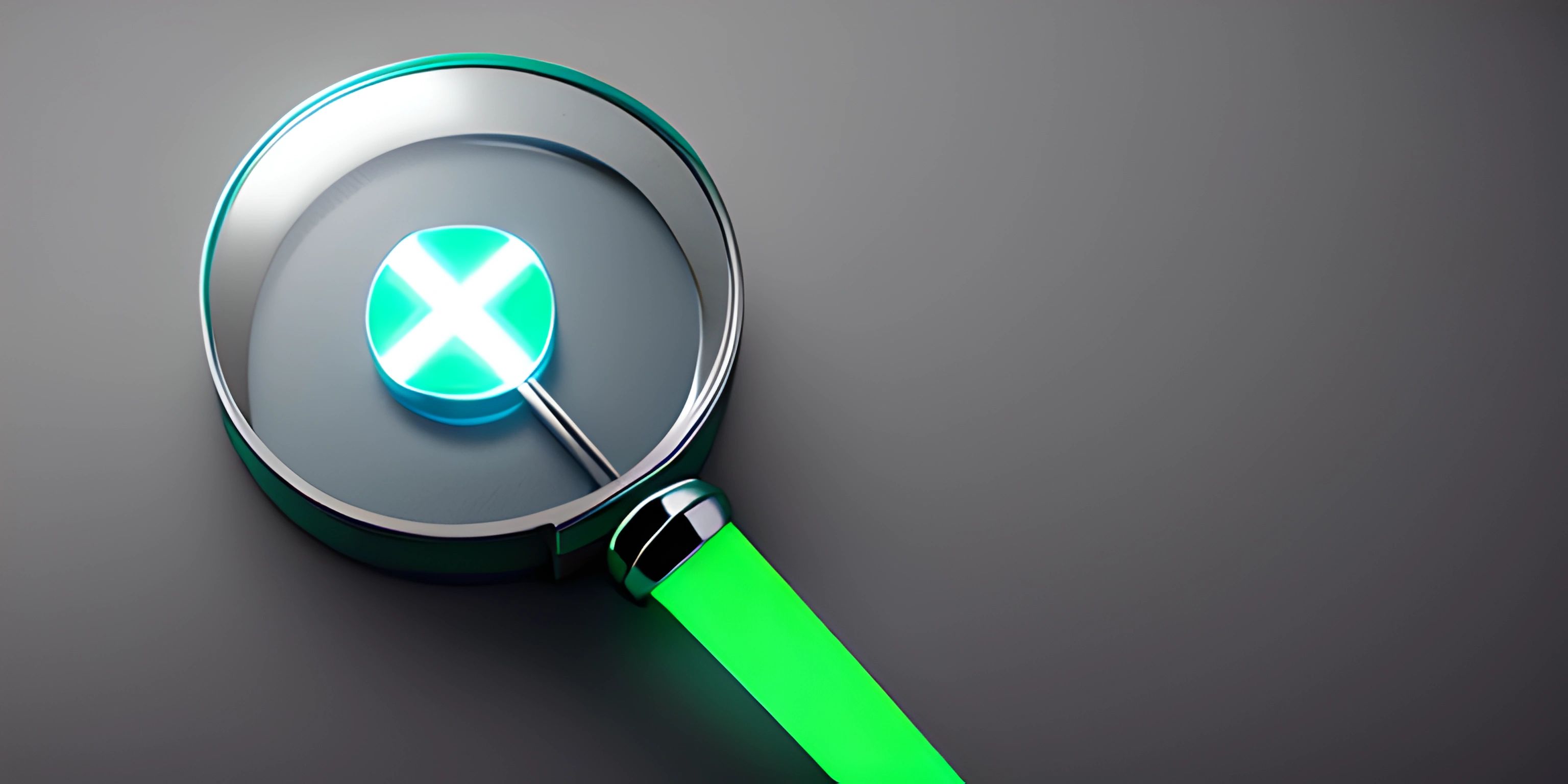
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
NUnit is a popular open-source testing framework for .NET applications, designed to help developers write and run unit tests effectively. Using NUnit, you can write tests for your code to ensure it behaves as expected, catch potential bugs early, and improve the overall quality of your software.
Why NUnit?
There are several testing frameworks available for .NET applications, but NUnit has become a favorite among developers for several reasons:
- It's open-source and widely used, with a strong community contributing to its development and improvement.
- NUnit is easy to learn and use, with intuitive syntax and powerful features.
- It's compatible with a wide range of .NET platforms, including .NET Core, .NET Framework, and Xamarin.
- NUnit integrates seamlessly with popular IDEs like Visual Studio, making it easy to write, run, and debug tests.
Setting Up NUnit
To get started with NUnit, you'll need to install the NUnit NuGet package in your project. Open your project in Visual Studio and follow these steps:
- Right-click on your project in the Solution Explorer and select "Manage NuGet Packages."
- In the NuGet Package Manager, search for "NUnit" and install the latest version of the package.
- Next, search for "NUnit3TestAdapter" and install the latest version of the package. This adapter allows Visual Studio to discover and run your NUnit tests.
Writing Your First Test
With NUnit installed, you're ready to write your first unit test. Create a new class in your project and add the following using statements:
using NUnit.Framework;
Now, you can write a simple test method. In NUnit, test methods are marked with the [Test]
attribute. Here's an example test that checks if the sum of two numbers is correct:
[Test] public void TestAddition() { int a = 5; int b = 10; int actual = a + b; int expected = 15; Assert.AreEqual(expected, actual, "The addition test failed."); }
In this test, we set up some variables, perform an addition operation, and then use the Assert
class provided by NUnit to check if the result is as expected.
Running Your Tests
To run your tests in Visual Studio, open the Test Explorer window by going to "Test > Windows > Test Explorer." Your NUnit tests should appear in the list. Simply click the "Run All" button to execute your tests.
If all goes well, your tests will pass, and you'll see a green checkmark next to each one. If a test fails, you can click on it to see the details of the failure, including any error messages and stack traces.
Advanced NUnit Features
NUnit offers many advanced features to help you write more efficient and flexible tests, such as:
[SetUp]
and[TearDown]
attributes for running code before and after each test.[TestFixture]
attribute for organizing tests into groups and sharing common setup/teardown code.[TestCase]
attribute for running a single test with multiple sets of input data.[Ignore]
attribute for temporarily disabling a test.[Category]
attribute for tagging and filtering tests based on specific criteria.
Exploring these features will help you write more effective unit tests and make the most of NUnit's capabilities.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: AI Test Playground (psst, it's free!).
FAQ
What is NUnit?
NUnit is an open-source testing framework for .NET applications, designed to help developers write and run unit tests effectively. It helps ensure code behaves as expected, catch potential bugs early, and improve overall software quality.
How do I set up NUnit in my project?
To set up NUnit, install the NUnit NuGet package and the NUnit3TestAdapter NuGet package in your project using Visual Studio's NuGet Package Manager. This will enable you to write, discover, and run NUnit tests in your .NET application.
How do I write a basic NUnit test?
To write a basic NUnit test, create a new class in your project, and import the NUnit.Framework namespace. Then, write a test method marked with the [Test] attribute. Inside the test method, use the Assert class provided by NUnit to check if the results of your code meet the expected outcomes.
How do I run NUnit tests in Visual Studio?
To run NUnit tests in Visual Studio, open the Test Explorer window (Test > Windows > Test Explorer). Your NUnit tests should appear in the list. Click the "Run All" button to execute your tests and see the results.
What are some advanced features of NUnit?
Advanced features of NUnit include SetUp and TearDown attributes, TestFixture attribute, TestCase attribute, Ignore attribute, and Category attribute. These features help you write more efficient and flexible tests, organize tests into groups, share common setup/teardown code, run tests with multiple sets of input data, temporarily disable tests, and tag/filter tests based on specific criteria.