Dependency Injection
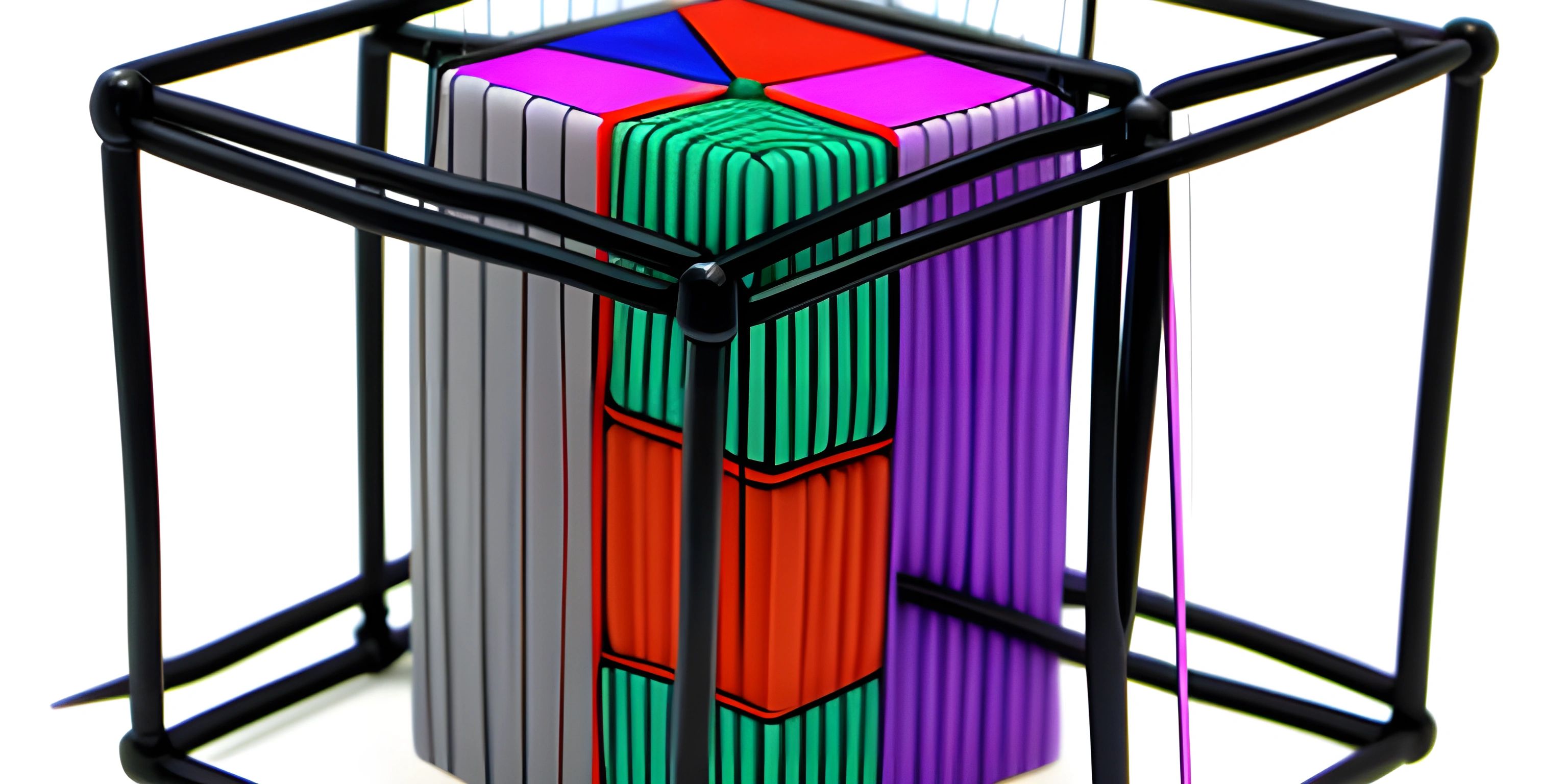
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Dependency injection is a design pattern that helps us create flexible, testable, and maintainable code. It's like giving your code a set of Lego bricks that it can use to build and modify itself, instead of having to rely on a fixed, pre-built structure. In a nutshell, dependency injection is all about providing an object with the resources it needs, instead of forcing it to create or find them itself.
Why Dependency Injection?
Imagine you're building a robot to help you with your chores. You could hardwire all the robot's components, like its arms, legs, and power supply, directly into its brain. Now, what if you want to upgrade your robot with longer arms or a more powerful battery? You'd have to rip it apart and rewire everything.
But what if, instead of hardwiring everything, you designed your robot with detachable, swappable components? This would make upgrading and maintenance a breeze! That's the beauty of dependency injection – it helps you build more modular, flexible, and future-proof code.
How Does It Work?
In dependency injection, we separate an object's creation and usage. We "inject" dependencies (i.e., the resources an object needs to function) from the outside. This can be done in several ways:
- Constructor injection: Pass the dependencies as arguments to the object's constructor.
- Setter injection: Use setter methods to provide the dependencies after the object has been constructed.
- Interface injection: Implement an interface that specifies methods to receive the dependencies.
Let's see an example in Python:
class Engine: def start(self): print("Engine started") class Car: def __init__(self, engine): # Constructor injection self.engine = engine def drive(self): self.engine.start() print("Car is moving") engine = Engine() car = Car(engine) car.drive()
Here, the Car
class has a dependency on the Engine
class. Instead of creating an Engine
object inside the Car
class, we pass it as an argument. This way, we can easily replace the Engine
with another implementation without changing the Car
class.
Benefits of Dependency Injection
- Flexibility: Easier to swap out or upgrade components.
- Testability: Simplifies unit testing by allowing you to inject mock dependencies.
- Reusability: Promotes code reuse and separation of concerns, leading to cleaner and more maintainable code.
Implementing Dependency Injection in Different Languages
Dependency injection can be implemented in most programming languages. In some languages, like Java and C#, you can use dedicated dependency injection frameworks (Spring for Java and Unity for C#). In other languages, you can implement dependency injection manually, as shown in the Python example above.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Advanced Data Types (psst, it's free!).
FAQ
What is dependency injection?
Dependency injection is a design pattern that provides objects with the resources they need to function, instead of forcing them to create or find these resources themselves. This creates more flexible, testable, and maintainable code.
What are the benefits of dependency injection?
Dependency injection offers several benefits, including greater flexibility, easier testability, and improved code reusability. By decoupling object creation and usage, dependency injection promotes code reuse and separation of concerns, making it easier to maintain and upgrade your code.
Can dependency injection be used in any programming language?
Yes, dependency injection can be implemented in most programming languages. Some languages, like Java and C#, have dedicated dependency injection frameworks, while others, like Python, can implement dependency injection manually.