Next.js API Routes
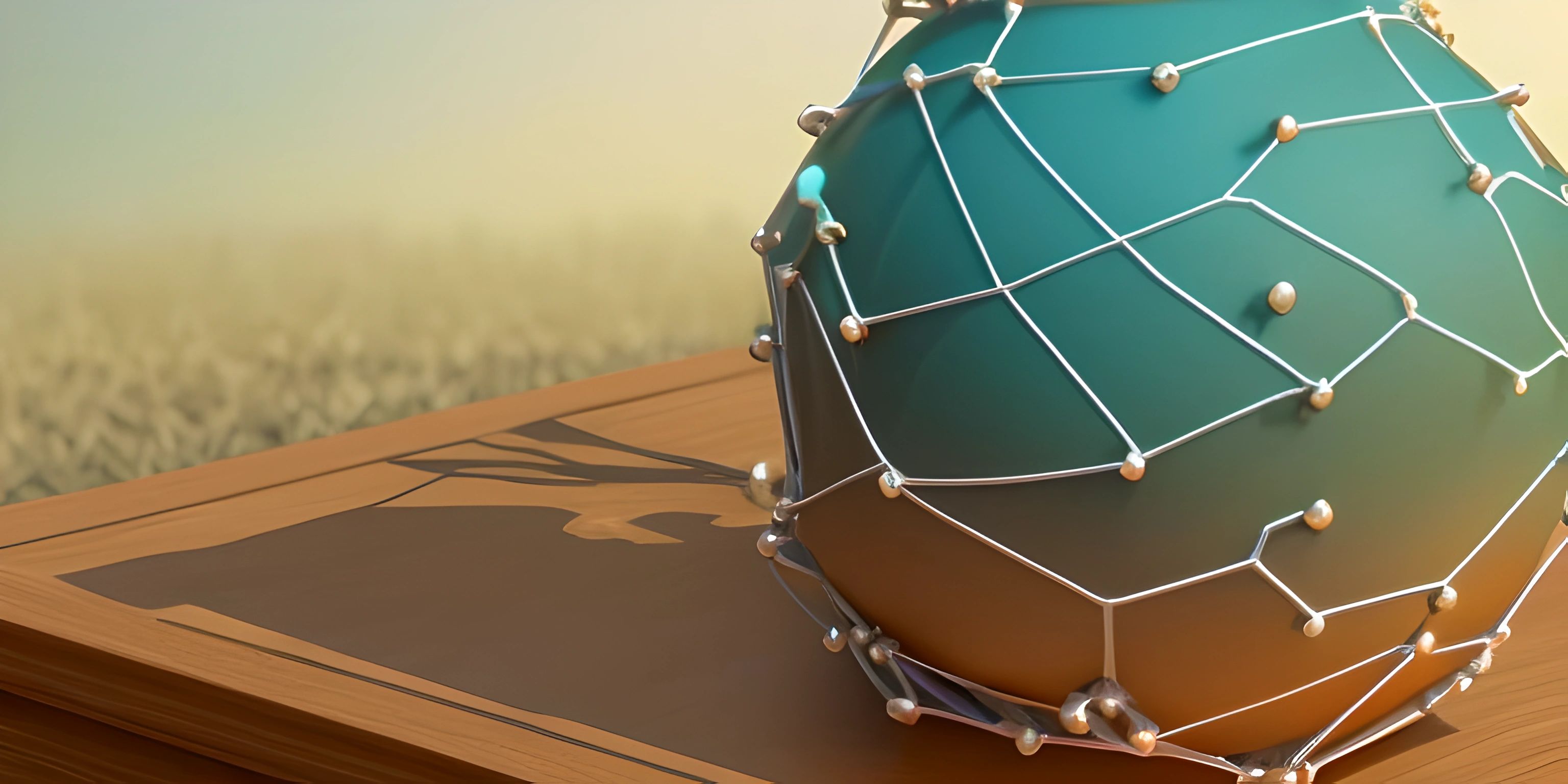
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Next.js is a powerful React framework that simplifies server-side rendering and API creation. One of the standout features in Next.js is its API routes. They allow you to create server-side API endpoints without the need for a separate server or API setup. In this adventure, we'll explore how to create and use API routes in a Next.js application.
API Routes in a Nutshell
API routes in Next.js are functions that run on the server-side and return data to the client. They are perfect for handling tasks like authentication, data fetching, and form submissions. API routes are contained within the pages/api
directory of your Next.js project, and each file represents an API endpoint.
Creating an API Route
To create an API route, simply add a new file with a .js
or .ts
extension inside the pages/api
directory. The file name will be used as the API route path. For example, if you create a file named hello.js
, the API route will be accessible at http://localhost:3000/api/hello
.
Inside this file, we'll write a function that handles incoming HTTP requests. Let's create a basic hello
API route:
// pages/api/hello.js export default function handler(req, res) { res.status(200).json({ message: "Hello from the API route!" }); }
In this example, we've created a default exported function named handler
. It takes two arguments: req
(request) and res
(response). The req
object holds information about the incoming request, while the res
object is used to send a response back to the client.
We send a 200 status code (OK) and a JSON object containing a greeting message as our response.
Fetching Data from an API Route
Now that we have our API route set up, let's fetch data from it using the built-in fetch()
function in a Next.js component:
// pages/index.js import { useEffect, useState } from "react"; export default function Home() { const [greeting, setGreeting] = useState(""); useEffect(() => { async function fetchData() { const response = await fetch("/api/hello"); const data = await response.json(); setGreeting(data.message); } fetchData(); }, []); return ( <div> <h1>{greeting}</h1> </div> ); }
In this example, we use the useState
and useEffect
hooks from React to fetch and display the greeting message from our /api/hello
endpoint. The fetch()
function returns a Promise that resolves to the Response object representing the response to the request. We then parse the response as JSON and update our component state with the fetched data.
And with that, you've successfully created and consumed an API route in your Next.js application! As your project grows, you can create more API routes to handle various server-side tasks and keep your application's functionality neatly organized.
Remember, the possibilities with Next.js API routes are vast. You can integrate them with databases, external APIs, or even use them for server-side rendering. So go forth and conquer the world of server-side functionality in your Next.js app!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).