Understanding Object-Relational Mapping and Its Benefits
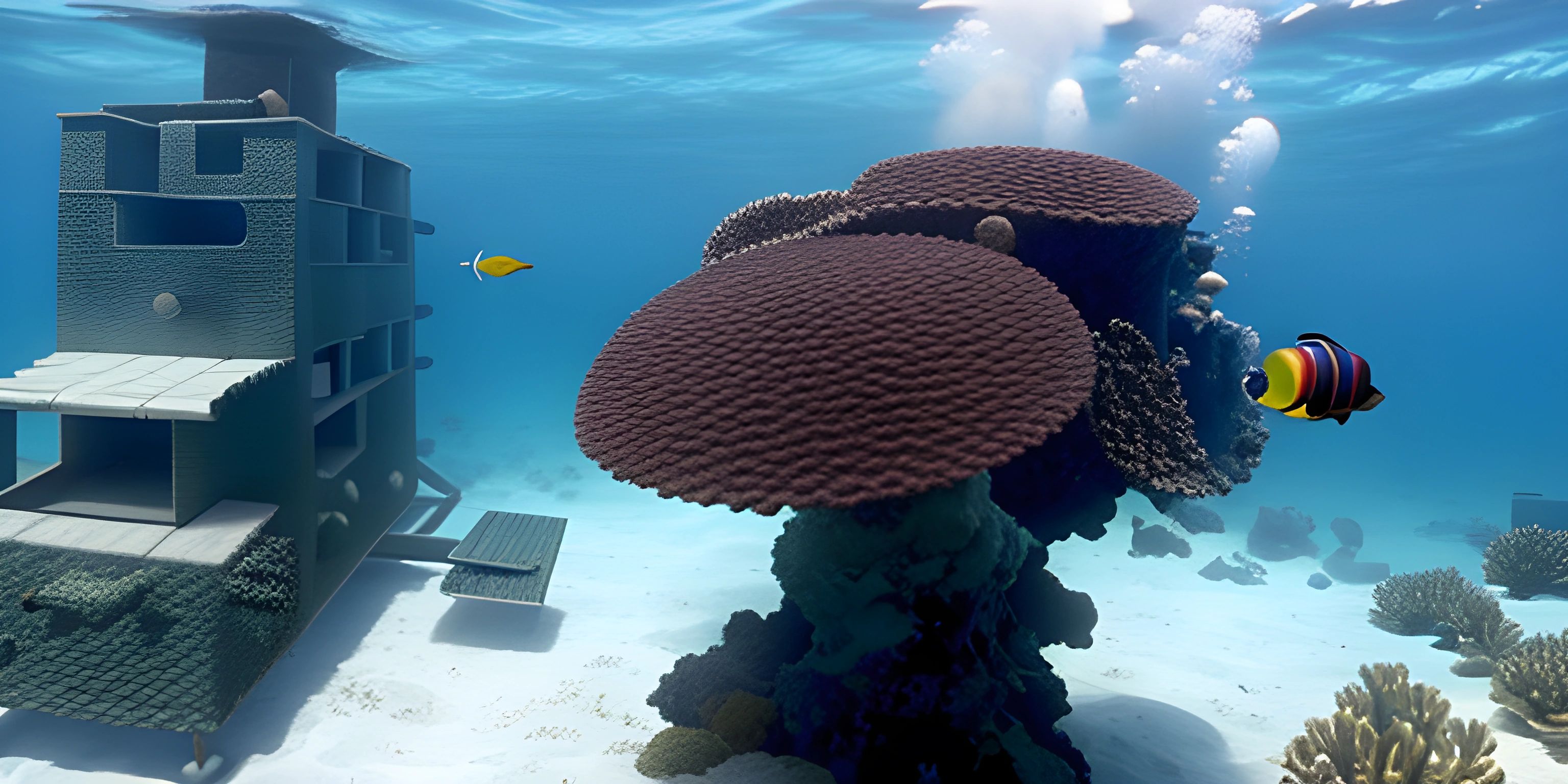
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ever felt like your code and your database are speaking different languages? Like trying to communicate with your dog through interpretive dance? Well, that's where Object-Relational Mapping, or ORM, comes to the rescue. ORM is like a translator that helps your code and your database work together smoothly.
What is ORM?
Object-Relational Mapping (ORM) is a technique that allows you to interact with a relational database using an object-oriented paradigm. Essentially, it lets you work with database records as if they were regular objects in your programming language.
Imagine you have a database table called "users" with columns like "id", "name", and "email". Without ORM, you'd write SQL queries to fetch and manipulate this data. With ORM, you can use objects and methods instead. Here's a quick example in Python using the SQLAlchemy library:
from sqlalchemy import create_engine, Column, Integer, String from sqlalchemy.ext.declarative import declarative_base from sqlalchemy.orm import sessionmaker # Create a database engine engine = create_engine("sqlite:///example.db") # Define a base class for our ORM models Base = declarative_base() # Define a User class mapped to the "users" table class User(Base): __tablename__ = "users" id = Column(Integer, primary_key=True) name = Column(String) email = Column(String) # Create the "users" table in the database Base.metadata.create_all(engine) # Create a session to interact with the database Session = sessionmaker(bind=engine) session = Session() # Create a new user object new_user = User(name="John Doe", email="[email protected]") # Add the new user to the session and commit the transaction session.add(new_user) session.commit()
In this example, the User
class represents a row in the "users" table. You can create, read, update, and delete users using Python objects and methods instead of raw SQL queries.
Benefits of Using ORM
Simplified Code
ORM simplifies your code by abstracting away the details of SQL queries. You can focus on your application's logic rather than worrying about constructing and executing SQL statements. This makes your code cleaner and easier to read.
Database Agnosticism
One of the biggest advantages of using ORM is that it makes your code database-agnostic. This means you can switch from one database system to another (e.g., from SQLite to PostgreSQL) without having to rewrite large portions of your code. The ORM handles the differences between database systems for you.
Increased Productivity
By using ORM, you can speed up your development process. ORM libraries often come with features like query building, relationship management, and schema migrations out of the box. This means you can get more done with less code.
Reduced Boilerplate Code
ORM reduces the amount of boilerplate code you need to write. For example, instead of writing numerous SQL statements for CRUD (Create, Read, Update, Delete) operations, you can use simple method calls.
Enhanced Security
ORM frameworks often include built-in protection against SQL injection attacks. This is because they use parameterized queries under the hood, which helps ensure that user input is properly escaped.
Better Maintainability
Since ORM abstracts database interactions, your codebase can be more modular and easier to maintain. Changes to the database schema can be managed through migrations, and the impact on your application code is minimized.
Common ORM Libraries
SQLAlchemy (Python)
SQLAlchemy is a popular ORM library for Python. It offers a comprehensive set of tools for working with databases, including an ORM, schema migrations, and query building.
Hibernate (Java)
Hibernate is a widely-used ORM framework for Java. It provides a powerful set of features for mapping Java objects to database tables and managing relationships between them.
ActiveRecord (Ruby)
ActiveRecord is the ORM layer included with Ruby on Rails. It offers a simple and intuitive API for working with databases and supports a wide range of database systems.
Entity Framework (C#)
Entity Framework is the ORM library for the .NET framework. It provides a rich set of tools for working with databases in C# and supports both code-first and database-first approaches.
When Not to Use ORM
While ORM offers many benefits, it's not always the best choice for every project. Here are some scenarios where you might want to avoid using ORM:
- Complex Queries: If your application requires complex SQL queries or advanced database features, ORM might not be sufficient. In these cases, writing raw SQL might be more efficient.
- Performance: ORM can introduce performance overhead due to its abstraction layer. For performance-critical applications, direct SQL queries might be more suitable.
- Learning Curve: ORM frameworks come with a learning curve. If your team is not familiar with ORM, it might take some time to get up to speed.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Web Frameworks (React) (psst, it's free!).
FAQ
What is Object-Relational Mapping (ORM)?
Object-Relational Mapping (ORM) is a technique that allows you to interact with a relational database using an object-oriented paradigm. It simplifies database operations by letting you work with database records as if they were regular objects in your programming language.
What are some popular ORM libraries?
Some popular ORM libraries include SQLAlchemy for Python, Hibernate for Java, ActiveRecord for Ruby, and Entity Framework for C#. These libraries provide tools for mapping objects to database tables and managing relationships between them.
What are the benefits of using ORM?
The benefits of using ORM include simplified code, database agnosticism, increased productivity, reduced boilerplate code, enhanced security, and better maintainability. ORM abstracts away the details of SQL queries, making your code cleaner and easier to manage.
When should I avoid using ORM?
You might want to avoid using ORM in scenarios where your application requires complex SQL queries, performance is critical, or your team is not familiar with ORM frameworks. In such cases, writing raw SQL might be more efficient and easier to manage.
How does ORM enhance security?
ORM frameworks often include built-in protection against SQL injection attacks. They use parameterized queries under the hood, which helps ensure that user input is properly escaped and reduces the risk of SQL injection vulnerabilities.