Parsing Algorithms
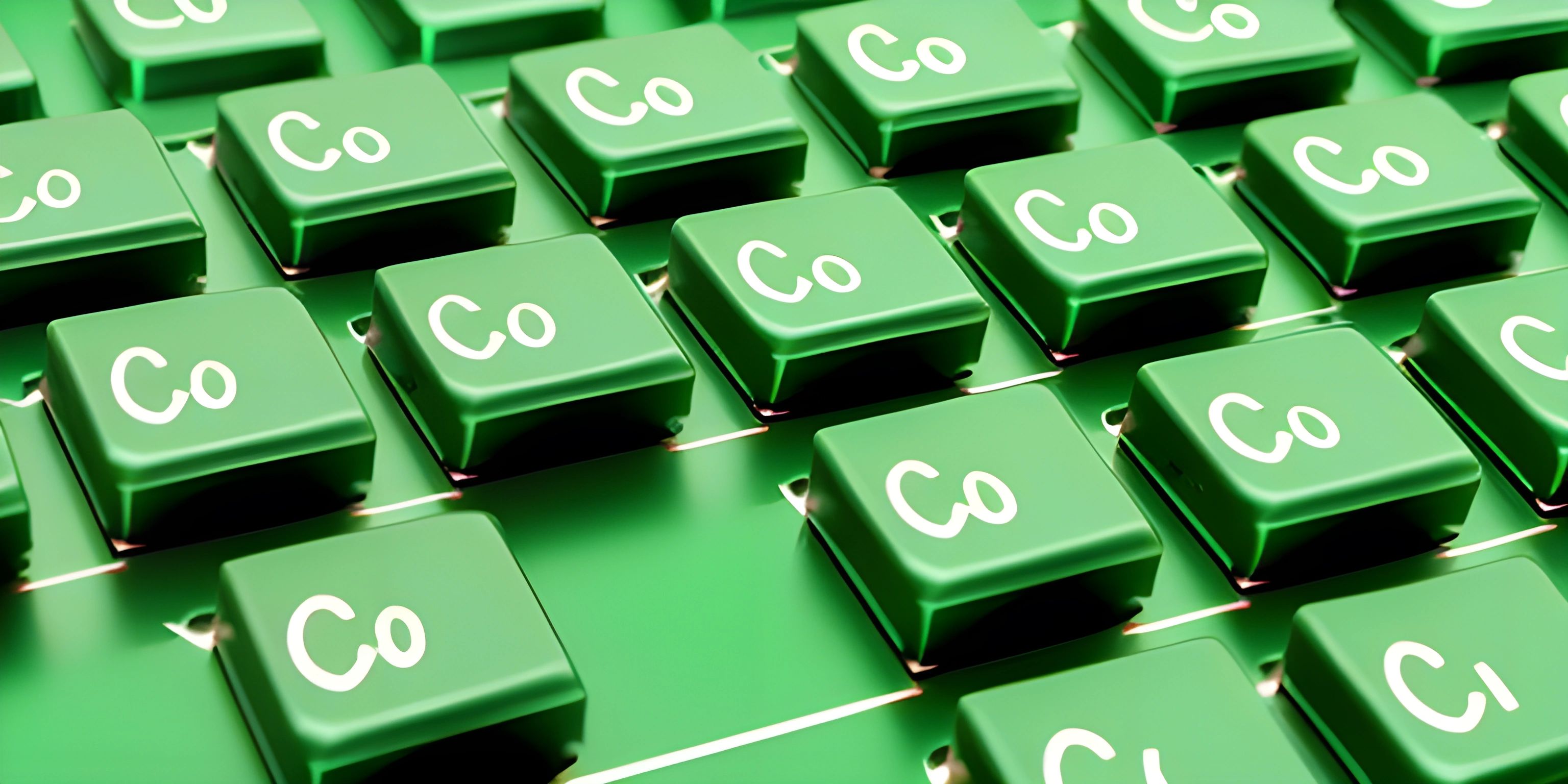
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Parsing is an essential part of any compiler or interpreter. It refers to the process of analyzing a sequence of tokens (usually the output of lexical analysis) to determine their grammatical structure based on a formal grammar. In other words, parsing helps us understand the meaning behind the code we've written. In this article, we'll explore various parsing algorithms and their importance in compilers.
Compiler Overview
A compiler is a program that transforms source code written in a high-level programming language into a lower-level language, usually machine code or assembly language. The compilation process consists of several stages:
- Lexical Analysis
- Syntax Analysis (Parsing)
- Semantic Analysis
- Intermediate Code Generation
- Code Optimization
- Code Generation
In this article, we'll focus on the second stage, Syntax Analysis, where parsing algorithms play a crucial role.
Top-Down Parsing and Bottom-Up Parsing
There are two main approaches to parsing: top-down parsing and bottom-up parsing.
Top-Down Parsing
Top-down parsing starts with the root (start symbol) of the parse tree and then generates the input string by following the production rules of the grammar. It works by predicting what the input should be and then validating the prediction by examining the input.
Recursive Descent Parser is an example of a top-down parsing algorithm. It's a simple yet powerful method, which constructs the parse tree by recursively applying the production rules of the grammar. However, this technique might involve backtracking, making it inefficient for certain grammars.
Bottom-Up Parsing
Bottom-up parsing constructs the parse tree from the input string by reducing the string to the start symbol using the production rules of the grammar. This approach works by recognizing the structure of the input and then constructing the parse tree accordingly.
Shift-Reduce Parser is an example of a bottom-up parsing algorithm. It shifts the input onto a stack and then reduces the input by applying the production rules in reverse order. The process continues until the start symbol is reached. One popular variant of the shift-reduce parser is the LR Parser (Left-to-right, Rightmost derivation), which is widely used due to its efficiency and ability to parse a large class of grammars.
Earley Parser
Earley Parser is a parsing algorithm that can handle both top-down and bottom-up parsing. It's capable of parsing any context-free grammar and is known for its efficiency and flexibility. The algorithm was developed by Jay Earley in 1970 and is still considered one of the most general parsing algorithms in existence.
Selection and Complexity
Choosing the right parsing algorithm depends on the grammar of the programming language being parsed and the desired performance characteristics. Some algorithms are more efficient for certain types of grammars, while others can handle a broader range of grammars but may not be as efficient.
In terms of complexity, parsing algorithms can be classified into polynomial-time and exponential-time algorithms. Polynomial-time algorithms, such as LR Parsers, are more efficient and suitable for practical use. Exponential-time algorithms, like general top-down parsers with backtracking, may not be suitable for large-scale applications due to their performance limitations.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Web Frameworks (React) (psst, it's free!).
FAQ
What is parsing in the context of compilers?
Parsing is the process of analyzing a sequence of tokens, usually the output of lexical analysis, to determine their grammatical structure based on a formal grammar. This helps us understand the meaning behind the code we've written. Parsing is an essential step of the syntax analysis stage in the compilation process.
What are the two main approaches to parsing?
The two main approaches to parsing are top-down parsing and bottom-up parsing. Top-down parsing starts with the root (start symbol) of the parse tree and generates the input string by following the production rules of the grammar. Bottom-up parsing constructs the parse tree from the input string by reducing the string to the start symbol using the production rules of the grammar.
What are examples of top-down and bottom-up parsing algorithms?
Recursive Descent Parser is an example of a top-down parsing algorithm, while Shift-Reduce Parser is an example of a bottom-up parsing algorithm. One popular variant of the shift-reduce parser is the LR Parser (Left-to-right, Rightmost derivation).
What is the Earley Parser?
The Earley Parser is a parsing algorithm that can handle both top-down and bottom-up parsing. It's capable of parsing any context-free grammar and is known for its efficiency and flexibility. It was developed by Jay Earley in 1970 and is still considered one of the most general parsing algorithms in existence.