Syntax Analysis
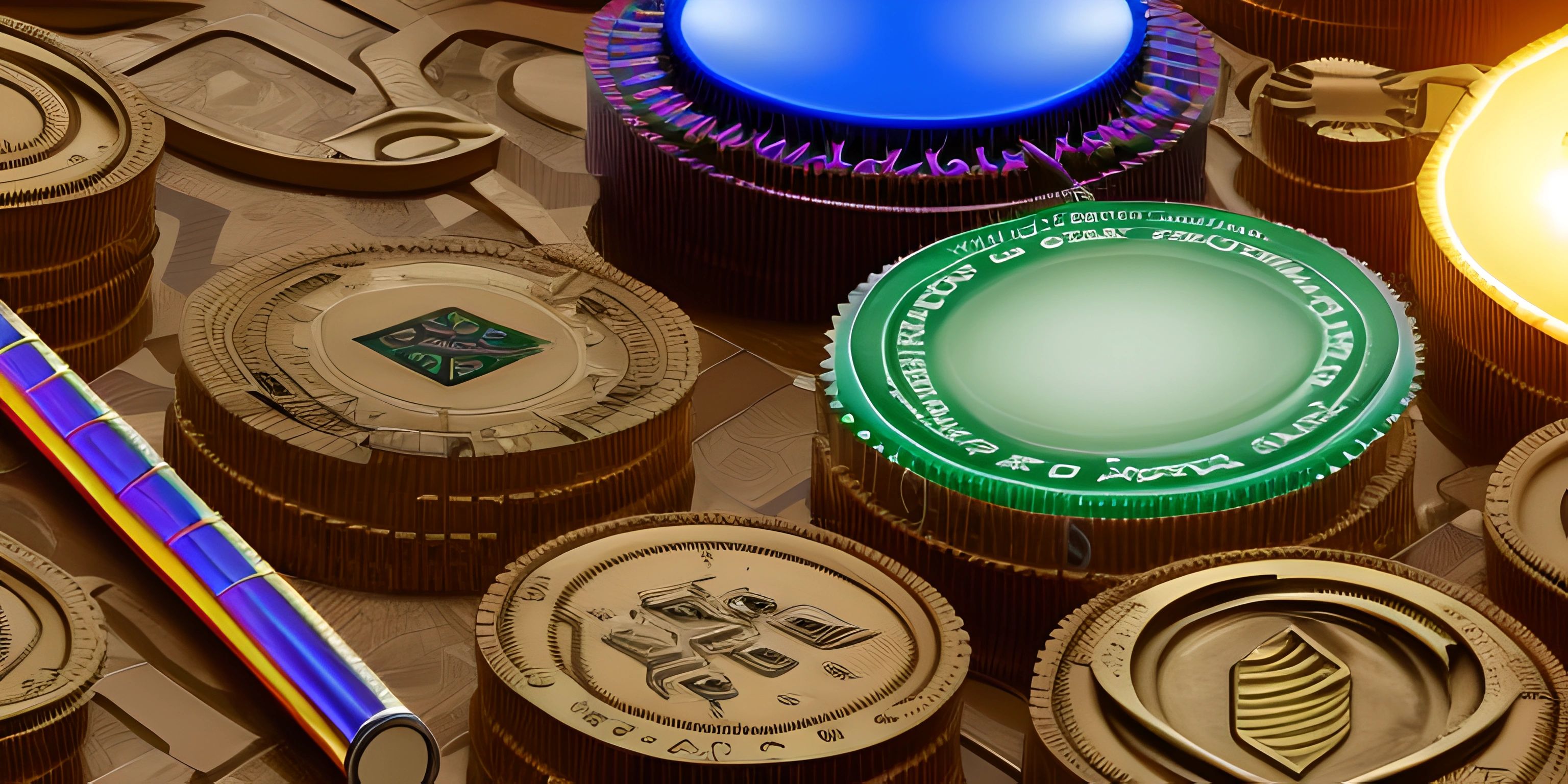
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
We may read code like a piece of literature, but for a computer, it's a completely different story. When writing code, it's essential to adhere to specific rules and structures so the computer can understand what you're trying to say. This is where syntax analysis comes into play.
What is Syntax Analysis?
Syntax analysis, also known as parsing, is the process of analyzing a program's source code to ensure it follows the rules and structure of a programming language. Computers can't understand code written in a casual, human-like way, so syntax analysis helps translate our code into a format that a computer can process.
The syntax analysis is performed by a crucial component of a compiler or interpreter called the parser. The parser takes the source code as input, checks if it's written according to the language's grammar rules, and produces an output called the abstract syntax tree (AST). The AST represents the code's structure, which is later used in other stages of compilation or interpretation.
Lexical Analysis
Before we dive deeper into syntax analysis, it's essential to understand the step that comes right before it: lexical analysis. Lexical analysis is the process of breaking down the source code into smaller units called tokens. Tokens are the basic building blocks, like keywords, identifiers, literals, and operators, that make up a program's source code. The output of this stage is a stream of tokens that the parser uses during syntax analysis.
Parsing Techniques
Now that we have a basic understanding of syntax analysis and its role in compilers and interpreters, let's explore some common parsing techniques.
Top-Down Parsing
In top-down parsing, the parser starts from the highest-level grammar rule and works its way down to the actual source code. Two popular top-down parsing methods are:
-
Recursive Descent Parsing: This approach involves writing recursive procedures for each grammar rule. The parser calls these procedures according to the structure of the code, effectively simulating the derivation of the source code from the grammar rules.
-
Predictive Parsing: This approach relies on the lookahead tokens to decide which production rule to apply without backtracking. The most widely-used predictive parsing method is the LL(1) parsing.
Bottom-Up Parsing
In bottom-up parsing, the parser starts from the source code and tries to derive the highest-level grammar rule. The parser builds the AST by gradually reducing the input tokens to the start symbol of the grammar. A popular bottom-up parsing method is:
- Shift-Reduce Parsing: This approach involves shifting input tokens onto a stack and reducing them to grammar rules when a right-hand side of a production rule is found. The most popular shift-reduce parsing method is the LR(1) parsing.
Error Handling and Recovery
During syntax analysis, parsers can encounter errors in the source code that violate the language's grammar rules. Error handling and recovery is an essential aspect of syntax analysis to help developers identify and fix these issues.
Some common error handling strategies are:
-
Panic Mode: The parser discards input tokens until it finds a token that can synchronize the parsing process, allowing it to continue parsing the remaining code.
-
Phrase-Level Recovery: The parser attempts to replace, insert, or delete tokens to create a syntactically correct phrase.
-
Error Productions: The grammar rules are extended with error-handling productions that allow the parser to recover from specific syntax errors.
Understanding syntax analysis and parsing techniques is crucial for writing code that follows the rules of a programming language. Not only does it help you write better code, but it also provides insight into how compilers and interpreters process your code, ensuring your programs run smoothly and efficiently.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).