Understanding Priority Queues and Their Implementations
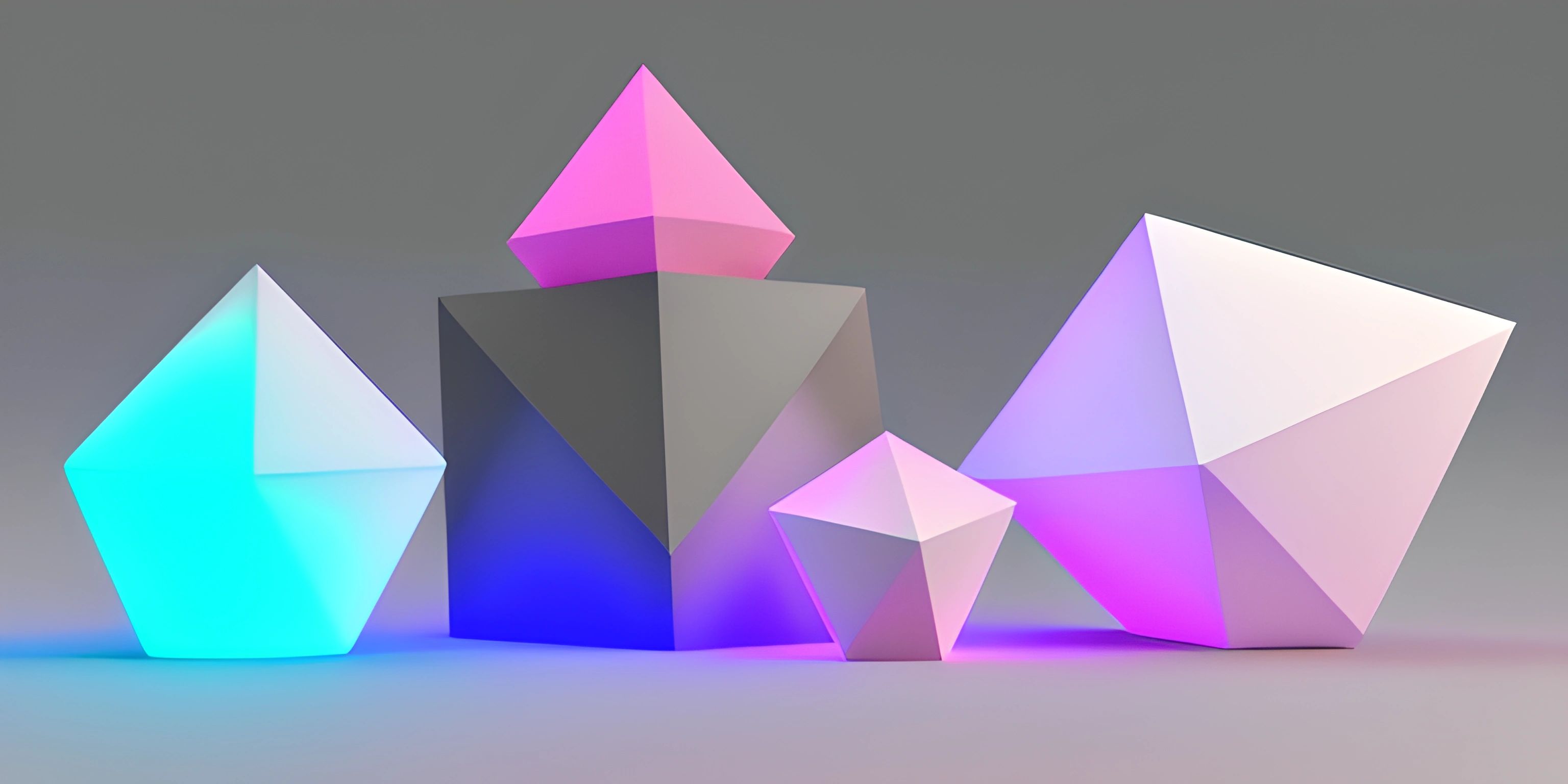
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Priority queues are like the VIP section of data structures. They are a special kind of queue that allows elements to be inserted with a priority level. When you pull an element out of a priority queue, the element with the highest priority is removed first. Gather around, and let's dive into the world of priority queues!
What is a Priority Queue?
A priority queue is a data structure that stores elements along with their priority levels. The priority levels determine the order in which elements are dequeued. Elements with higher priority are removed before elements with lower priority. If two elements have the same priority, they are dequeued based on their order of insertion, making it a first-in-first-out (FIFO) structure for elements with equal priority.
Priority queues are used in various scenarios, such as scheduling processes in an operating system, finding the shortest path in graph algorithms like Dijkstra's algorithm, and managing events in a simulation.
Implementing Priority Queues
There are several ways to implement a priority queue, and each has its own set of trade-offs. Let's take a look at some common implementations.
Array or List
One simple way to implement a priority queue is by using an array or a list. When inserting an element, we can either append it to the end of the list or insert it in the correct position based on its priority. The dequeue
operation would require finding and removing the element with the highest priority.
def insert(array, value, priority): index = 0 while index < len(array) and priority > array[index][1]: index += 1 array.insert(index, (value, priority)) def dequeue(array): return array.pop(0)[0]
While this implementation is easy to understand, it has a drawback: the insert
and dequeue
operations have linear time complexity (O(n)) due to the need to shift elements in the array. This can be inefficient for large priority queues.
Binary Heap
A more efficient way to implement a priority queue is by using a binary heap. A binary heap is a complete binary tree that maintains the heap property, where the key of each node is either greater than or equal to (max-heap) or less than or equal to (min-heap) the keys of its children.
In a max-heap, the highest priority element is always the root node. When inserting an element, we add it to the end of the tree and then "bubble up" by swapping it with its parent if it has a higher priority. Similarly, the dequeue
operation involves removing the root node and "bubbling down" to maintain the heap property.
class MaxHeap: def __init__(self): self.heap = [] def insert(self, value, priority): self.heap.append((value, priority)) self.bubble_up(len(self.heap) - 1) def dequeue(self): max_value = self.heap[0][0] self.heap[0] = self.heap.pop() self.bubble_down(0) return max_value # ... additional methods for bubble_up and bubble_down ...
Using a binary heap, the insert
and dequeue
operations have logarithmic time complexity (O(log n)), making it more efficient for large priority queues.
Other Implementations
There are other, more advanced implementations of priority queues, such as Fibonacci heaps and pairing heaps. These data structures offer even better time complexity for certain operations but are more complex to understand and implement.
In Conclusion
Priority queues are versatile data structures that efficiently manage elements based on their priority levels. While there are multiple ways to implement them, choosing the right implementation depends on the specific use case and performance requirements. Armed with this knowledge, you can now confidently tackle problems where prioritization is key!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Common Programming Pitfalls (psst, it's free!).
FAQ
What is a priority queue and how does it differ from a regular queue?
A priority queue is a specialized type of queue data structure in which each element has a priority associated with it. Elements are dequeued or served based on their priority, with the highest priority element being dequeued first. In contrast, a regular queue follows the First-In-First-Out (FIFO) principle, where elements are dequeued in the same order they were enqueued.
What are some common use cases for priority queues?
Priority queues are useful in a variety of applications, such as:
- Task scheduling systems, where tasks with higher priority should be executed before those with lower priority.
- Network traffic management, where packets with higher priority should be transmitted before those with lower priority.
- Graph algorithms such as Dijkstra's shortest path and A* search, where nodes with the lowest cost should be processed first.
How can priority queues be implemented?
Priority queues can be implemented using different data structures, such as:
- Arrays or linked lists: Elements can be stored in a sorted or unsorted manner. However, these implementations may not be efficient for large datasets due to their time complexity in insertion, deletion, and searching operations.
- Binary heaps: A popular and efficient implementation, binary heaps are complete binary trees that maintain the heap property, ensuring the parent nodes have higher priority than their children. The two types of binary heaps are max-heaps and min-heaps.
- Fibonacci heaps, Binomial heaps, and others: These are more advanced data structures that offer better time complexity for various operations.
What are the basic operations of a priority queue?
The basic operations of a priority queue include:
- Enqueue (insertion): Adding an element with a specified priority to the priority queue.
- Dequeue (deletion): Removing and returning the highest priority element from the priority queue.
- Peek: Retrieving the highest priority element without removing it from the priority queue.
- IsEmpty: Checking if the priority queue is empty.
Can you provide an example of a priority queue implementation in Python?
Sure! Here's a simple example using Python's heapq
module, which implements a priority queue using a min-heap:
import heapq # Create an empty priority queue priority_queue = [] # Enqueue elements with their priorities heapq.heappush(priority_queue, (1, "High priority task")) heapq.heappush(priority_queue, (3, "Low priority task")) heapq.heappush(priority_queue, (2, "Medium priority task")) # Dequeue and print the highest priority element highest_priority_element = heapq.heappop(priority_queue) print(highest_priority_element) # Output: (1, "High priority task")
In this example, the priorities are represented by integers, and the lower the integer value, the higher the priority.