Understanding Procedural Generation
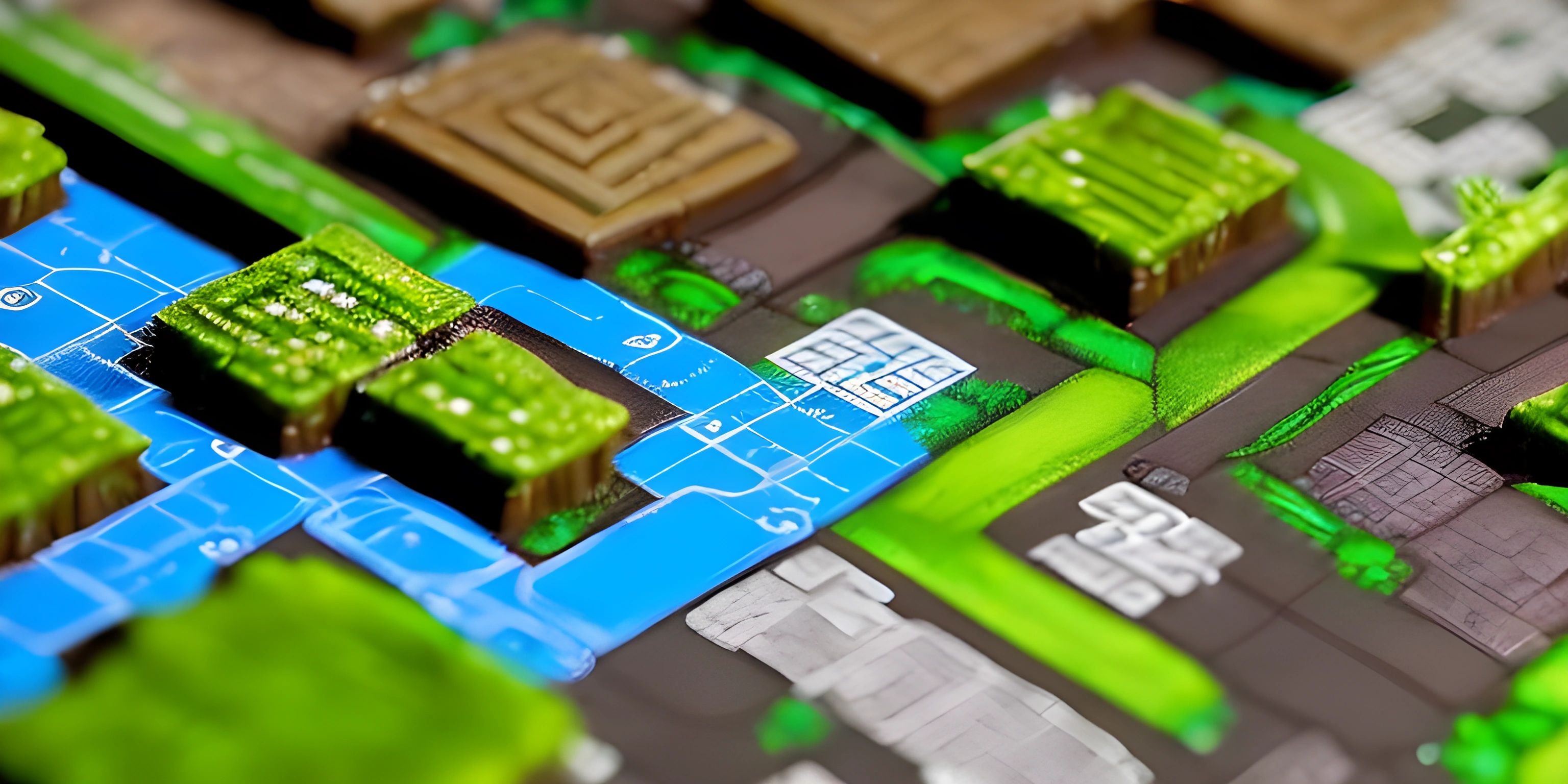
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine you're playing a video game, and every time you start a new game, the world looks different. The mountains, forests, and rivers are all rearranged in a new and exciting way. This isn't the work of some overworked game developer manually designing each world – it's the magic of procedural generation at play!
Procedural generation is like having a digital chef who can whip up a brand-new meal every time you ask, ensuring that no two are ever the same. But instead of ingredients, our chef uses algorithms and randomness to cook up unique content.
What is Procedural Generation?
At its core, procedural generation is a method of creating data algorithmically rather than manually. This data could be anything from textures and levels in video games to entire galaxies in space simulators. The key idea here is the use of algorithms to produce content on the fly, often with an element of randomness to ensure variety.
The Ingredients of Procedural Generation
Just like any good recipe, procedural generation relies on a few key ingredients:
- Algorithms: These are the instructions our computer follows to create content. They can range from simple formulas to complex mathematical functions.
- Randomness: This adds the spice of unpredictability. By introducing randomness, we ensure that the generated content is varied and unique each time.
- Seed Values: Think of this as the starting point for our randomness. Using the same seed value will produce the same output, allowing for reproducibility when needed.
How Does Procedural Generation Work?
Procedural generation typically follows a few steps, much like a recipe:
-
Define Rules and Parameters: This is where we set the boundaries and rules for our generated content. For example, in a game, we might decide that mountains can only appear within certain regions, or that rivers should always flow downhill.
-
Incorporate Randomness: Introduce random elements within the defined rules. This is where our seed values come in. By using different seeds, we get different results each time.
-
Generate the Content: Use our algorithms and randomness to create the content based on the defined rules. This is the fun part where our virtual world comes to life.
Example: Generating Terrain
Let's walk through a simple example of generating terrain. We'll use a basic approach called Perlin noise, which is commonly used for creating natural-looking textures and landscapes.
import numpy as np import matplotlib.pyplot as plt def generate_perlin_noise(width, height, scale): """Generate a 2D grid of Perlin noise.""" def lerp(a, b, x): return a + x * (b - a) def fade(t): return t * t * t * (t * (t * 6 - 15) + 10) def gradient(h, x, y): vectors = np.array([[0,1], [0,-1], [1,0], [-1,0]]) g = vectors[h % 4] return g[:, :, 0] * x + g[:, :, 1] * y lin = np.linspace(0, scale, width, endpoint=False) x, y = np.meshgrid(lin, lin) p = np.arange(256, dtype=int) np.random.shuffle(p) p = np.stack([p,p]).flatten() xi = x.astype(int) yi = y.astype(int) xf = x - xi yf = y - yi u = fade(xf) v = fade(yf) n00 = gradient(p[p[xi] + yi], xf, yf) n01 = gradient(p[p[xi] + yi + 1], xf, yf - 1) n11 = gradient(p[p[xi + 1] + yi + 1], xf - 1, yf - 1) n10 = gradient(p[p[xi + 1] + yi], xf - 1, yf) x1 = lerp(n00, n10, u) x2 = lerp(n01, n11, u) result = lerp(x1, x2, v) return result # Generate and display the terrain width, height, scale = 100, 100, 10 terrain = generate_perlin_noise(width, height, scale) plt.imshow(terrain, cmap="gray") plt.title("Generated Terrain using Perlin Noise") plt.show()
In this example, we use Perlin noise to generate a 2D grid representing terrain. The resulting image shows a landscape with smooth transitions between different heights, mimicking natural terrain.
Applications of Procedural Generation
Procedural generation isn't just limited to games; it has a wide range of applications, including:
- Game Development: From entire worlds in games like Minecraft to random dungeons in roguelikes, procedural generation adds variety and replayability.
- Film and Animation: Creating vast landscapes and detailed textures without manually designing each element.
- Art and Design: Artists use procedural techniques to generate intricate patterns and designs.
- Data Analysis: Generating synthetic data for testing algorithms and models.
Case Study: No Man's Sky
A great example of procedural generation is the game No Man's Sky. This game uses procedural generation to create an entire universe with billions of planets, each with its own unique terrain, flora, and fauna. The developers used sophisticated algorithms to ensure that each planet feels distinct and interesting, while also fitting within the game's overall aesthetic.
Challenges of Procedural Generation
While procedural generation is powerful, it's not without its challenges:
- Quality Control: Ensuring that the generated content is always of high quality and free from glitches can be tough.
- Predictability: Balancing randomness with coherence is important. Too much randomness can lead to chaotic results, while too little can make things repetitive.
- Performance: Generating content on the fly can be computationally expensive, so optimizing algorithms for performance is crucial.
Conclusion
Procedural generation is a fascinating field that combines the rigors of algorithmic thinking with the creativity of content creation. Whether you're a game developer looking to create vast, replayable worlds or an artist seeking to generate intricate patterns, procedural generation offers endless possibilities. So why not dive in and see what unique creations you can come up with?
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Lifetimes (psst, it's free!).
FAQ
What is procedural generation?
Procedural generation is a method of creating data algorithmically rather than manually. It uses algorithms and randomness to produce unique content each time, often used in video games, art, and simulations.
What are the main components of procedural generation?
The main components of procedural generation are algorithms, randomness, and seed values. Algorithms define the rules for content creation, randomness ensures variety, and seed values provide a reproducible starting point.
How does procedural generation work?
Procedural generation involves defining rules and parameters, incorporating randomness, and using algorithms to generate content. The process ensures that content is both varied and adheres to specified rules.
What are some common applications of procedural generation?
Common applications include game development (e.g., creating levels and worlds), film and animation (e.g., generating landscapes), art and design (e.g., intricate patterns), and data analysis (e.g., synthetic data generation).
What are some challenges associated with procedural generation?
Challenges include ensuring quality control, balancing randomness with coherence, and optimizing performance to handle the computational demands of generating content on the fly.