Introduction to Pub/Sub Messaging
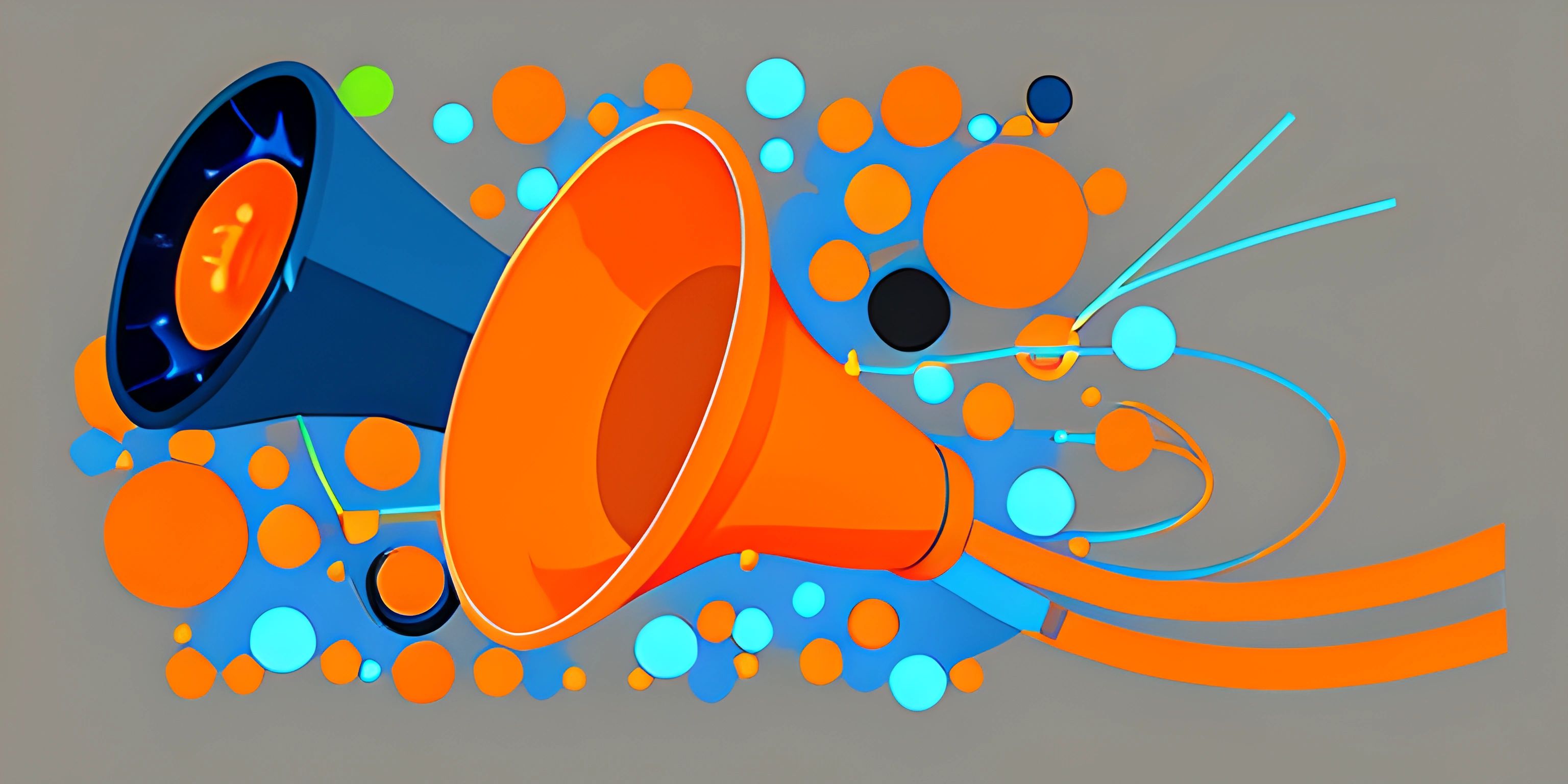
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Pub/Sub messaging, short for publish/subscribe, is a messaging pattern that simplifies communication between different parts of a system. It's like a game of telephone, where one part of the system whispers a message to another, and so on. This pattern allows for more scalable, maintainable, and decoupled systems.
The Pub/Sub Pattern
In the pub/sub pattern, publishers send messages to channels or topics, and subscribers listen to those channels to receive the messages. The beauty of this pattern is that publishers and subscribers are unaware of each other's existence. They communicate indirectly through the shared channels, like ships passing in the night.
Here's an analogy: Imagine a busy train station with multiple trains arriving and departing. The trains (publishers) announce their arrival or departure times over a PA system (channel). The passengers (subscribers) listen to these announcements and act accordingly. The trains and passengers don't directly interact; they communicate through the PA system.
Publishers
Publishers are responsible for producing and sending messages. They don't care who receives those messages or what they do with them. Their sole focus is on publishing information to a specific channel.
# Example of a simple publisher in Python import pubsub pub = pubsub.PubSub() pub.publish("train-arrivals", "Train A arriving at 3:00 PM")
Channels
Channels (or topics) are the middlemen in the pub/sub dance. They act as conduits for messages between publishers and subscribers. Channels are usually identified by a unique name or pattern.
# Example of a channel in Python channel = "train-arrivals"
Subscribers
Subscribers listen to specific channels and act upon the messages they receive. They don't care who sent the message, just that they received it and can process it accordingly.
# Example of a simple subscriber in Python def on_train_arrival(message): print(f"Announcement: {message}") sub = pubsub.PubSub() sub.subscribe("train-arrivals", on_train_arrival)
Advantages of Pub/Sub Messaging
There are several key benefits to using pub/sub messaging in your applications:
- Decoupling: By separating publishers and subscribers, you can change or update one without affecting the other. This makes it easier to maintain and extend your system.
- Scalability: Having a clear separation between publishers and subscribers makes it easier to scale your system. You can add more publishers or subscribers without the need to modify existing components.
- Asynchronous: Pub/sub messaging is inherently asynchronous. This allows your system to handle multiple tasks concurrently, improving performance and responsiveness.
- Event-driven: The pub/sub pattern promotes an event-driven architecture, where components react to events rather than actively polling for updates.
In conclusion, pub/sub messaging is a powerful pattern that can greatly improve the design and flexibility of your applications. By understanding the basics and advantages of this pattern, you'll be well on your way to building more robust and scalable systems. So go ahead, hop on the pub/sub train and enjoy a smoother ride through your software journey!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Chat App Backend (psst, it's free!).