Rust Error Handling
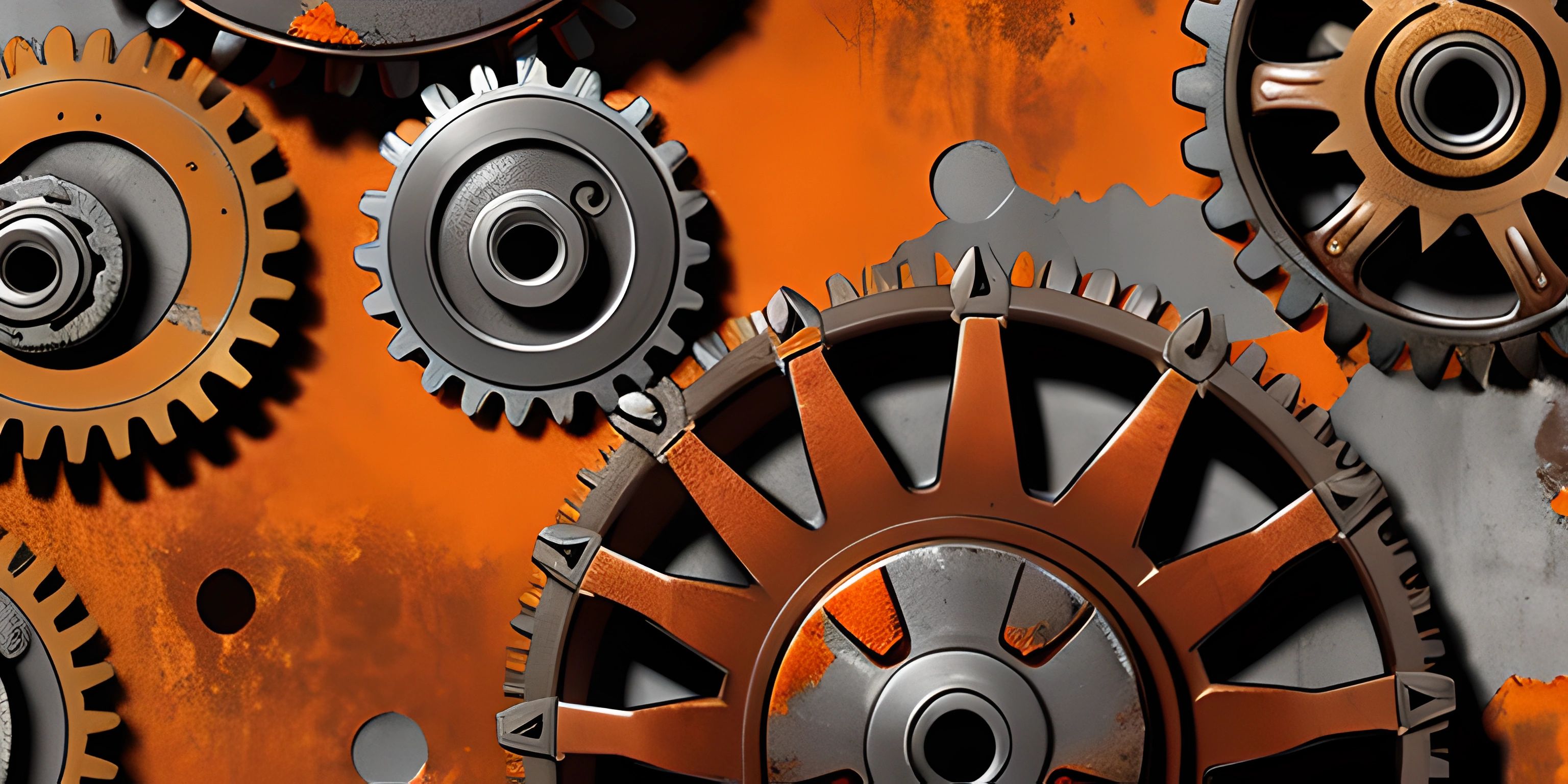
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Error handling is an important aspect of any programming language, and Rust is no exception. In fact, Rust has some unique features that make error handling particularly elegant and expressive. In this whirlwind tour, we'll cover some of the most common techniques and patterns for dealing with errors in Rust, from the basics to advanced tips and tricks. You'll be a Rust error wrangling wizard in no time!
The Result Type
Rust's standard library provides a Result
type, which is an enum with two variants: Ok(T)
and Err(E)
. It's commonly used to represent the result of a computation that can fail. If the computation was successful, the Ok
variant is used, wrapping the result of the computation. If it failed, the Err
variant is used, wrapping an error value that provides more information about the failure.
Here's an example function that reads the contents of a file and returns a Result<String, std::io::Error>
:
use std::fs::File; use std::io::Read; fn read_file_contents(file_name: &str) -> Result<String, std::io::Error> { let mut file = File::open(file_name)?; let mut contents = String::new(); file.read_to_string(&mut contents)?; Ok(contents) }
The ?
operator is used to propagate errors, making error handling more concise and easy to read.
The Option Type
Another useful enum provided by Rust's standard library is Option
. It represents a value that can be either Some(T)
or None
. This is useful for representing situations where a value may or may not be present. It's similar to Result
, but without the error information.
Here's an example of a function that looks up a value in a map and returns an Option<&str>
:
use std::collections::HashMap; fn find_value(map: &HashMap<String, String>, key: &str) -> Option<&str> { map.get(key) }
Unwrapping and Expecting
When dealing with Result
and Option
, you'll often need to extract the underlying value if it's present. Two common methods to do this are unwrap
and expect
.
unwrap
will return the wrapped value if it's an Ok
or Some
, but will panic if it's an Err
or None
. This can be useful for quickly prototyping or when you're sure the value will be present, but it's not recommended for production code.
expect
is similar to unwrap
, but allows you to provide a custom error message that will be displayed if the value is not present. This can help with debugging and understanding why an error occurred.
let value = find_value(&map, "key"); let unwrapped_value = value.unwrap(); // Panics if value is None let expected_value = value.expect("The key was not found in the map"); // Panics with custom message if value is None
Advanced Techniques
There's much more to Rust error handling than can fit in this brief overview. Some advanced topics include:
- Using the
match
expression for more complex error handling logic. - Defining custom error types with the
thiserror
crate. - Applying the
?
operator toOption
to convertNone
values into custom errors.
By mastering these techniques, you'll be well-equipped to handle errors gracefully in your Rust projects!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Enums (psst, it's free!).
FAQ
What are the common error handling techniques in Rust?
Rust offers several error handling techniques to ensure smooth program execution. The most common techniques are:
- The
Result
type: This is an enum with two variants,Ok(T)
andErr(E)
, whereT
andE
are generic types. Functions that may fail typically return aResult
to indicate success or failure. - The
Option
type: This is another enum with two variants,Some(T)
andNone
, used to represent optional values.Option
is used when a function may return a value or nothing at all. - The
match
expression: It's a powerful control structure that allows you to destructure and pattern matchResult
andOption
types, handling different scenarios accordingly. - The
unwrap
andexpect
methods: These are used to extract the value from aResult
orOption
type, but should be used with caution as they can cause the program to panic if an error occurs.
How can I use the `Result` type for error handling in Rust?
When writing a function that may fail, return a Result
type to represent the success or failure of the operation. The Result
type has two variants: Ok(T)
for success and Err(E)
for failure. Here's an example:
fn divide(a: f64, b: f64) -> Result<f64, String> { if b == 0.0 { Err("Cannot divide by zero.".to_string()) } else { Ok(a / b) } }
Use the match
expression to handle the returned Result
:
fn main() { let result = divide(5.0, 0.0); match result { Ok(value) => println!("Result: {}", value), Err(error) => println!("Error: {}", error), } }
What is the difference between `unwrap` and `expect` in Rust error handling?
Both unwrap
and expect
are methods that extract the value from a Result
or an Option
type. The key difference lies in how they handle errors:
unwrap
: If the type isOk(T)
orSome(T)
, it returns the inner valueT
. However, if it's anErr(E)
orNone
, the program will panic and terminate.expect
: Similar tounwrap
, but it takes an additional message as an argument. If the type isErr(E)
orNone
, the program will panic with a custom error message. While both methods can be helpful during development and debugging, they should be used with caution, as they can cause your program to crash if an error occurs. It's often better to handle errors gracefully usingmatch
,if let
, or other error handling techniques.