Deep Dive into Python Functions
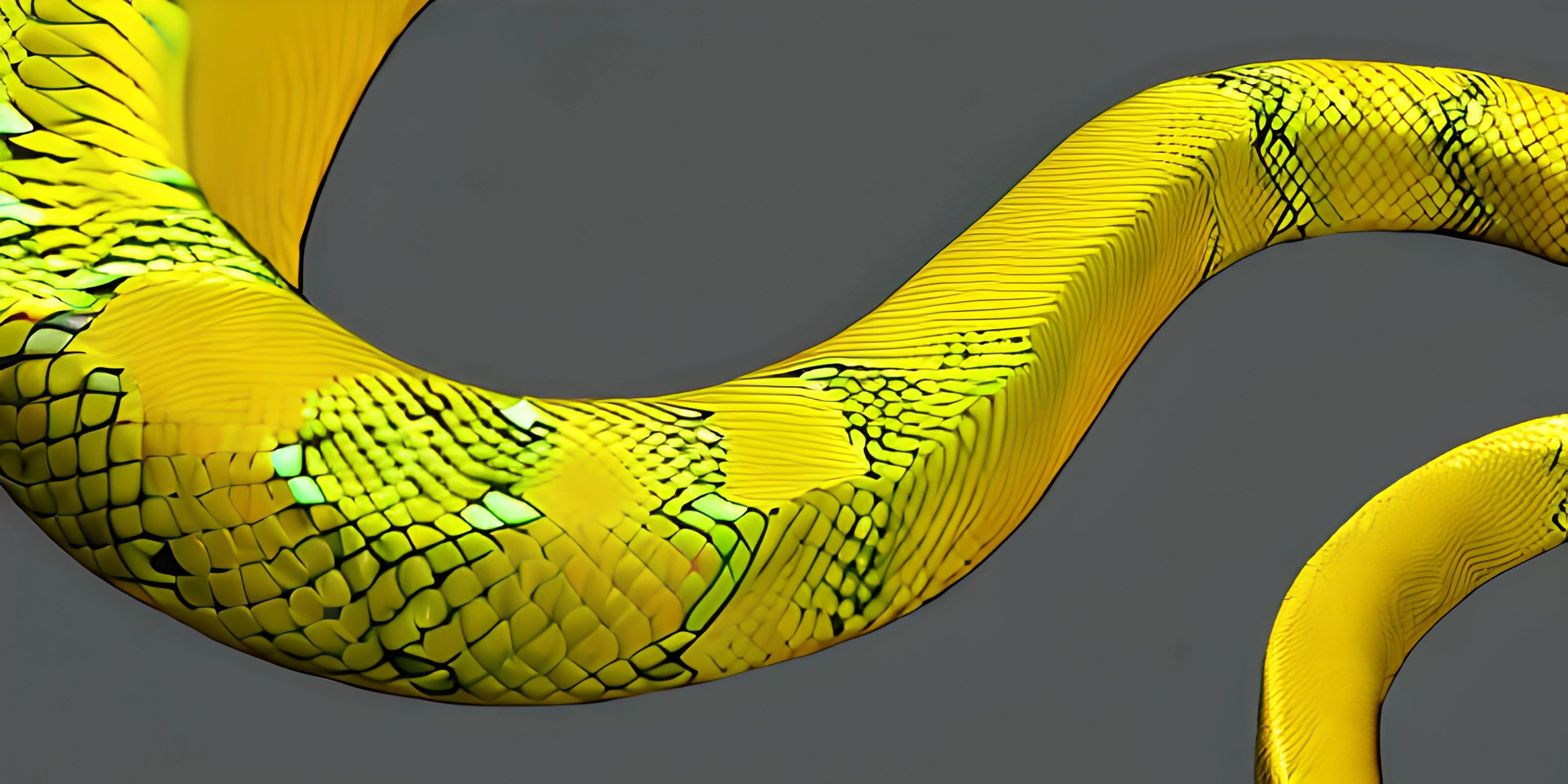
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Welcome to the mesmerizing world of Python functions! Functions are the building blocks of any Python program and serve as reusable pieces of code. They help break down complex tasks and keep code clean and organized. So, let's dive in and explore the depths of Python functions!
Defining Functions
In Python, a function is defined using the def
keyword, followed by the function name, a pair of parentheses, and a colon. The function body is indented, and typically includes a return
statement to send back the result.
Here's a simple example of a Python function that adds two numbers:
def add_numbers(a, b): result = a + b return result sum = add_numbers(4, 5) print(sum) # Output: 9
This function takes two parameters, a
and b
, and returns their sum. We call the function by providing the arguments and storing the result in the sum
variable.
Function Arguments
Python functions support various types of arguments, including positional, keyword, default, and variable-length arguments. Let's explore each type.
Positional Arguments
Positional arguments are the most basic type of arguments. They're passed in the order they're defined in the function signature:
def greet(name, greeting): return f"{greeting}, {name}!" message = greet("Alice", "Hello") print(message) # Output: Hello, Alice!
Keyword Arguments
Keyword arguments allow you to pass arguments using the parameter names. This can make the code more readable and less error-prone:
message = greet(name="Alice", greeting="Hello") print(message) # Output: Hello, Alice!
Default Arguments
You can provide default values for parameters using the assignment operator (=
) in the function definition. This makes the corresponding arguments optional:
def greet(name, greeting="Hello"): return f"{greeting}, {name}!" message = greet("Alice") print(message) # Output: Hello, Alice! message = greet("Alice", "Hi") print(message) # Output: Hi, Alice!
Variable-length Arguments
Variable-length arguments allow a function to accept an arbitrary number of arguments. You can use the asterisk (*
) for positional arguments and the double asterisk (**
) for keyword arguments:
def print_args(*args, **kwargs): print("Positional arguments:", args) print("Keyword arguments:", kwargs) print_args(1, 2, 3, a=4, b=5) # Output: # Positional arguments: (1, 2, 3) # Keyword arguments: {'a': 4, 'b': 5}
Recursive Functions
A recursive function is one that calls itself to solve a problem. Recursion can be a powerful technique, especially when solving problems that have a natural tree-like structure. Here's an example of a recursive function to compute the factorial of a number:
def factorial(n): if n == 0: return 1 else: return n * factorial(n - 1) result = factorial(5) print(result) # Output: 120
Be careful with recursion, though, as it can lead to a stack overflow if the function calls itself too many times.
Conclusion
Python functions are versatile and powerful tools in a programmer's arsenal. By understanding how to create and use them effectively, you can write clean, modular, and efficient code. So go forth and function-ize your Python programs!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).