Python Classes: A Comprehensive Guide
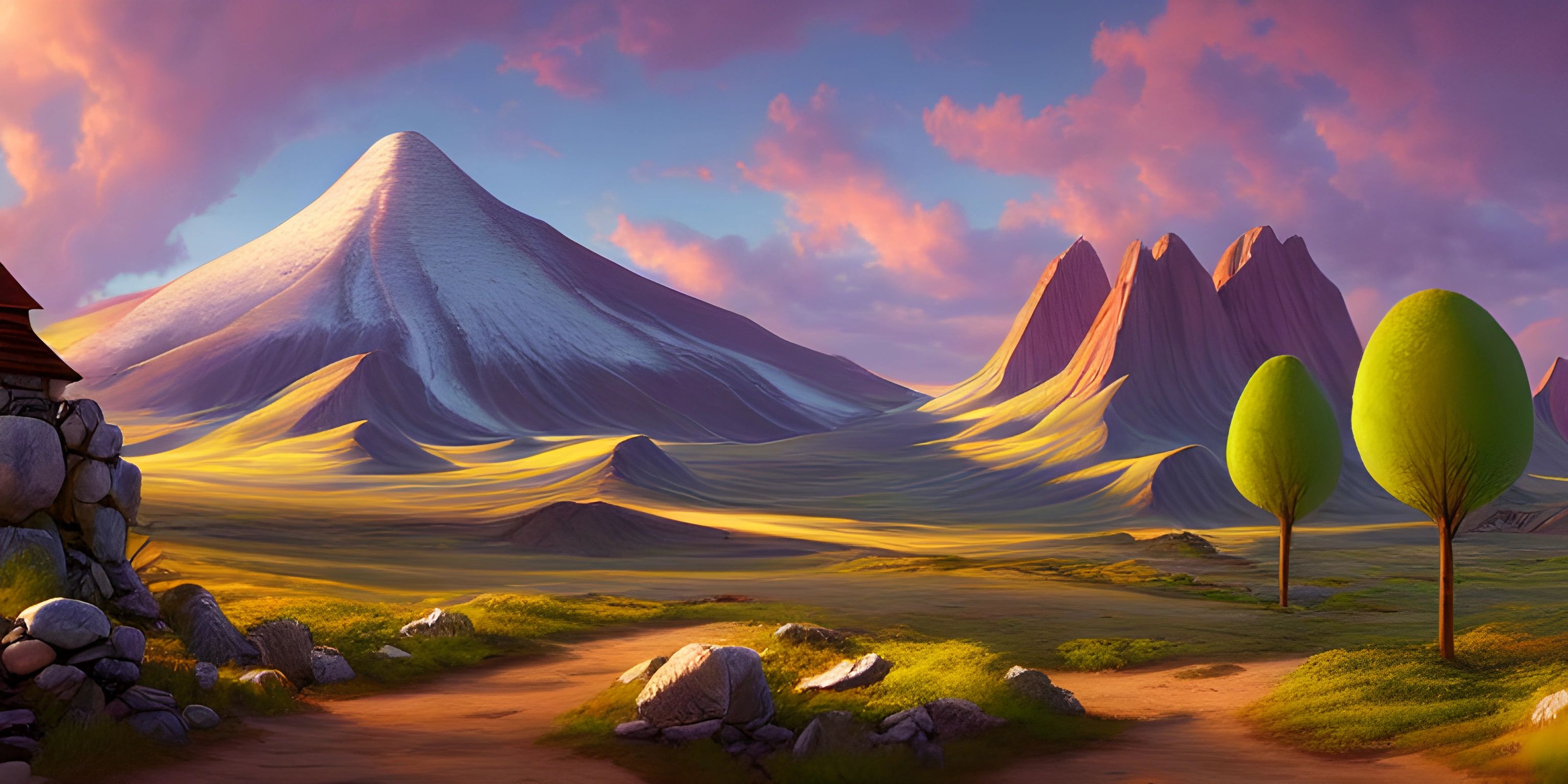
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Let's embark on a journey into the magical world of Python classes. Imagine Python classes as blueprints for creating your own objects. They are a fundamental concept in Object-Oriented Programming (OOP), which allows us to model real-world entities. If you've ever dreamt of creating your own world with wizards, dragons, or even an army of robots, classes are the way to go!
What Exactly is a Class?
A class in Python is a blueprint for creating objects. Think of it as a cookie cutter, and the cookies are the objects created from this cutter. Each cookie can have unique features, but they all come from the same cutter.
Here's a simple example of a class:
class Dog: # Class attribute species = "Canis familiaris" def __init__(self, name, age): # Instance attributes self.name = name self.age = age # Creating an instance of the Dog class my_dog = Dog("Buddy", 3) print(my_dog.name) # Output: Buddy print(my_dog.age) # Output: 3 print(my_dog.species) # Output: Canis familiaris
Breaking Down the Example
The __init__
Method
The __init__
method is a special method that initializes an object's attributes. It's like a constructor in other programming languages. When you create an instance of a class, __init__
sets up the initial state of the object.
In our example, when we create my_dog
, Python calls the __init__
method with the arguments "Buddy"
and 3
, setting name
and age
respectively.
Attributes
Attributes are variables that belong to an object. They can be either class attributes (shared by all instances of the class) or instance attributes (unique to each instance).
In our example:
species
is a class attribute, meaning every instance ofDog
will have the samespecies
.name
andage
are instance attributes, unique to eachDog
instance.
Methods
Methods are functions defined inside a class that describe the behaviors of the object. They are similar to functions but are called on instances of the class.
Here’s an example:
class Dog: species = "Canis familiaris" def __init__(self, name, age): self.name = name self.age = age def bark(self): return f"{self.name} says woof!" # Creating an instance of the Dog class my_dog = Dog("Buddy", 3) print(my_dog.bark()) # Output: Buddy says woof!
In this example, bark
is a method that returns a string indicating that the dog is barking.
Inheritance: Reusing Code Like a Pro
Inheritance allows a class to inherit attributes and methods from another class. This helps in reusing code and creating a hierarchical relationship between classes.
Here's an example of inheritance:
class Animal: def __init__(self, name): self.name = name def speak(self): raise NotImplementedError("Subclasses must implement this method") class Dog(Animal): def speak(self): return f"{self.name} says woof!" class Cat(Animal): def speak(self): return f"{self.name} says meow!" # Creating instances of Dog and Cat dog = Dog("Buddy") cat = Cat("Whiskers") print(dog.speak()) # Output: Buddy says woof! print(cat.speak()) # Output: Whiskers says meow!
In this example, both Dog
and Cat
inherit from the Animal
class. Each subclass implements its own version of the speak
method.
Encapsulation: Keeping Things Safe
Encapsulation is the concept of wrapping data and methods that work on data within one unit, such as a class. It restricts direct access to some of the object's components, which can prevent accidental interference and misuse.
In Python, we use underscores to indicate private attributes and methods:
class Car: def __init__(self, make, model): self.make = make self.model = model self._secret = "Hidden feature" def _display_secret(self): return self._secret # Creating an instance of Car my_car = Car("Toyota", "Corolla") print(my_car.make) # Output: Toyota print(my_car._display_secret()) # Output: Hidden feature
Polymorphism: One Interface, Many Implementations
Polymorphism lets us use a unified interface for different data types. It allows functions or methods to use objects of various types at different times.
Here’s an example:
class Bird: def make_sound(self): return "Chirp" class Dog: def make_sound(self): return "Bark" def animal_sound(animal): print(animal.make_sound()) # Creating instances of Bird and Dog sparrow = Bird() golden_retriever = Dog() animal_sound(sparrow) # Output: Chirp animal_sound(golden_retriever) # Output: Bark
In this example, both Bird
and Dog
classes have a make_sound
method. The animal_sound
function can take any object that implements the make_sound
method, demonstrating polymorphism.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What is a class in Python?
A class in Python is a blueprint for creating objects. It allows us to define the structure and behavior of the objects we want to create. Classes help in modeling real-world entities by grouping related attributes and methods together.
What is the purpose of the `__init__` method?
The __init__
method initializes an object's attributes and sets up its initial state. It's called automatically when a new instance of a class is created. It's akin to a constructor in other programming languages.
How does inheritance work in Python?
Inheritance allows a class to inherit attributes and methods from another class, promoting code reuse. The subclass can add its own attributes and methods or override those of the parent class. This creates a hierarchical relationship between classes.
What is encapsulation, and how is it implemented in Python?
Encapsulation is the concept of bundling data and methods that operate on that data within one unit, like a class. It restricts direct access to some components to prevent accidental interference. In Python, underscores are used to indicate private attributes and methods.
Can you explain polymorphism with an example?
Polymorphism allows us to use a unified interface for different data types, enabling functions or methods to operate on objects of various types. For example, different classes can have the same method name, and a function can call these methods on objects of those classes, demonstrating polymorphism.