Queue Basics
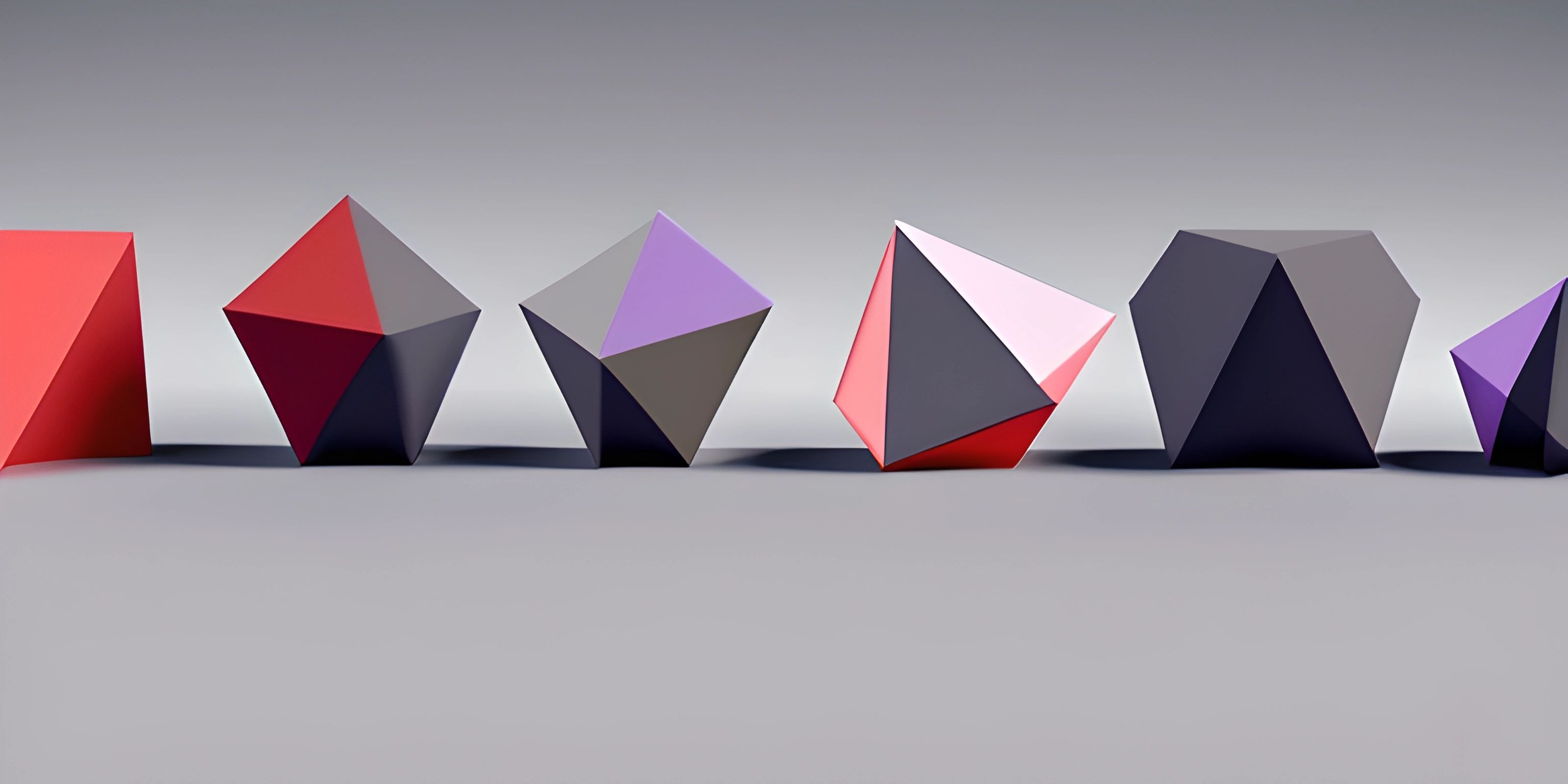
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Queue up, folks! In the programming world, a queue is a popular data structure that gets its name from our everyday experience of standing in lines (or "queues") for various services. It follows the First-In-First-Out (FIFO) principle, which means that the first element added to the queue will be the first one to leave.
Characteristics of a Queue
Queues are dynamic data structures, meaning they can grow and shrink as elements are added and removed. They have two primary operations: enqueue and dequeue.
Enqueue
When we add an element to the queue, we perform the enqueue operation. This adds the new element to the back (or "rear") of the queue.
Dequeue
To remove an element from the queue, we perform the dequeue operation. This removes the element from the front (or "head") of the queue.
Here's a simple analogy: Think of a queue as a conveyor belt at a grocery store checkout. As items are placed on the belt, they move towards the cashier (head), while new items are added to the back (rear). The cashier scans the items in the order they arrive, following the FIFO principle.
Queue Implementation
Queues can be implemented using various data structures, such as arrays, linked lists, or collections (in languages like Java or C#). The choice of implementation depends on the language and problem requirements. Let's take a look at a simple queue implementation using an array in Python:
class Queue: def __init__(self): self.queue = [] def enqueue(self, item): self.queue.append(item) def dequeue(self): if len(self.queue) < 1: return None return self.queue.pop(0) def size(self): return len(self.queue)
In this example, we define a Queue
class, which has an internal array named queue
. The enqueue
method appends an item to the end of the array, and the dequeue
method removes and returns the first item of the array. The size
method returns the total number of elements in the queue.
Use Cases and Limitations
Queues are commonly used in scenarios where we need to maintain order or serve requests in the order they arrive. Some examples include:
- Task scheduling: Queues help manage tasks in the order they were received or based on priority.
- Print spooling: Printer queues store print jobs in order, ensuring that they are printed sequentially.
- Message handling: Queues can be used to handle messages between different parts of a system, ensuring the order of delivery.
However, queues have some limitations, such as inefficient element access and the lack of a search function. Therefore, they are not suitable for all problem scenarios. It's essential to choose the right data structure based on the specific requirements of your programming task.
In summary, queues are a powerful and versatile data structure, following the FIFO principle with enqueue and dequeue operations. Understanding the basics of queues will give you a solid foundation for solving various programming problems and working with more advanced data structures.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Basic Concepts (psst, it's free!).
FAQ
What is a queue data structure?
A queue is a linear data structure that follows the First-In-First-Out (FIFO) principle, meaning the first element added to the queue will be the first one to be removed. It is similar to a real-life queue where people line up for a service, and the person who arrives first gets served first.
How do you perform enqueue and dequeue operations in a queue?
Enqueue is the process of adding an element to the rear of the queue, while dequeue is the process of removing the front element from the queue. Here's a simple example in Python:
class Queue: def __init__(self): self.items = [] def enqueue(self, item): self.items.append(item) def dequeue(self): if not self.is_empty(): return self.items.pop(0) def is_empty(self): return len(self.items) == 0
What are some common applications of queues?
Queues are commonly used in various applications, such as:
- Managing tasks in an operating system's scheduler.
- Handling requests in a web server.
- Simulating real-life scenarios like a supermarket checkout line or a printer queue.
- Buffering data in communication processes.
What is the time complexity of enqueue and dequeue operations in a queue?
The time complexity of enqueue operation is generally O(1) as it involves adding an element to the end of the queue. However, the time complexity of dequeue operation can vary depending on the implementation. In a standard list-based implementation, the dequeue operation may have a time complexity of O(n) due to the shifting of the remaining elements. To achieve constant-time dequeue operations, O(1), you can use a doubly-linked list or a circular buffer as the underlying data structure.
What is the difference between a queue and a stack?
A queue follows the First-In-First-Out (FIFO) principle, whereas a stack follows the Last-In-First-Out (LIFO) principle. In a queue, the first element added is the first one to be removed, while in a stack, the last element added is the first one to be removed. Both are linear data structures but differ in how they manage the addition and removal of elements.