Understanding Queues and Their Applications
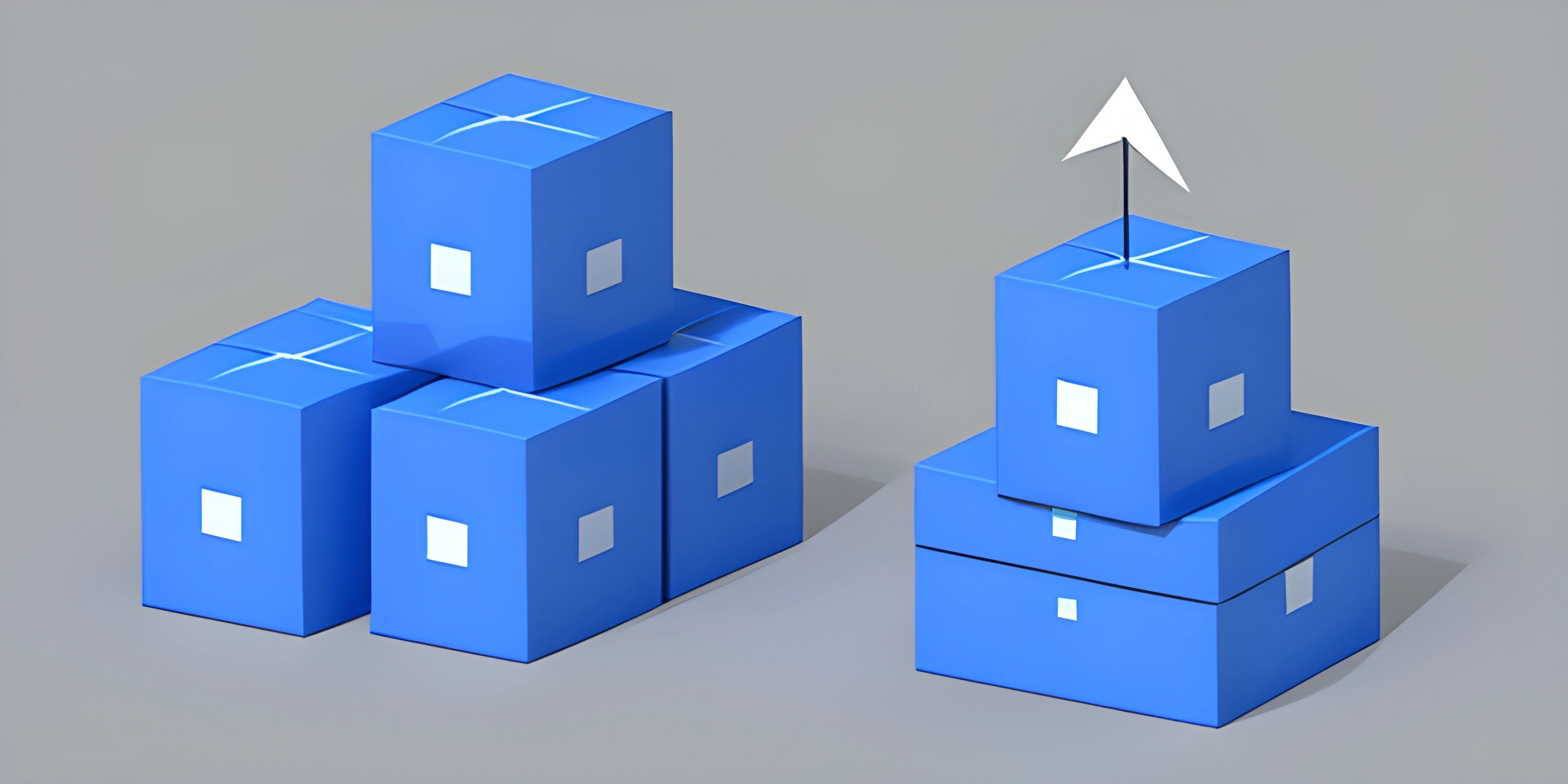
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Let's dive into the world of queues! No, not the ones you stand in while waiting for your morning coffee. We're talking about queues as a data structure in programming. Queues are an essential concept to grasp, as they come in handy in various coding scenarios.
Queues: The Basics
Picture a line of people waiting at a bus stop. The person who arrives first gets on the bus first, and the others follow in the order they arrived. This is the basic concept of a queue, and it follows the First In, First Out (FIFO) principle. In programming, a queue is a data structure that stores elements in a linear arrangement and supports two primary operations: enqueue and dequeue.
Enqueue
The enqueue operation adds an element to the end of the queue, just like a person joining the line at the bus stop. Here's a simple example using Python:
def enqueue(queue, element): queue.append(element)
Dequeue
The dequeue operation removes the element at the front of the queue, similar to the person at the front of the line boarding the bus. In code, it looks like this:
def dequeue(queue): if not is_empty(queue): return queue.pop(0)
Note that we also check if the queue is empty before dequeuing, as it's important not to attempt a dequeue operation on an empty queue.
Applications of Queues
Now that we've got the basics down, let's explore some applications of queues in different programming scenarios.
Task Scheduling
One common use of queues is for managing tasks in a system. Let's say you're building a print manager that processes multiple print jobs. You could use a queue to store the print jobs in the order they are received, and your print manager would process each job in the order they were added to the queue.
Web Server Requests
Queues also play a crucial role in handling web server requests. When multiple users access a website simultaneously, their requests are queued and processed in a FIFO manner. This ensures that the server doesn't get overwhelmed and maintains a fair processing order for users' requests.
Buffering
Have you ever wondered how video streaming services like YouTube manage to play videos smoothly even when your internet connection is slow? They use a technique called buffering, where a queue is used to store chunks of video data. The video is played from the front of the queue while new data is continuously added to the end. This helps maintain a smooth playback experience without any hiccups.
Wrapping Up
In a nutshell, queues are a versatile data structure that serves a wide range of applications in programming, from task scheduling to buffering. Understanding the concept of queues, their basic operations, and how they are applied in various programming scenarios is vital for any coder. So, the next time you find yourself in a real-life queue, you can impress your fellow queuers with your newfound knowledge of programming queues!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).
FAQ
What is a queue and how does it work?
A queue is a linear data structure that follows the First-In-First-Out (FIFO) principle, meaning the first element added to the queue will be the first one to be removed. It operates much like a real-life queue, such as waiting in line at a grocery store checkout. The main operations of a queue are enqueue (adding an element to the rear of the queue) and dequeue (removing an element from the front of the queue).
How can I implement a queue in Python?
You can implement a queue in Python using the list
data structure or the Queue
class available in the queue
module. Here's a basic implementation using a list:
class Queue: def __init__(self): self.items = [] def is_empty(self): return not bool(self.items) def enqueue(self, item): self.items.append(item) def dequeue(self): return self.items.pop(0) def size(self): return len(self.items)
What are the primary operations associated with queues?
The two primary operations associated with queues are:
- Enqueue: Adding an element to the rear of the queue.
- Dequeue: Removing an element from the front of the queue. Additionally, some other common operations include checking if the queue is empty and finding the size of the queue.
Can you provide an example of a real-world scenario where a queue can be applied?
Sure! One real-world example of a queue is job scheduling in a printer. Multiple print jobs are sent to the printer, and the printer maintains a queue of these jobs. The first job sent to the printer is completed first, followed by the next job in line, and so on. This follows the FIFO principle, which is the essence of a queue data structure.
What is the difference between a queue and a stack?
The main difference between a queue and a stack is their order of processing elements. A queue follows the First-In-First-Out (FIFO) principle, where the oldest element is removed first. On the other hand, a stack follows the Last-In-First-Out (LIFO) principle, where the newest element is removed first. In other words, a queue operates like a real-life queue (e.g., waiting in line), while a stack operates like a stack of plates, where the top plate is the first to be removed.