Using React Hooks for State and Side Effects
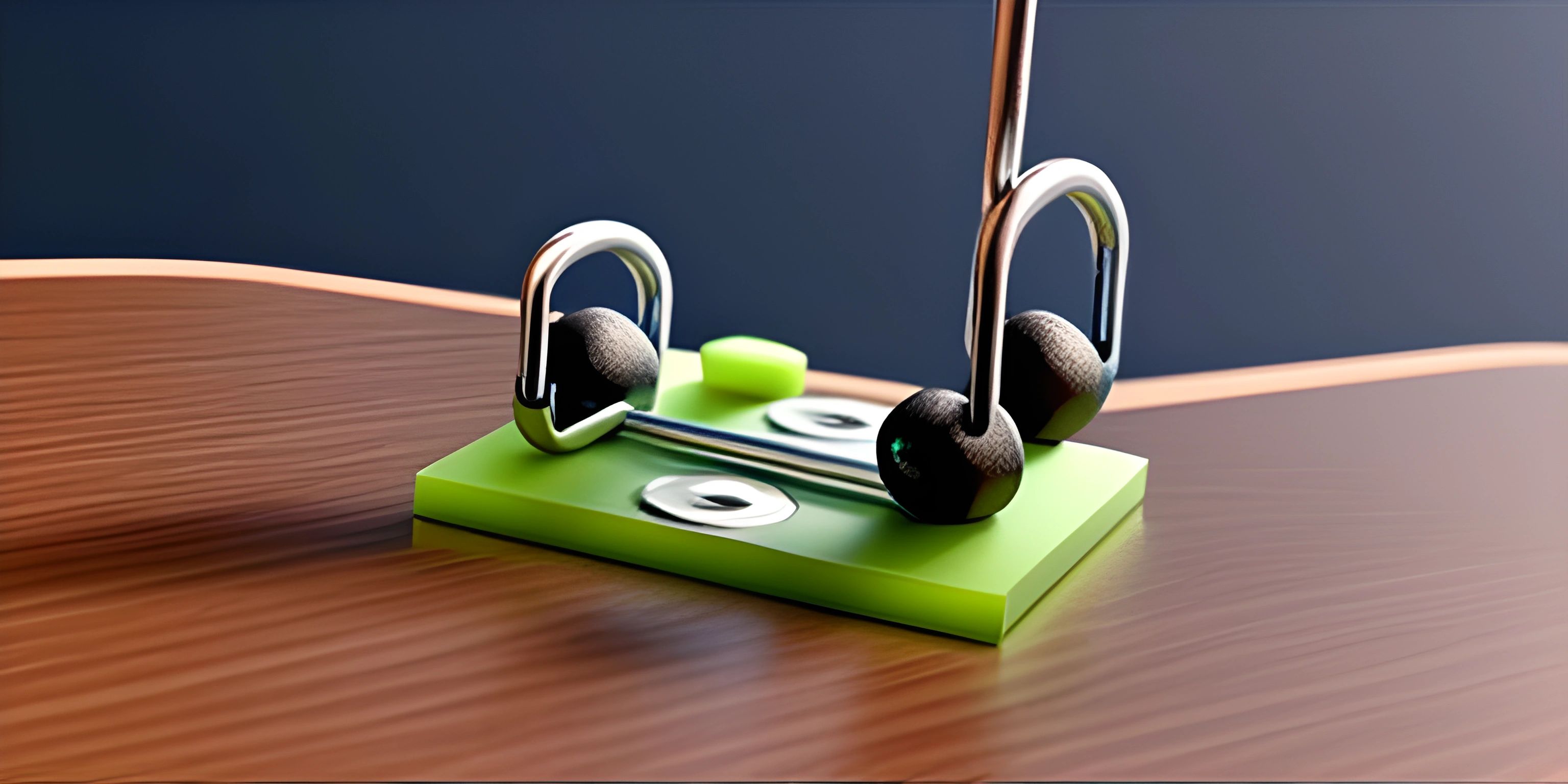
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
React Hooks are a fantastic addition to the React library, enabling developers to handle state and side effects in functional components. No longer do we need to rely on class components and their often-confusing this
keyword. Instead, we can write cleaner, more understandable code that's easier to test and maintain. So let's dive into the world of React Hooks and see how they can level up our development game.
useState Hook
The first hook we'll explore is useState
. This hook allows us to add and manage state in a functional component. Before hooks, this was only possible in class components using this.state
and this.setState
. Now, with useState
, we can have a stateful functional component with just a few lines of code.
To use useState
, first, we need to import it from the 'react' package:
import React, { useState } from "react";
Now we can use it inside our functional component:
function Counter() { const [count, setCount] = useState(0); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increase Count</button> </div> ); }
Here's how it works:
- We call
useState
with an initial state value (0 in this case). useState
returns an array with two elements: the current state value and a function to update that value.- We use array destructuring to assign these elements to variables:
count
andsetCount
. - Inside the component, we display the current state value (
count
) and use the update function (setCount
) to change the state when the button is clicked.
That's it! We have a fully functional stateful component, without the need for a class.
useEffect Hook
Next, let's talk about side effects. Side effects are actions like network requests, DOM manipulations, or subscriptions that typically happen outside of the React component lifecycle. In class components, these were handled using lifecycle methods like componentDidMount
, componentDidUpdate
, and componentWillUnmount
. With hooks, we can now use the useEffect
hook to handle these side effects in a cleaner and more efficient way.
As with useState
, we need to import useEffect
from the 'react' package:
import React, { useEffect } from "react";
Now, let's see how we can use useEffect
to fetch data from an API and display it in our component:
import React, { useState, useEffect } from "react"; function DataFetcher() { const [data, setData] = useState([]); const [loading, setLoading] = useState(true); useEffect(() => { fetch("https://api.example.com/data") .then((response) => response.json()) .then((data) => { setData(data); setLoading(false); }); }, []); if (loading) { return <p>Loading data...</p>; } return ( <div> {data.map((item) => ( <p key={item.id}>{item.name}</p> ))} </div> ); }
Here's how useEffect
works in this example:
- We set up an effect function that fetches data from an API.
- We pass this function to
useEffect
as its first argument. - The second argument is an empty dependency array (
[]
). This tells React to run the effect only once, when the component mounts. If we wanted the effect to run whenever some variable changes, we would include that variable in the dependency array. - Inside the effect, we fetch data, update the state with
setData
, and setloading
to false when the data is fetched.
With useEffect
, we can handle side effects in a much more efficient and declarative way than before.
Conclusion
React Hooks have genuinely revolutionized how we write React components. With useState
and useEffect
, we can now build stateful components and handle side effects in functional components, leading to cleaner, more maintainable code. So go forth and explore the world of React Hooks – your components will thank you!