Rust Borrowing
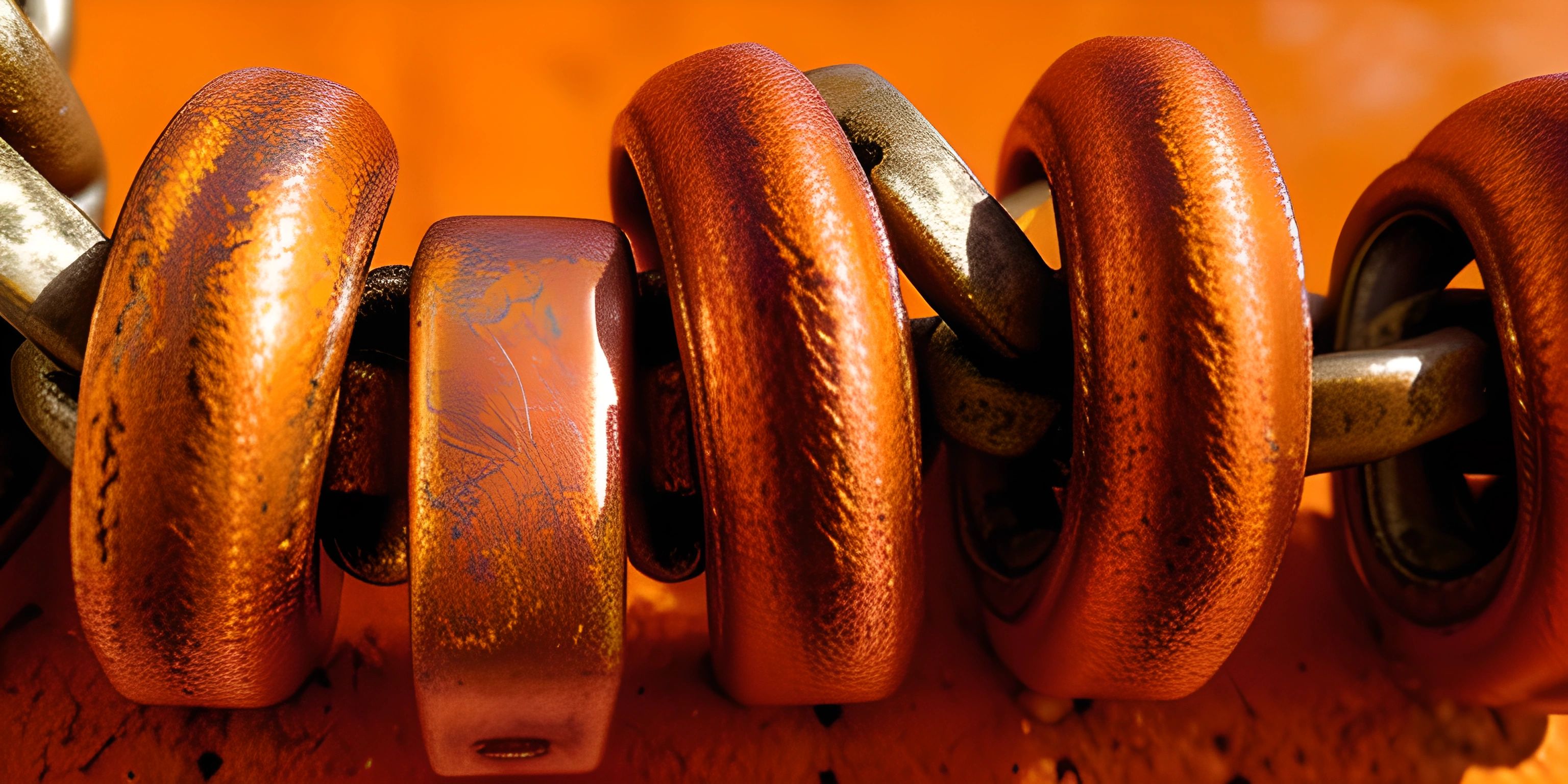
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Borrowing is a core concept in Rust that ensures memory safety without compromising on performance. It's like lending a book to a friend: while they're reading it, you can't make changes or give it to someone else. Let's explore how borrowing helps Rust guarantee safety and understand the rules that govern it.
What is Borrowing?
In Rust, borrowing allows one part of your code to temporarily use a value without taking ownership. This ensures that no other part of the code can access or modify the borrowed value during the borrowing period, maintaining memory safety.
Borrowing comes in two flavors: mutable and immutable.
Immutable Borrowing
Immutable borrowing allows multiple parts of your code to read a value without changing it. It's denoted using an ampersand (&
). Here's an example:
fn main() { let s1 = String::from("hello"); let len = calculate_length(&s1); println!("The length of '{}' is {}.", s1, len); } fn calculate_length(s: &String) -> usize { s.len() }
In this example, we pass a reference to s1
(an immutable borrow) to the calculate_length
function. This allows the function to read the value without taking ownership, ensuring that s1
remains accessible in main
.
Mutable Borrowing
Mutable borrowing allows one part of your code to temporarily modify a value. It's denoted using an ampersand followed by the mut
keyword (&mut
). Here's an example:
fn main() { let mut s = String::from("hello"); change(&mut s); println!("Modified string: {}", s); } fn change(s: &mut String) { s.push_str(", world!"); }
In this example, we pass a mutable reference to s
(a mutable borrow) to the change
function. This allows the function to modify the value without taking ownership, ensuring that s
remains accessible in main
.
Borrowing Rules
To maintain memory safety, Rust enforces the following rules for borrowing:
-
At any given time, you can have either one mutable reference or any number of immutable references. This prevents data races, where multiple parts of your code attempt to access and modify a value simultaneously.
-
References must always be valid. Borrowed values cannot outlive the values they reference, ensuring that you never have a dangling reference.
By following these rules, Rust ensures memory safety without the need for a garbage collector, providing you with blazing-fast performance.
Conclusion
Borrowing is the secret sauce that makes Rust a powerful and safe language. It allows you to temporarily access values without taking ownership, and its strict rules guarantee memory safety. Now that you have a grasp on borrowing, you can confidently write Rust code that's both safe and efficient. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Ownership and Borrowing (psst, it's free!).
FAQ
What is borrowing in Rust?
Borrowing in Rust is a mechanism that allows you to temporarily access the value of a variable without taking ownership of it. It plays a crucial role in memory safety, as it ensures that data is accessed in a safe and controlled manner, adhering to Rust's strict ownership rules.
How do I borrow a variable in Rust?
To borrow a variable in Rust, you can use the ampersand (&
) symbol, which creates a reference to the original variable. Here's an example in Rust:
fn main() { let s = String::from("hello world"); let len = calculate_length(&s); println!("The length of '{}' is {}.", s, len); } fn calculate_length(s: &String) -> usize { s.len() }
In this example, we pass a reference to the String
object s
to the calculate_length
function, which then returns its length. The original s
remains unchanged and accessible after the function call.
What are mutable and immutable borrows?
In Rust, there are two types of borrows: mutable borrows and immutable borrows. Immutable borrows, which are created using &variable_name
, allow you to access the value of a variable without modifying it. Mutable borrows, denoted by &mut variable_name
, permit you to modify the value of a borrowed variable. However, Rust enforces strict rules to maintain memory safety:
- There can be either one mutable borrow or multiple immutable borrows in the same scope.
- Mutable and immutable borrows cannot coexist in the same scope.
Can I have multiple mutable borrows in Rust?
No, Rust's ownership rules prevent you from having multiple mutable borrows of the same variable in the same scope. This rule exists to guarantee memory safety and avoid issues such as data races. If you need multiple mutable borrows, you might need to restructure your code or use other mechanisms such as RefCell
or Mutex
from the Rust standard library.
What is the difference between borrowing and slicing in Rust?
Borrowing is a general concept in Rust that allows you to temporarily access a variable's value without taking ownership of it. Slicing, on the other hand, is a more specific operation applied to collections like strings, arrays, and vectors. Slicing creates a view of a contiguous range within a collection, without modifying the original data. While both borrowing and slicing allow you to access data without modifying the original variable, slicing is specifically used to access a range of elements within a collection, whereas borrowing is used to temporarily access the value of any variable.