Introducing Rust Language
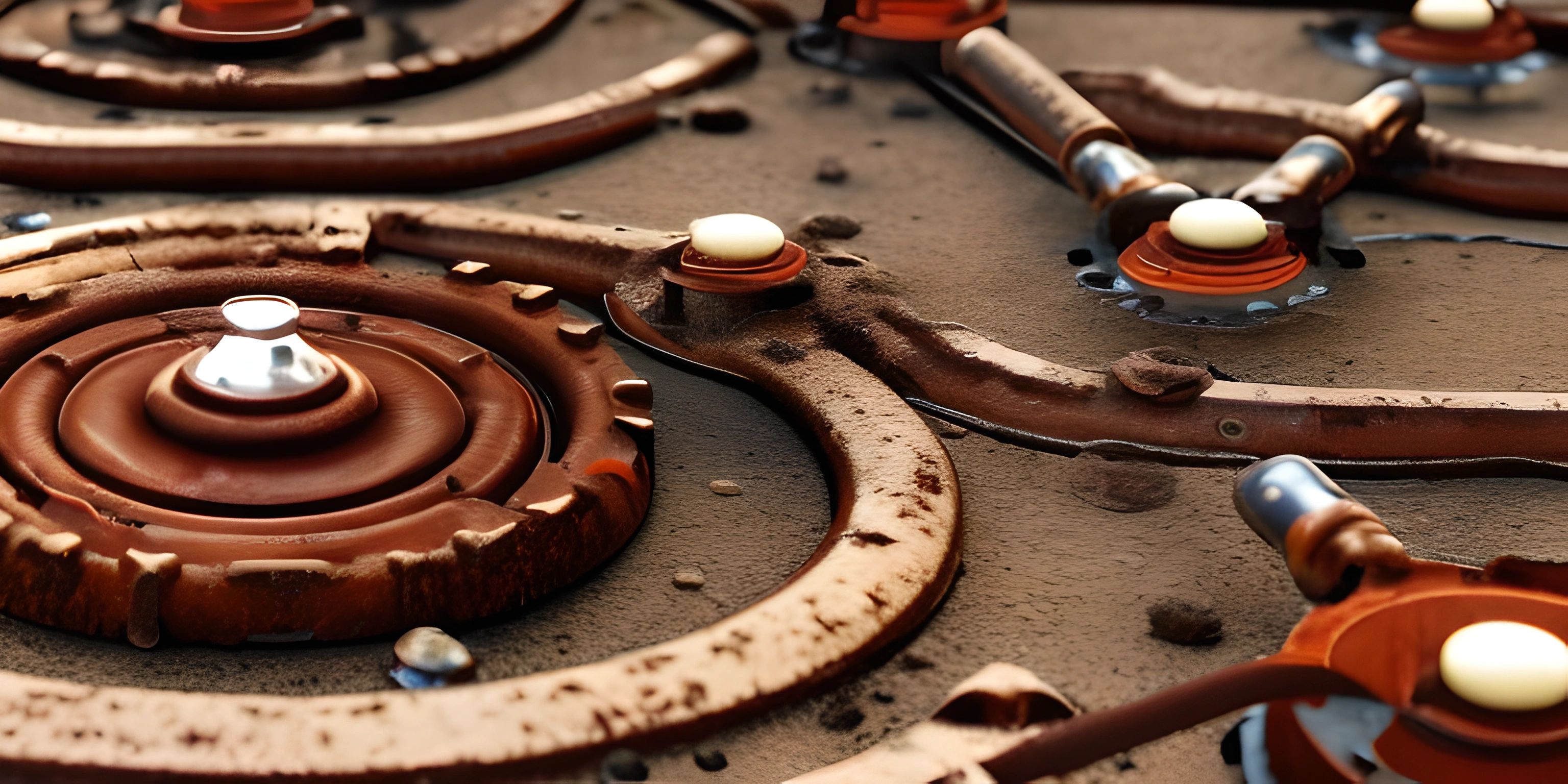
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Rust is a systems programming language that puts safety, concurrency, and performance at the forefront of its design. It empowers you to build fast, reliable, and efficient software while preventing common bugs like segmentation faults and data races. Rust's unique approach to memory management and its strong compile-time checks make it an attractive option for any programming project where safety and performance are a priority.
Why Rust?
In the world of programming languages, Rust stands out for a few key reasons:
Safety
Rust's main focus is on safety. It achieves this by employing a concept called ownership with a strong type system and compile-time checks. The ownership system guarantees that resources like memory are properly managed, which helps to eliminate common bugs such as null pointers and buffer overflows.
Concurrency
Another major advantage of Rust is its support for concurrency. Concurrency refers to the ability of a program to execute multiple tasks at the same time. Rust's ownership model ensures that data races, a common source of bugs in concurrent programs, are caught during compilation, making it much easier to write safe and efficient concurrent code.
Performance
Rust is designed for performance, providing fine-grained control over system resources and low-level hardware. It does this without the need for a garbage collector or runtime, which translates to fast and predictable execution of your programs.
A Taste of Rust
Let's take a look at a simple "Hello, World!" program in Rust:
fn main() { println!("Hello, World!"); }
In this example, fn main()
is the entry point of the program. The println!
macro is used to print the string "Hello, World!" to the console. Rust uses macros (denoted with a !
after the name) for code generation and other compile-time tasks.
Variables and Data Types
Rust has a strong static type system which means that all variables must have a type explicitly defined or inferred by the compiler. Here's how you declare a variable in Rust:
let x: i32 = 5;
In this example, we declare a variable named x
of type i32
(a 32-bit integer) and assign it the value 5. We can also let the compiler infer the type for us:
let y = 10;
In this case, the compiler will infer that y
is of type i32
based on the assigned value.
Functions
Functions in Rust are defined with the fn
keyword, followed by the function name, a parenthesized list of input parameters, a return type, and a block of code. Here's a simple example:
fn add(a: i32, b: i32) -> i32 { a + b }
This function takes two i32
parameters, a
and b
, and returns the sum of the two as an i32
. The last expression in a function is automatically returned, or you can use the return
keyword to return early.
Dive Deeper into Rust
Now that you've had a glimpse of what Rust is all about, it's time to dive deeper and explore its features and capabilities. Check out these in-depth articles to learn more about specific topics in Rust:
- Understanding Ownership in Rust
- Error Handling in Rust
- Concurrency in Rust
- Working with Modules and Packages in Rust
Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is Rust language and why should I learn it?
Rust is a systems programming language that focuses on safety, concurrency, and performance. It's designed to help developers write efficient and reliable code, avoiding common pitfalls like memory leaks and data races. Rust is an excellent choice if you're looking for a modern language that offers low-level control, high performance, and built-in safety features.
Can Rust be used for web development?
Yes, Rust can be used for web development! While Rust is primarily known for its systems programming capabilities, its performance and safety features make it an appealing option for web development as well. There are several web frameworks available for Rust, such as Rocket and Actix, which allow you to build fast and secure web applications.
How does Rust ensure memory safety?
Rust ensures memory safety through its unique ownership system and the concept of borrowing. In Rust, each value has a single "owner," and when the owner goes out of scope, the value is automatically deallocated. Borrowing allows temporary access to a value without taking ownership. This system prevents common issues like double-free errors, dangling pointers, and data races, making it easier to write safe and efficient code.
What platforms and operating systems does Rust support?
Rust supports a wide range of platforms and operating systems, including Windows, macOS, Linux, and various Unix-like systems. It also has support for WebAssembly, allowing you to run Rust code in web browsers. Rust's extensive platform support makes it a versatile choice for developers working on different systems.
How can I get started with Rust?
To get started with Rust, you can visit the official Rust website (https://www.rust-lang.org/) and follow their installation guide. Once you have Rust installed, you can use "cargo," Rust's package manager and build tool, to create, build, and run your projects. There's also an extensive Rust book available online (https://doc.rust-lang.org/book/) that covers everything from the basics to advanced concepts, making it easy to learn the language at your own pace.