Custom Allocators in Rust
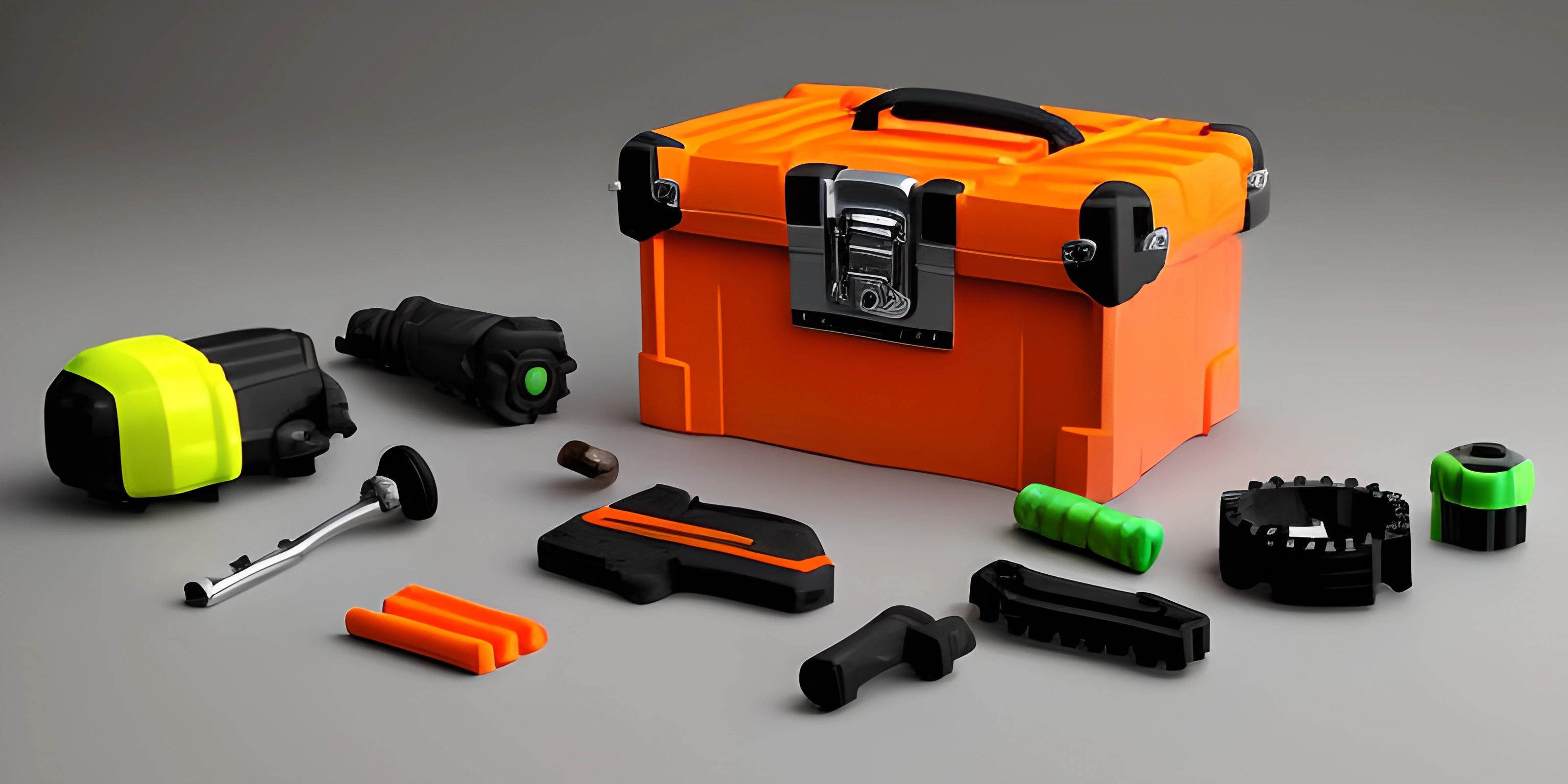
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Gaining control over memory allocation and deallocation is often a crucial step in writing high-performance or low-level code. In Rust, you can use custom allocators to achieve this level of control, allowing you to fine-tune your memory management strategy. Let's dive into the world of custom allocators and explore how they can help you optimize your Rust programs.
The Global Allocator
By default, Rust provides a global allocator that handles memory allocation and deallocation for you. This default allocator is designed to be efficient and general-purpose, but sometimes you may want to replace it with a custom one tailored to your specific needs.
To do this, you'll need to implement the std::alloc::GlobalAlloc
trait and mark your custom allocator with the #[global_allocator]
attribute. Here's a simple example of how to create a custom allocator in Rust:
use std::alloc::{GlobalAlloc, Layout, System}; struct MyCustomAllocator; unsafe impl GlobalAlloc for MyCustomAllocator { unsafe fn alloc(&self, layout: Layout) -> *mut u8 { System.alloc(layout) } unsafe fn dealloc(&self, ptr: *mut u8, layout: Layout) { System.dealloc(ptr, layout) } } #[global_allocator] static GLOBAL: MyCustomAllocator = MyCustomAllocator;
In this example, we've defined a MyCustomAllocator
struct and implemented the GlobalAlloc
trait for it. The alloc
and dealloc
methods delegate the actual allocation and deallocation to the default System
allocator. Lastly, we declare a static GLOBAL
variable with the #[global_allocator]
attribute to tell Rust to use our custom allocator instead of the default one.
Custom Allocator Use Cases
There are several reasons why you might want to use a custom allocator in Rust:
-
Performance Optimization: Custom allocators can be tailored to specific use cases or data structures, allowing for more efficient memory management.
-
Memory Tracking: Custom allocators can help track memory usage, leaks, and fragmentation, enabling better debugging and profiling of your application.
-
Low-level Control: Custom allocators can provide fine-grained control over memory allocation and deallocation, which is crucial in systems programming or embedded devices.
-
Memory Pooling: Custom allocators can implement memory pooling strategies that allocate a large block of memory upfront and then distribute smaller chunks as needed, reducing the number of calls to the system allocator.
Writing a Custom Allocator
When writing a custom allocator, you'll need to consider several aspects, such as thread safety, alignment, and error handling. Let's explore these concepts in more detail:
Thread Safety
Memory allocation and deallocation can potentially be called from multiple threads, so your custom allocator must be thread-safe. To ensure thread safety, you can use synchronization primitives like Mutex
or RwLock
to protect shared data, or design lock-free data structures using atomic operations.
Alignment
Memory alignment is an important aspect of memory management, as it can affect performance and compatibility with certain hardware. When implementing the alloc
method, you'll need to respect the Layout
's alignment requirements. The Layout
type provides the align()
and pad_to_align()
methods to help you achieve the proper alignment.
Error Handling
Handling allocation errors, such as running out of memory, is another crucial aspect of writing a custom allocator. The alloc
method should return a null pointer if the allocation fails, and the dealloc
method should handle null pointers gracefully. You can use the try_alloc
and try_dealloc
methods provided by the GlobalAlloc
trait to handle errors more ergonomically.
Conclusion
Custom allocators in Rust give you the power to fine-tune memory management in your programs. By understanding the basic concepts and use cases, you can create a custom allocator tailored to your specific needs and optimize your Rust applications for performance, control, and safety. Now go forth and conquer the world of memory management!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Web Frameworks (React) (psst, it's free!).
FAQ
What is a custom allocator in Rust?
A custom allocator in Rust is a user-defined memory allocator, designed to replace the default allocator provided by Rust. It allows you to gain more control over how memory is allocated and deallocated in your Rust programs, enabling you to optimize memory usage and performance according to your specific requirements.
How do I create a custom allocator in Rust?
To create a custom allocator in Rust, you need to implement the std::alloc::GlobalAlloc
trait and define your own allocation and deallocation functions. Here's a basic example in Rust:
use std::alloc::{GlobalAlloc, Layout, System}; use std::ptr::null_mut; struct MyCustomAllocator; unsafe impl GlobalAlloc for MyCustomAllocator { unsafe fn alloc(&self, layout: Layout) -> *mut u8 { // Your custom allocation logic here } unsafe fn dealloc(&self, ptr: *mut u8, layout: Layout) { // Your custom deallocation logic here } } #[global_allocator] static GLOBAL: MyCustomAllocator = MyCustomAllocator;
How do I use my custom allocator in a Rust program?
To use your custom allocator in a Rust program, you must define it as the global_allocator
using the #[global_allocator]
attribute. This will replace the default allocator with your custom allocator. Here's an example:
#[global_allocator] static GLOBAL: MyCustomAllocator = MyCustomAllocator;
Now, whenever you allocate memory in your Rust program, your custom allocator will be used instead of the default one.
What can I do with a custom allocator in Rust?
Custom allocators in Rust can help you:
- Optimize memory usage and performance, by tailoring the allocation strategy to your specific use case.
- Implement custom memory management schemes, like memory pooling or garbage collection.
- Track and analyze memory usage, allocation, and deallocation patterns in your application.
- Debug memory-related issues, such as leaks, buffer overflows, and use-after-free bugs.
Can I use multiple custom allocators in a single Rust program?
While it is possible to create multiple custom allocators in Rust, you can only define one as the global_allocator
. However, you can use different custom allocators within separate modules or libraries in your program. To do this, simply import the allocator you want to use and implement it within that specific module or library. Keep in mind that this approach won't replace the global allocator for the entire program.