Rust Ownership
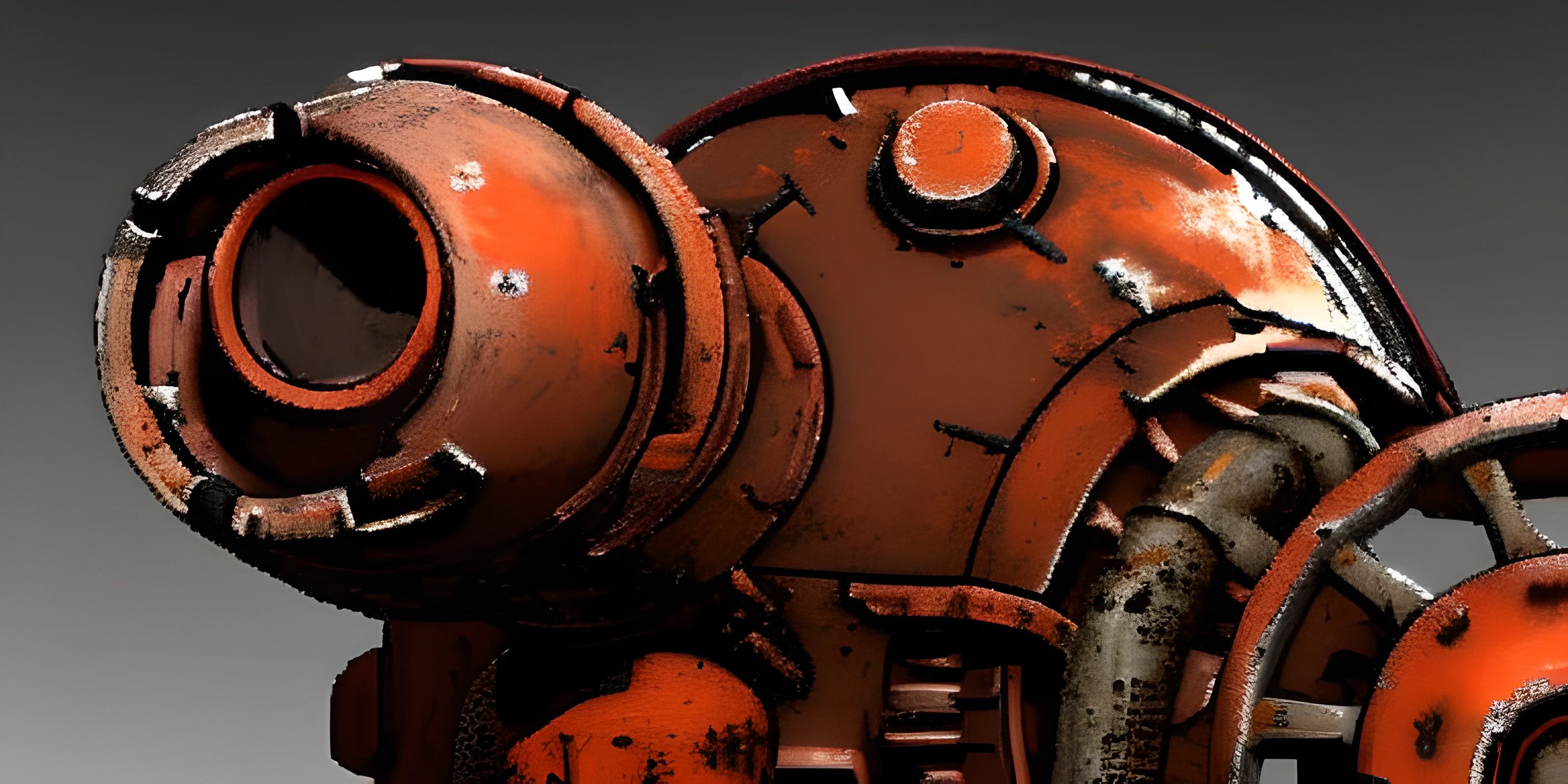
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the world of Rust, the word "ownership" carries a lot of weight. As a systems programming language, Rust strives for performance, safety, and concurrency. To achieve these goals, Rust's memory management employs a unique concept called ownership that enforces strict rules without the need for a garbage collector.
The Basics of Ownership
At the heart of Rust's ownership system are three key rules:
- Each value in Rust has a single variable called its owner.
- There can only be one owner at a time.
- When the owner goes out of scope, the value is dropped (i.e., the memory is freed).
These rules are enforced at compile time, which means there's no runtime overhead associated with them. Let's explore how these rules work in practice.
Ownership Example
Consider the following Rust code:
fn main() { let s1 = String::from("Hello, World!"); let s2 = s1; println!("{}", s1); }
This code won't compile. Why? The ownership of the String
value has been moved from s1
to s2
. Rust's ownership rules prevent us from using s1
after its ownership has been transferred to another variable. This helps eliminate issues like double frees and dangling pointers.
Borrowing and References
In many cases, we want to access a value without taking ownership of it. Rust allows us to do that using references and a concept called borrowing.
When you create a reference to a value, you're borrowing the value without taking ownership. There are two types of references in Rust: immutable and mutable. Immutable references allow read-only access, while mutable references permit read-write access.
Here's a simple example using immutable borrowing:
fn main() { let s1 = String::from("Hello, World!"); let s2 = &s1; println!("{}", s1); println!("{}", s2); }
This code compiles and runs just fine. We've borrowed s1
using an immutable reference, and the ownership remains with s1
.
Mutable Borrowing
If we want to modify a value while borrowing it, we need to use a mutable reference. Keep in mind that Rust enforces strict rules for mutable borrowing, such as:
- There can only be one mutable reference to a value in a particular scope.
- You cannot have both mutable and immutable references to the same value in the same scope.
The rules above ensure that your Rust programs are inherently safe from data races, a common issue in concurrent programming.
Here's an example of mutable borrowing:
fn main() { let mut s1 = String::from("Hello, World!"); let s2 = &mut s1; s2.push_str(" Rust is cool!"); println!("{}", s1); }
In this example, we've created a mutable reference to s1
and modified the value through the mutable reference. Keep in mind that we cannot use s1
while s2
is in scope because it's been mutably borrowed.
Conclusion
Rust's ownership, borrowing, and reference system might seem complex at first, but it provides a strong foundation for writing safe, concurrent, and high-performance code. By understanding and embracing these concepts, you'll find that writing efficient and reliable Rust programs becomes second nature. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Ownership and Borrowing (psst, it's free!).
FAQ
What is ownership in Rust?
Ownership is a core concept in Rust programming language that helps manage memory and prevent issues like data races and dangling references. In Rust, every value has a single variable that "owns" it. When the owner goes out of scope, the value is automatically deallocated, ensuring memory safety and efficient resource management.
How does Rust handle data when ownership is transferred between variables?
When the ownership of a value is transferred from one variable to another, Rust performs a "move" operation. In this process, the original variable loses access to the value and cannot be used after the transfer. This prevents unintentional data sharing and ensures that only the new owner can access and modify the value.
How can I share data between multiple parts of my program without transferring ownership?
Rust allows you to share data using references. You can create a reference to a value using the &
operator, which creates a read-only (immutable) reference. If you need to create a mutable reference, you can use the &mut
operator. However, Rust enforces certain rules, known as "borrowing rules," to ensure safety while using references:
- At any given time, either there can be multiple read-only references or one mutable reference, but not both.
- References must always be valid; they cannot outlive the value they're pointing to.
What is a "slice" in Rust, and how is it related to ownership?
Slices are a useful feature in Rust that allows you to access a contiguous sequence of elements in a collection, like an array or a string, without transferring the ownership of the entire collection. A slice is essentially a reference to a part of the collection, and it follows the borrowing rules like any other reference. To create a slice, you can use the syntax [start..end]
, where start
is the index of the first element and end
is the index after the last element you want to include.
How does Rust ownership help in preventing data races in concurrent programs?
Rust ownership, along with borrowing rules, ensures safe concurrency by preventing data races. A data race occurs when two or more threads access shared data simultaneously, and at least one of them modifies the data. Since Rust's ownership system allows either multiple immutable references or a single mutable reference, but not both at the same time, it guarantees that either the data is read-only or only one thread can modify it, effectively preventing data races.