Rust Traits vs Interfaces
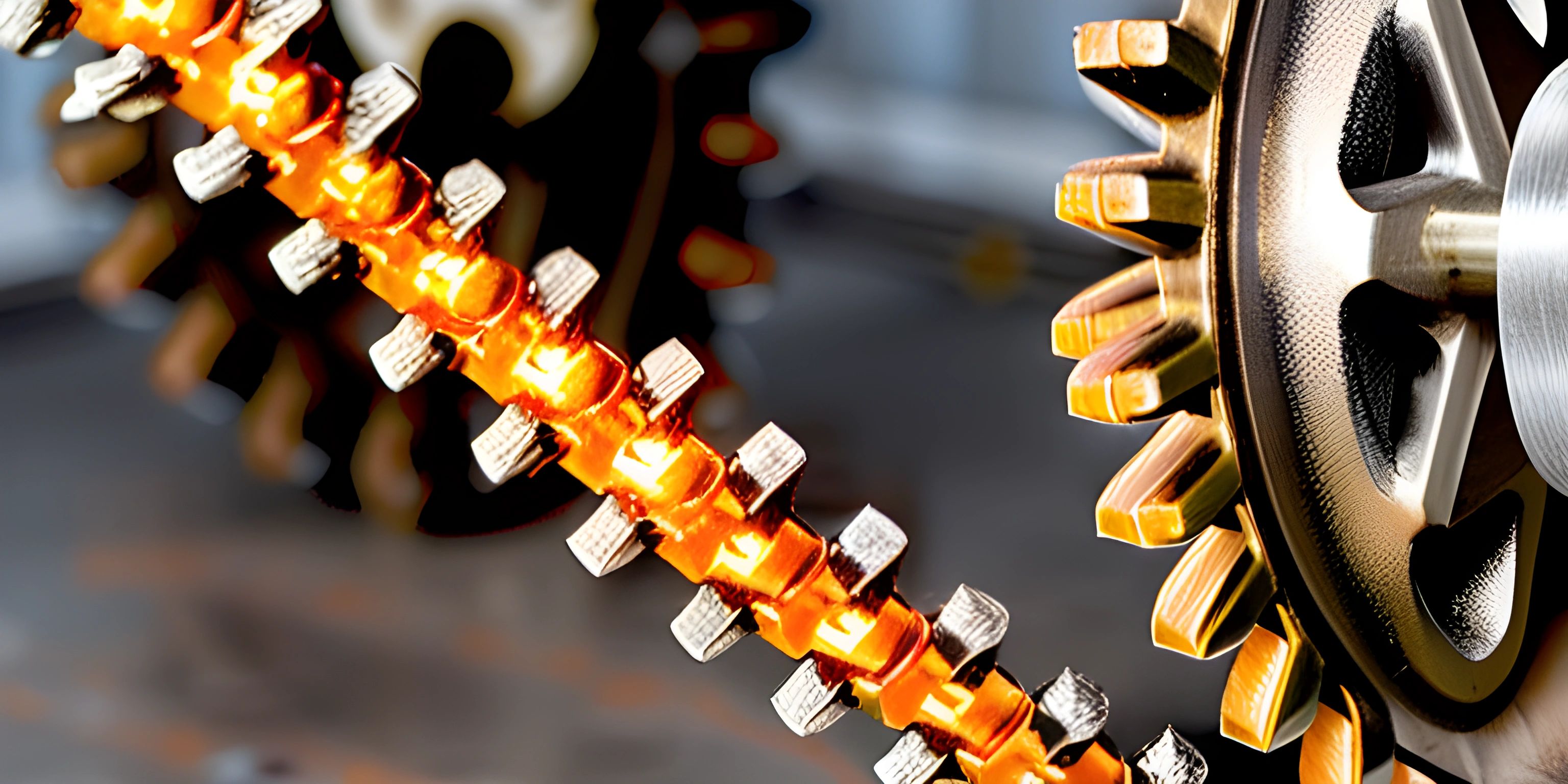
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Rust is becoming more and more popular as a systems programming language, thanks to its focus on safety and performance. One of the key features of Rust is traits, which are used to define shared behavior across different types. You might have heard about interfaces in other languages, like Java or TypeScript, which serve a similar purpose. In this article, we'll be diving into the similarities and differences between Rust traits and interfaces in other languages.
Traits in Rust
Traits in Rust are a way to define shared behavior between different types. They can be thought of as a set of methods that can be implemented for any data type. Traits are a powerful feature of Rust that allows for abstraction and code reuse. Let's see an example of a trait in Rust:
trait Speak { fn speak(&self); } struct Dog; struct Cat; impl Speak for Dog { fn speak(&self) { println!("Woof!"); } } impl Speak for Cat { fn speak(&self) { println!("Meow!"); } } fn main() { let dog = Dog; let cat = Cat; dog.speak(); cat.speak(); }
In this example, we defined a Speak
trait with a single method speak
. We then implemented the Speak
trait for two different structs, Dog
and Cat
. When we call the speak
method on both Dog
and Cat
instances, we get the appropriate output.
Interfaces in Other Languages
Interfaces in other programming languages, like Java and TypeScript, are a way to define a contract for implementing classes or objects. They describe the structure and behavior that the implementing classes or objects must adhere to. Here's an example of an interface in Java:
interface Speakable { void speak(); } class Dog implements Speakable { @Override public void speak() { System.out.println("Woof!"); } } class Cat implements Speakable { @Override public void speak() { System.out.println("Meow!"); } }
And an example in TypeScript:
interface Speakable { speak(): void; } class Dog implements Speakable { speak(): void { console.log("Woof!"); } } class Cat implements Speakable { speak(): void { console.log("Meow!"); } }
In both cases, we defined an interface Speakable
with a single method speak
. Then we created two classes, Dog
and Cat
, that implement the Speakable
interface.
Comparing Traits and Interfaces
Now that we've seen examples of traits and interfaces, let's explore their similarities and differences:
Similarities
- Both traits and interfaces define a contract for implementing types, specifying a set of methods that must be implemented.
- They promote code reuse by allowing multiple types to share the same behavior.
- Both can be used for abstraction, making it possible to write generic code that works with different types implementing a trait or an interface.
Differences
- Rust traits can have default method implementations, while interfaces in many languages (e.g., Java prior to version 8) cannot. This allows for more flexible code reuse and evolution in Rust.
- Traits in Rust can also have associated types and constants, whereas interfaces typically only define methods.
- Rust traits use the
impl
keyword for implementing a trait for a type, while interfaces use theimplements
keyword (or similar) in other languages.
Conclusion
While Rust traits and interfaces in other languages share some similarities in terms of defining shared behavior and promoting code reuse, they also have their differences. Traits in Rust offer more flexibility with default method implementations, associated types, and constants, making them a powerful feature in the Rust programming language.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What are Rust traits?
Traits in Rust are a way to define shared behavior between different types. They can be thought of as a set of methods that can be implemented for any data type. Traits are a powerful feature of Rust that allows for abstraction and code reuse.
How are Rust traits similar to interfaces in other languages?
Both Rust traits and interfaces in other languages define a contract for implementing types, specifying a set of methods that must be implemented. They both promote code reuse and abstraction by allowing multiple types to share the same behavior.
What are some differences between Rust traits and interfaces?
Differences between Rust traits and interfaces include: Rust traits can have default method implementations, associated types, and constants, while interfaces typically only define methods; Rust traits use the impl
keyword for implementing a trait for a type, while interfaces use the implements
keyword (or similar) in other languages.