Error Handling in Compiler Design
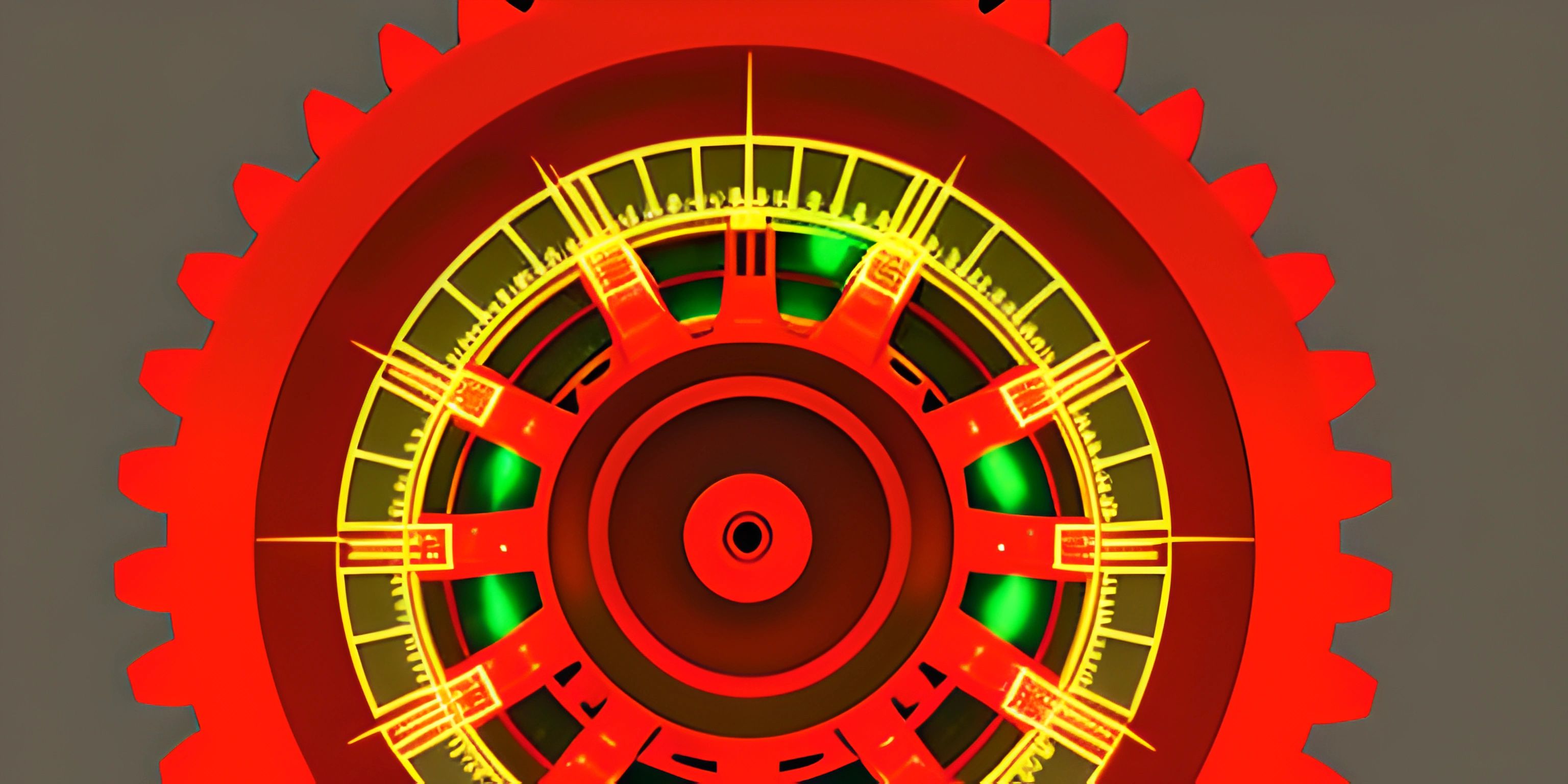
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Error handling is a crucial aspect of compiler design. Just like humans make mistakes while typing, programmers can write incorrect or incomplete code. It's the compiler's job to catch these errors and inform the programmer, so they can fix the problem. We'll discuss the different types of errors, how to detect them, and strategies for error reporting and recovery.
Types of Errors
Errors in compiler design can be broadly categorized into three types: lexical, syntax, and semantic.
Lexical Errors
Lexical errors occur when the compiler can't recognize a sequence of characters as a valid token. For example, if a programmer accidentally types 5+*3
, the *
symbol doesn't correspond to any valid token in the programming language. To handle this error, the compiler identifies the problematic character(s) and reports it to the programmer.
Syntax Errors
Syntax errors involve violations of the programming language's grammar rules. For instance, if a programmer forgets a closing parenthesis in an expression like 3 * (5 + 2
, the compiler can't parse the code correctly. A common approach to handling syntax errors is by using parsing algorithms that can detect and report these issues.
Semantic Errors
Semantic errors happen when the code is syntactically correct but doesn't make sense logically. For example, trying to divide a number by a string or using a variable that was never declared. Semantic errors are more difficult to handle, and they often require advanced static analysis techniques.
Error Reporting
Effective error reporting helps programmers quickly locate and fix problems in their code. A good error message should:
- Clearly identify the error type (lexical, syntax, or semantic)
- Specify the location (line number and character position) of the error in the code
- Provide a helpful description of the issue and possible suggestions to fix it
Many compilers also provide integrated development environments (IDEs) with features like code navigation and real-time error checking, making it easier for programmers to handle errors efficiently.
Error Recovery
Error recovery is the process of allowing the compiler to continue processing the code after encountering an error. This is important because it enables the compiler to report multiple errors in a single run, rather than stopping at the first issue.
A common error recovery strategy is the panic mode. When the compiler encounters an error, it enters panic mode and skips characters or tokens until it finds a specific synchronization point (e.g., a semicolon or closing brace). The compiler then resumes normal parsing from that point, potentially reporting more errors if found.
Another approach is the phrase-level recovery, which involves replacing, deleting, or inserting tokens to create a syntactically correct version of the code. However, this method can introduce new errors or mask existing ones, so it requires careful implementation.
In summary, handling errors in compiler design is essential for user-friendly development environments. By detecting and reporting lexical, syntax, and semantic errors, as well as implementing error recovery strategies, compilers can help programmers write better, more reliable code.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Chat App Backend (psst, it's free!).