Thread Safety in Rust
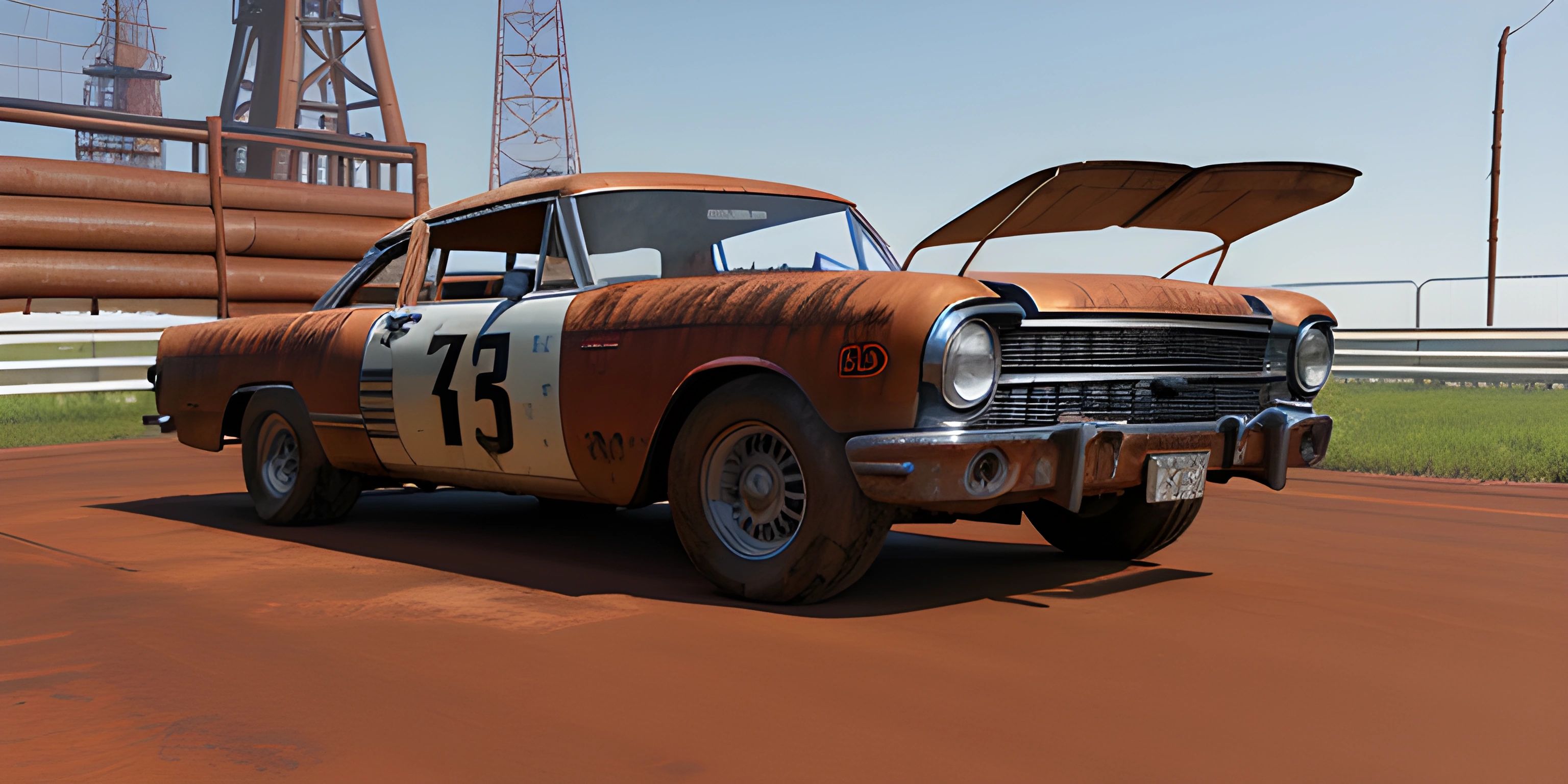
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
To scale new heights in programming, it's essential to have a grip on concurrency, which is like teaching your program to juggle several tasks at once. But with great power comes great responsibility, and that's where the concept of thread safety comes into play. Thread safety in Rust is like a superhero that saves your program from the evil villain known as 'data race'. So put on your capes, and let's dive into the world of thread safety in Rust!
Concurrency in Rust
Before we kick start our journey, let's understand what concurrency is all about. In Rust, concurrency is the execution of independent tasks in a program at the same time, making your program faster and more efficient. It's like having multiple chefs in a kitchen, each preparing a different dish simultaneously. But what if two chefs need the same knife at the same time? Chaos ensues, right? This is where thread safety comes to the rescue!
Mutex and Locks
Imagine a chef's knife is a shared resource in your program. To prevent two chefs (or threads) from using the knife at the same time, Rust introduces the concept of Mutex and Locks. Mutex stands for 'mutual exclusion', like the principle "My turn, your wait". It's a protocol that ensures only one thread can access a shared resource at a time. The thread locks the Mutex before using the resource and unlocks it after using it, keeping your program safe from unexpected results.
use std::sync::Mutex; let mutex = Mutex::new(0); // shared resource { let mut num = mutex.lock().unwrap(); // locking the mutex *num = 2; } // unlocking the mutex automatically when it goes out of scope
This code creates a Mutex around an integer. The mutex is locked with .lock()
, and then the value can be modified. The mutex is automatically unlocked when num
goes out of scope.
Atomic Operations
But what if you're juggling with tasks that are so fast, mutexes slow things down? Atomic operations come to the rescue! They are operations that run in a single step, without being interrupted. These operations are like the Flash of your program, they're so quick, they can complete their task before another thread even gets a chance to interfere!
Here's how you can perform an atomic operation in Rust:
use std::sync::atomic::{AtomicUsize, Ordering}; let atomic = AtomicUsize::new(0); atomic.store(2, Ordering::SeqCst);
This Rust code creates an atomic integer and stores a value in it. The Ordering::SeqCst
parameter ensures that operations happen in the order you expect, preventing surprises in your code.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is thread safety in Rust?
Thread safety in Rust is a way to ensure that concurrent threads of execution play nice with each other. Rust provides several tools to achieve thread safety, like Mutexes, Locks, and Atomic operations. These tools help prevent data races in your program, ensuring reliable and predictable performance.
How does a Mutex work in Rust?
In Rust, a Mutex (Mutual Exclusion) is a protocol that allows only one thread to access a shared resource at a time. A thread locks the Mutex before accessing the resource and unlocks it after using it. This ensures that other threads can't interfere with the resource while it's being used.
What are atomic operations in Rust?
Atomic operations in Rust are operations that complete in a single, uninterrupted step. They're like the Flash of your program, completing their task so quickly that other threads don't get a chance to interfere. This is especially useful for very fast operations, where using a Mutex might slow things down.