Understanding Threads in Programming
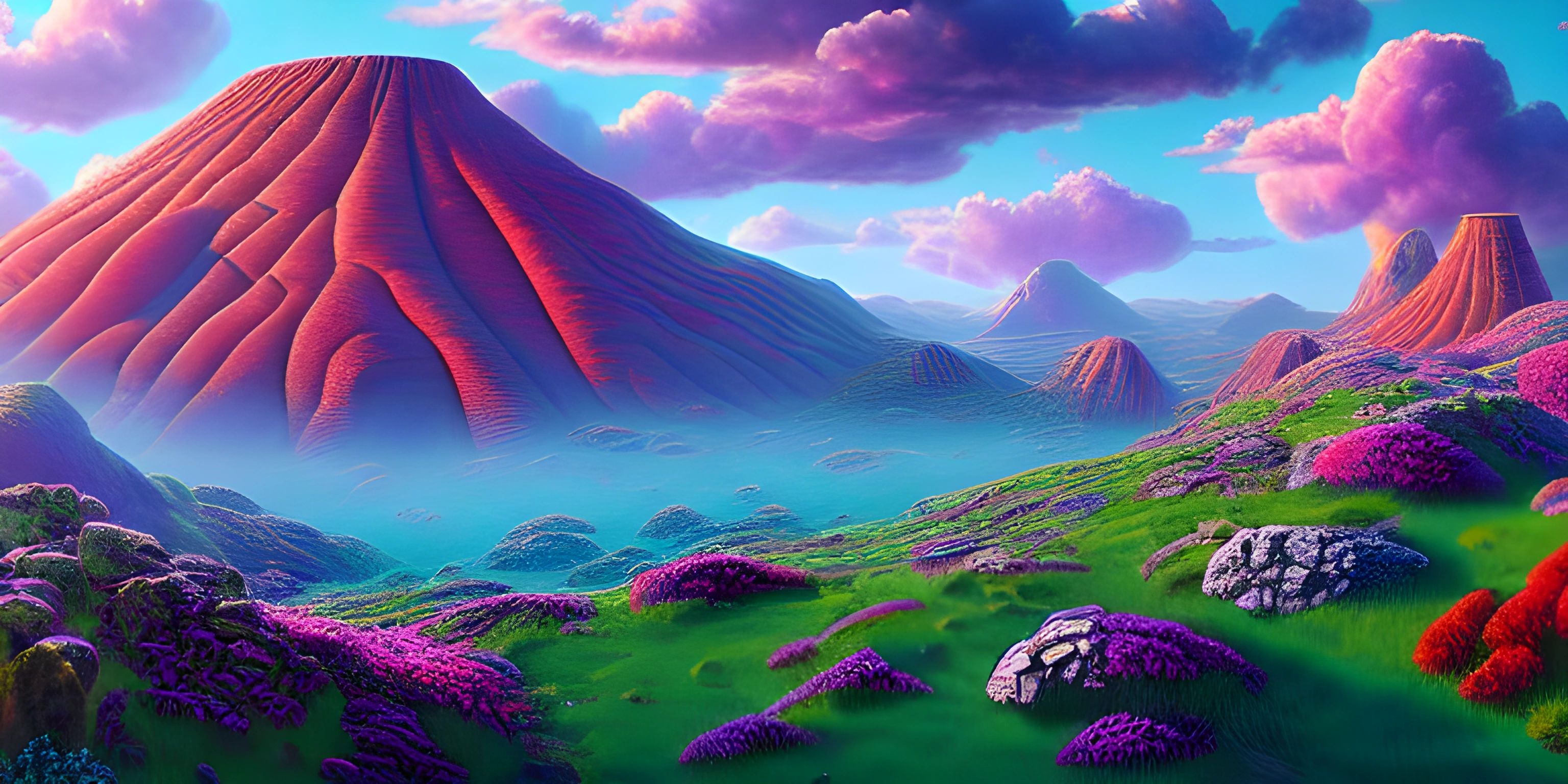
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ever wondered how your computer juggles multiple tasks at once? It's like having a circus troupe where each performer is a thread, and the ringmaster is your CPU. Let's delve into the world of threads and see how they bring multitasking magic to life.
What is a Thread?
Imagine a thread as a single path of execution within a program. When you run a program, it usually starts with a single thread, the main thread. However, for more complex operations, like handling user inputs while processing data, you need multiple threads running concurrently.
Threads are like the performers in our circus analogy. Each performer (thread) can execute tasks independently but simultaneously. This concurrent execution is what gives us the ability to multitask efficiently.
Why Use Threads?
Threads are essential for improving the performance of applications, especially in scenarios that require concurrent or parallel execution. Here are some real-world analogies:
- Cooking Dinner: Imagine you're cooking dinner and you need to boil water while chopping vegetables. If you waited for the water to boil before chopping, dinner would take forever. Instead, you do both tasks simultaneously, just like threads can handle multiple tasks at once.
- Browsing the Web: When you're browsing the web, multiple threads allow for loading images, fetching data, and rendering the page all at the same time. Without threads, you’d have to wait for one task to finish before starting another.
How Threads Work
Let's look at how threads are created and managed. Different programming languages have different ways to handle threads. We'll explore some examples in Python and Java.
Python Example
Python provides a threading module to work with threads. Here's a simple example:
import threading def print_numbers(): for i in range(5): print(f"Number: {i}") # Create a thread thread = threading.Thread(target=print_numbers) # Start the thread thread.start() # Wait for the thread to finish thread.join() print("Thread has finished execution.")
In this example, we've created a thread to run the print_numbers
function. The thread.start()
method begins the execution, and thread.join()
waits for the thread to finish before proceeding.
Java Example
Java uses the Thread
class and the Runnable
interface for threading. Here's how you can create and start a thread:
class PrintNumbers implements Runnable { public void run() { for (int i = 0; i < 5; i++) { System.out.println("Number: " + i); } } } public class Main { public static void main(String[] args) { Thread thread = new Thread(new PrintNumbers()); thread.start(); try { thread.join(); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("Thread has finished execution."); } }
Here, we implement the Runnable
interface in the PrintNumbers
class and override the run
method. In the Main
class, we create a new thread and start it.
Thread Management
Managing threads involves more than just starting and stopping them. It includes synchronization, preventing race conditions, and ensuring thread safety. These are crucial for making sure threads don't step on each other's toes.
Synchronization
Synchronization is like setting up a traffic signal at a busy intersection. It ensures that threads coordinate access to shared resources. In Java, the synchronized
keyword can be used:
class Counter { private int count = 0; public synchronized void increment() { count++; } public int getCount() { return count; } } class Main { public static void main(String[] args) { Counter counter = new Counter(); Thread t1 = new Thread(() -> { for (int i = 0; i < 1000; i++) { counter.increment(); } }); Thread t2 = new Thread(() -> { for (int i = 0; i < 1000; i++) { counter.increment(); } }); t1.start(); t2.start(); try { t1.join(); t2.join(); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("Final count: " + counter.getCount()); } }
In this example, the synchronized
keyword ensures that only one thread can execute the increment
method at a time, preventing race conditions.
Thread Safety
Thread safety ensures that your code runs correctly when accessed by multiple threads simultaneously. Strategies for achieving thread safety include using locks, atomic variables, and concurrent data structures.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is the difference between a thread and a process?
A process is an independent program running in its own memory space, while a thread is a path of execution within a process. Threads within the same process share the same memory space, allowing for more efficient communication and resource sharing.
Can threads improve performance on single-core processors?
Yes, threads can improve performance on single-core processors through better utilization of CPU cycles by allowing I/O-bound tasks to proceed while CPU-bound tasks are waiting for input/output operations.
What are some common issues with multithreading?
Common issues include race conditions, deadlocks, and thread starvation. These can lead to unpredictable behavior, performance degradation, and bugs that are hard to reproduce and fix.
How do you debug multithreaded applications?
Debugging multithreaded applications can be challenging. Tools like thread analyzers, profilers, and debuggers that support thread inspection can help. Logging and tracing can also be useful for understanding thread interactions.
Are there alternatives to threading for concurrency?
Yes, alternatives include using asynchronous programming models (like async/await in JavaScript), parallel processing (using multiple processes), and reactive programming frameworks that handle concurrency in a different way.