Stack Data Structure
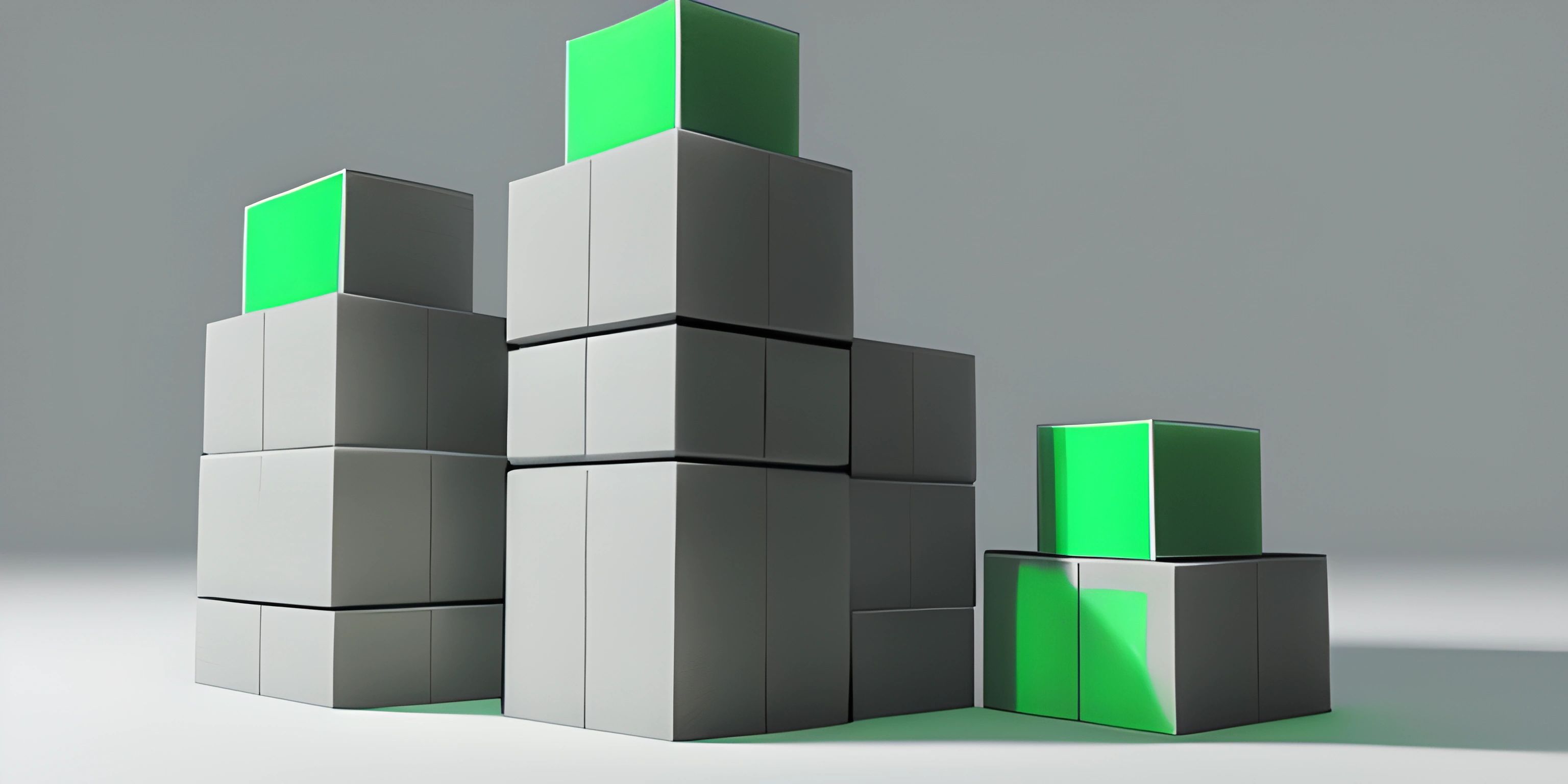
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine you have a pile of books that you can only add or remove from the top. That's exactly how a stack data structure works! A stack is a collection of elements with two main operations: push (add an element to the top) and pop (remove the top element). But what makes it so special, and where is it used? Let's dive in.
Last In, First Out (LIFO)
The stack data structure follows the Last In, First Out (LIFO) principle, meaning that the most recently added element is always the first one to be removed. In the case of our book pile, the book placed on top will be the first one taken off.
Basic Operations
Stacks typically have two primary operations, along with some additional utility methods. Here's a brief overview:
- Push: Add an element to the top of the stack.
- Pop: Remove and return the top element from the stack.
- Peek (optional): Return the top element without removing it.
- IsEmpty (optional): Check if the stack is empty.
Use Cases
Now that we know how a stack works, let's explore some of the scenarios where it proves to be extremely useful:
Balanced Parentheses
A common use case for stacks is checking whether a given expression has balanced parentheses, brackets, or braces. For example, the expression "{[()]}[]"
is balanced, while "{[()]"
is not. Using a stack, we can iterate through the expression and "push" each opening symbol onto the stack. When we encounter a closing symbol, we "pop" the stack and check if it matches the corresponding opening symbol. If not, the expression is unbalanced.
Function Calls and Recursion
When working with functions, each time a function is called, its execution context (including local variables and return address) is stored on the call stack. This allows the program to know where to continue executing once the function returns. In the case of recursion, the call stack can help keep track of multiple nested function calls.
Undo and Redo Actions
Imagine you're using a text editor, and you'd like to undo or redo your actions. Stacks can be used to implement this functionality by storing each action in two separate stacks: one for undoing and one for redoing. Whenever an action is performed, it's pushed onto the undo stack. When the undo button is pressed, the action is popped from the undo stack and pushed onto the redo stack. Similarly, pressing the redo button pops the action from the redo stack and pushes it back onto the undo stack.
Implementing a Stack
Stacks can be implemented using arrays, linked lists, or other data structures, depending on the requirements and the programming language in use. Many languages also provide built-in stack implementations or libraries that make it easy to work with stacks.
Now you have a solid understanding of the stack data structure and its use cases. Next time you're faced with a programming problem, consider whether a stack might be the right tool for the job. Happy stacking!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is a stack data structure?
A stack data structure is a linear data structure that follows the Last In First Out (LIFO) principle, meaning that the most recently added element is the first one to be removed. It is similar to a real-life stack of plates, where you can only add or remove plates from the top of the stack.
What are the primary operations performed on a stack?
The primary operations performed on a stack are:
- Push: Adds an element to the top of the stack.
- Pop: Removes and returns the top element from the stack.
- Peek/Top: Returns the top element without removing it from the stack.
- isEmpty: Checks if the stack is empty or not.
Can you provide a code example of a simple stack implementation?
Here's a basic Python example of a stack implementation using a list:
class Stack: def __init__(self): self.items = [] def push(self, item): self.items.append(item) def pop(self): if not self.isEmpty(): return self.items.pop() def peek(self): if not self.isEmpty(): return self.items[-1] def isEmpty(self): return len(self.items) == 0 # Usage example stack = Stack() stack.push(1) stack.push(2) stack.push(3) print(stack.pop()) # Output: 3 print(stack.peek()) # Output: 2 print(stack.isEmpty()) # Output: False
What are some common use cases for stacks?
Stacks have a wide range of use cases, including:
- Evaluating expressions and performing calculations in programming languages.
- Managing the order of function calls and execution in programming languages.
- Implementing undo/redo functionality in applications.
- Navigating back and forth in web browsers.
- Parsing and validating syntax in compilers and interpreters.
What is the time complexity of stack operations?
The time complexity of primary stack operations (push, pop, peek, and isEmpty) is O(1) since these operations involve constant time access to the top element of the stack.