Trie Data Structure
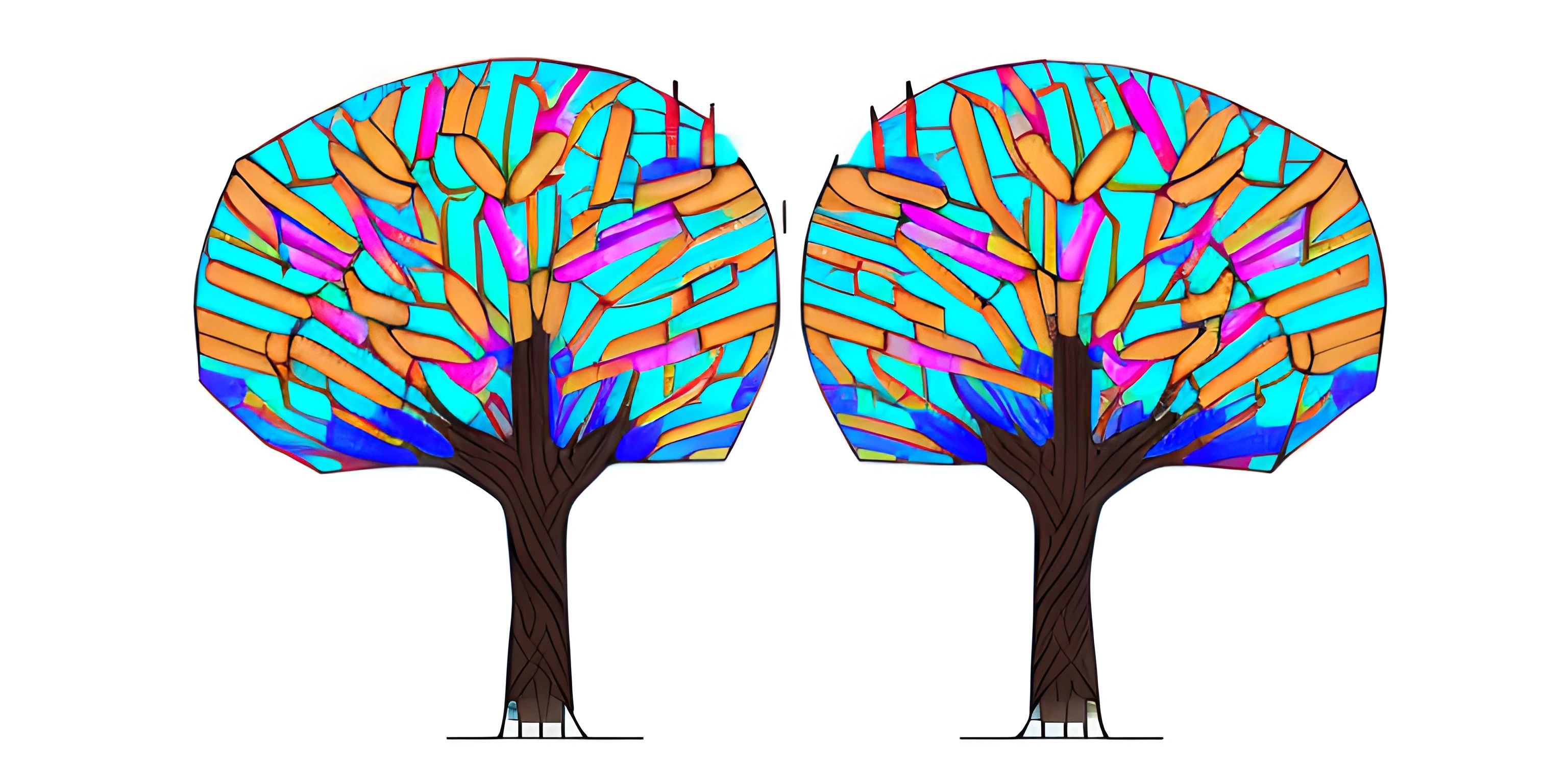
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Tries, also known as prefix trees, are a fascinating data structure that's perfect for certain text processing tasks. They might not be the star of the show in every application, but when it comes to organizing and searching for strings, they're a life-saver.
What is a Trie?
A trie is a tree-like data structure that stores a dynamic set of strings. It's particularly useful for scenarios involving a large dataset of strings and prefix-based searches. The name "trie" is derived from the word "retrieval" – as in retrieving information from a collection.
In a trie, each node represents a character, and nodes along a path form a string. Strings are stored in such a way that all descendants of a node share a common prefix associated with that node. This clever organization makes it easy to search for strings based on their prefixes.
Here's a visual example of a trie containing the words "to", "tea", "ted", "ten", and "inn":
(root) / \ t i / \ \ o e n / \ \ a d n / \ t e
Applications of Tries
Tries have an impressive range of applications in text processing. Some of the most common ones include:
-
Auto-complete: When you start typing in a search engine, it suggests possible queries based on the prefix you've entered. To accomplish this, search engines use tries to quickly find matching terms.
-
Spell checking: Tries can be used to check whether a word is spelled correctly by searching for it in a trie containing a dictionary of valid words.
-
IP routing: In networking, tries can be used to efficiently store and search for IP addresses and their routing information.
-
String matching: Tries can help identify strings that share a common prefix or find the longest common prefix between multiple strings.
Now that we've seen what tries are capable of, let's dive deeper and explore how to create and manipulate them.
Creating a Trie
To create a trie, we first need to define the structure for the nodes. Each node will have a character value and a dictionary to store its children. Additionally, we'll include a boolean flag to indicate whether a node represents the end of a word.
Here's a simple Python implementation of a trie node:
class TrieNode: def __init__(self, char): self.char = char self.children = {} self.is_word_end = False
To insert a string into the trie, we start at the root node and traverse the trie, character by character. If a character isn't found in the current node's children, we create a new node for it and add it to the children dictionary. Finally, we set the is_word_end
flag for the last character in the string.
class Trie: def __init__(self): self.root = TrieNode(None) def insert(self, word): node = self.root for char in word: if char not in node.children: node.children[char] = TrieNode(char) node = node.children[char] node.is_word_end = True
Searching in a Trie
Searching for a string in a trie is pretty straightforward. Starting at the root node, we follow the path of characters in the trie. If we reach a dead end or the trie doesn't contain the string, we return False
. If we reach the end of the input string and the is_word_end
flag is set, we return True
.
def search(self, word): node = self.root for char in word: if char not in node.children: return False node = node.children[char] return node.is_word_end
Tries might not always be the first data structure that comes to mind, but they're incredibly powerful when applied to the right problems. With their ability to efficiently store and search strings, tries are a valuable tool in any programmer's toolkit.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Reading Data Files (psst, it's free!).