Understanding Two-Pass Assemblers
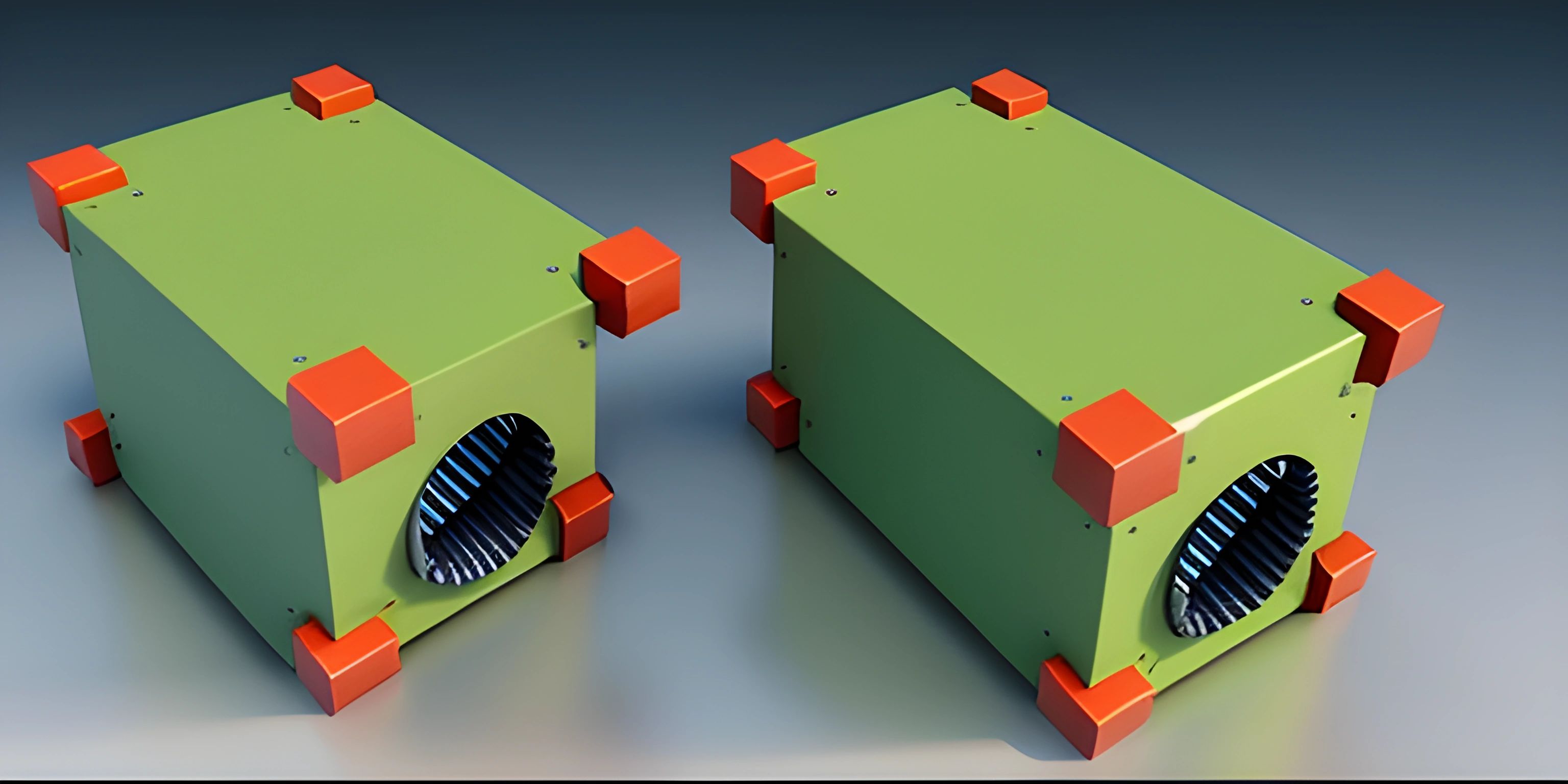
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine you're building a complex Lego set. You first sort all the pieces, ensuring you have everything, then go through the instructions to assemble the model. This is similar to how a two-pass assembler works in systems programming. Let's dive into the nitty-gritty of two-pass assemblers and understand why they are a critical component in the world of low-level programming.
What is an Assembler?
An assembler is a program that converts assembly language, a low-level human-readable language, into machine code, which is directly executable by a computer's CPU. Assembly language uses mnemonics, short codes that represent machine-level instructions, and allows programmers to write code that closely interacts with the hardware.
The Two-Pass Assembler
A two-pass assembler processes the assembly code in two separate phases or "passes." This method ensures that all symbols and addresses are correctly resolved before generating the final machine code.
First Pass: Symbol Table Creation
In the first pass, the assembler goes through the code to create a symbol table. A symbol table is like a dictionary that maps labels and variables to their respective memory addresses. Here's an example in a simplified assembly language:
START: LDA VALUE ; Load the value into the accumulator ADD TEN ; Add 10 to the accumulator STA RESULT ; Store the result VALUE: .DATA 5 ; Define VALUE as 5 TEN: .DATA 10 ; Define TEN as 10 RESULT: .DATA 0 ; Reserve space for the result
During the first pass, the assembler identifies all labels (START
, VALUE
, TEN
, and RESULT
) and their corresponding addresses. No machine code is generated in this pass.
Second Pass: Code Generation
In the second pass, the assembler uses the symbol table to resolve addresses and generate the final machine code. Here's the machine code for the example above:
0001: LDA 0005 ; Load the value at address 0005 0002: ADD 0006 ; Add the value at address 0006 0003: STA 0007 ; Store the result at address 0007 0005: .DATA 5 ; Value 5 at address 0005 0006: .DATA 10 ; Value 10 at address 0006 0007: .DATA 0 ; Reserve space for the result at address 0007
Advantages of Two-Pass Assemblers
Symbol Resolution
One of the biggest advantages of two-pass assemblers is their ability to resolve forward references. A forward reference occurs when a symbol is used before it is defined. For example:
JMP END START: LDA VALUE END: STA RESULT
In this code, END
is used before it is defined. A one-pass assembler would struggle with this, but a two-pass assembler handles it gracefully.
Error Detection
Two-pass assemblers also provide better error detection and reporting. Since the assembler goes through the code twice, it can catch undefined symbols, duplicate labels, and other errors more effectively.
Optimization Opportunities
Because the two-pass assembler has a complete view of the code during the second pass, it can perform optimizations that a one-pass assembler cannot. For example, it might replace certain instructions with more efficient ones or optimize memory usage.
Disadvantages of Two-Pass Assemblers
Performance Overhead
The primary disadvantage of two-pass assemblers is the performance overhead. Since the assembler processes the code twice, it takes more time to assemble the code compared to a one-pass assembler. However, this overhead is often justified by the improved accuracy and optimization.
Increased Complexity
Two-pass assemblers are inherently more complex than their one-pass counterparts. This complexity can make the assembler more difficult to implement and maintain.
Memory Usage
Two-pass assemblers require more memory to store the intermediate symbol table and other data structures. In systems with limited resources, this can be a significant drawback.
Real-World Applications
Two-pass assemblers are commonly used in systems programming, where precise control over hardware is essential. They are also used in embedded systems, where optimization and efficient memory usage are critical. For example, the Linux kernel and other operating systems often rely on two-pass assemblers for parts of their code.
Conclusion
Two-pass assemblers strike a balance between accuracy, optimization, and complexity. They are a powerful tool in the arsenal of systems programmers, allowing for precise control over hardware while ensuring that all symbols and addresses are correctly resolved.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).
FAQ
What is a symbol table in a two-pass assembler?
A symbol table is a data structure used by the assembler to map labels and variables to their respective memory addresses. It is created during the first pass and used in the second pass to resolve addresses and generate the final machine code.
How does a two-pass assembler handle forward references?
In the first pass, the assembler creates a symbol table without generating machine code. This allows it to record the addresses of all labels and variables. In the second pass, it uses this symbol table to resolve forward references and generate the final machine code.
What are the main advantages of using a two-pass assembler?
The main advantages include accurate symbol resolution, better error detection and reporting, and the opportunity for code optimization. These benefits often outweigh the performance overhead and increased complexity.
Why are two-pass assemblers used in systems programming?
Two-pass assemblers provide precise control over hardware, which is essential in systems programming. They ensure accurate symbol resolution and allow for optimizations that improve the efficiency and performance of the code.
What is the primary disadvantage of a two-pass assembler?
The primary disadvantage is the performance overhead, as the assembler processes the code twice. This can make the assembly process slower compared to a one-pass assembler.