x86 NASM Assembly Introduction
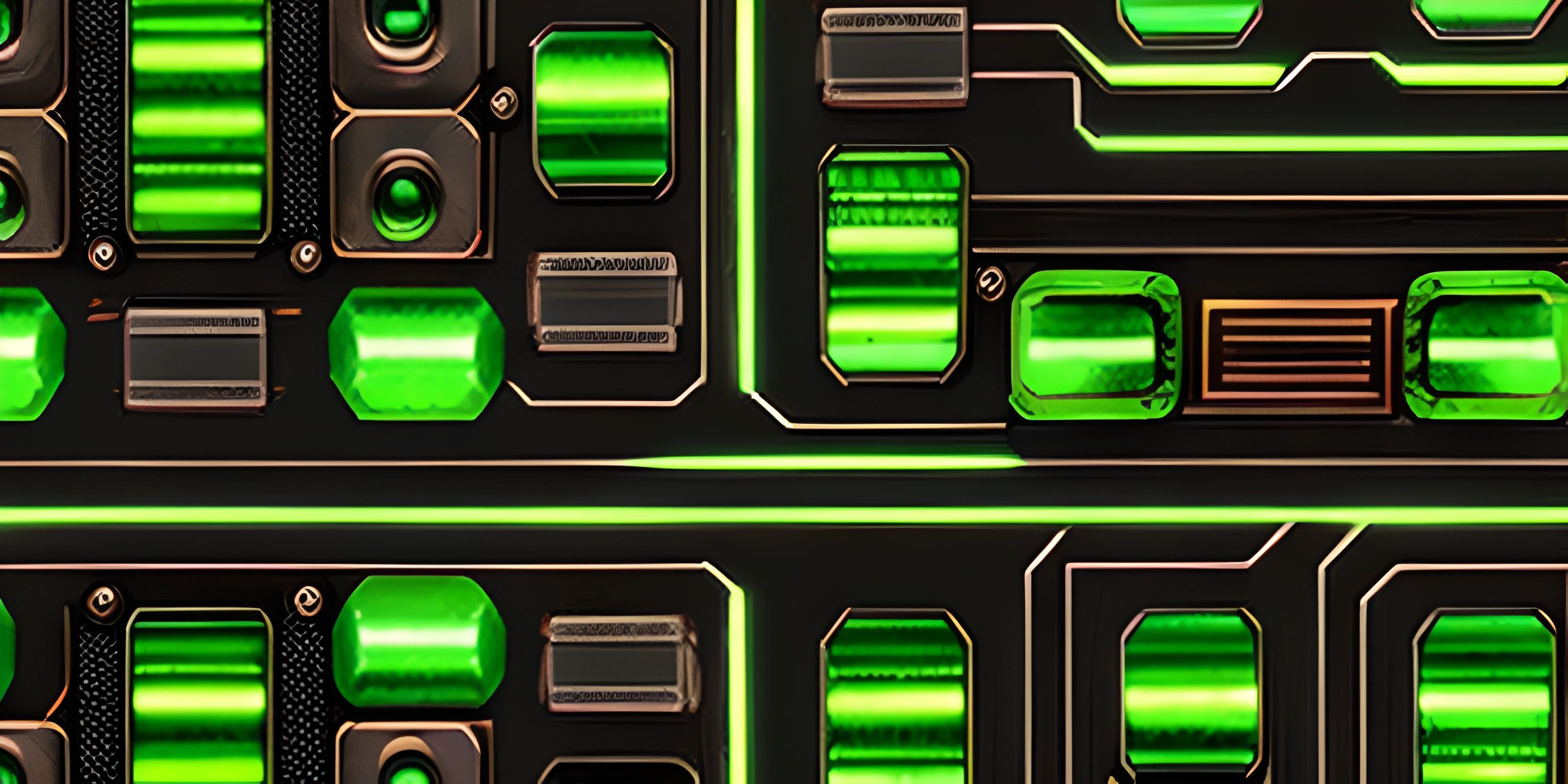
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the world of programming, there are various languages to choose from, and x86 assembly language is one of them. It allows you to communicate with your computer at a low level, using mnemonics to represent machine-level instructions. In this primer, you'll be introduced to x86 assembly language and the NASM assembler.
x86 Assembly Language
x86 assembly language is a family of low-level programming languages that are tied to the x86 instruction set architecture (ISA). This means that programs written in x86 assembly language are closely related to the underlying hardware, making them particularly useful for tasks that require fine-grained control over the computer's operation.
Registers
In x86 assembly, there are a number of registers that hold data. These are like small storage spaces directly inside the CPU, allowing for quick access to the data they contain. Some common registers include the EAX, EBX, ECX, and EDX registers, which are general-purpose registers used for various tasks, and the EIP (instruction pointer) register, which tells the CPU which instruction to execute next.
Instructions
Instructions in x86 assembly language are the building blocks of your program. Each instruction corresponds to a specific operation that the CPU can perform. Some examples of x86 instructions include:
MOV
: Move data between registers or memory locationsADD
: Add two values togetherSUB
: Subtract one value from anotherJMP
: Jump to a specific location in the code
NASM Assembler
The Netwide Assembler (NASM) is an assembler and disassembler for the x86 architecture. It can be used to create programs written in x86 assembly language by converting your assembly code into machine code that can be executed by the CPU. NASM has a simple syntax and supports a wide range of output formats, making it a popular choice for x86 assembly development.
Installation
To get started with NASM, you'll need to install it on your computer. You can download the latest version from the official website and follow the installation instructions for your operating system.
Writing a Simple Program
Once you have NASM installed, you can write a simple x86 assembly program. Here's an example that prints "Hello, World!" using the sys_write
and sys_exit
system calls:
section .data hello db 'Hello, World!', 0xA helloLen equ $ - hello section .text global _start _start: ; write "Hello, World!" to stdout mov eax, 4 mov ebx, 1 lea ecx, [hello] mov edx, helloLen int 0x80 ; exit the program mov eax, 1 xor ebx, ebx int 0x80
Assembling and Running the Program
To assemble this program, save it as a file with a .asm
extension (e.g., hello.asm
) and run the following command:
nasm -f elf32 hello.asm -o hello.o
This will produce an object file (hello.o) that can be linked to create an executable. To link and run the program, use the following commands:
ld -m elf_i386 hello.o -o hello ./hello
You should see "Hello, World!" printed to the screen.
Congratulations! You've now been introduced to the world of x86 assembly language and the NASM assembler. As you continue to explore, you'll discover the power and flexibility this low-level programming language offers.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Cratecode Playground (psst, it's free!).
FAQ
What is x86 assembly language?
x86 assembly language is a low-level programming language used for programming x86-based processors. It is a human-readable representation of machine code, consisting of mnemonics and operands that correspond to processor instructions and memory locations. Writing code in assembly language allows programmers to write efficient and highly optimized programs, taking advantage of the processor's capabilities.
What is NASM?
NASM, or the Netwide Assembler, is a popular assembler for x86 architectures. It takes assembly language code as input and generates machine code as output, which can be executed on an x86-based processor. NASM supports a wide range of output formats, including flat binary files, Executable and Linkable Format (ELF), and Microsoft's Portable Executable (PE) format.
How do I install NASM on my computer?
To install NASM on your computer, follow the steps below: For Linux and macOS users:
- Open a terminal window.
- Type
sudo apt-get install nasm
(for Debian-based systems) orbrew install nasm
(for macOS) and press Enter. - Enter your password when prompted, and NASM will be installed. For Windows users:
- Visit the NASM website at https://www.nasm.us/
- Download the latest version for Windows.
- Extract the downloaded archive to a folder of your choice.
- Add the folder containing
nasm.exe
to your system's PATH environment variable.
How do I write a simple "Hello, World!" program in x86 NASM assembly?
To write a "Hello, World!" program in x86 NASM assembly, follow the code below:
section .data hello db 'Hello, World!', 0xA section .text global _start _start: ; Write "Hello, World!" to the screen mov eax, 4 mov ebx, 1 lea ecx, [hello] mov edx, 13 int 0x80 ; Exit the program mov eax, 1 xor ebx, ebx int 0x80
To assemble and run the program, use the following commands:
nasm -f elf64 -o hello.o hello.asm ld -o hello hello.o ./hello
What are some resources to learn x86 NASM assembly?
There are several resources available to learn x86 NASM assembly:
- The NASM documentation: This serves as the official reference for the NASM assembler and provides detailed information on its syntax and usage. It can be found at https://www.nasm.us/doc/
- Online tutorials and courses: Several websites offer in-depth tutorials and courses on x86 assembly language and the NASM assembler. Websites like tutorialspoint.com, asmtutor.com, and coursera.org are good starting points.
- Books: There are numerous books available that cover x86 assembly language and the NASM assembler, such as "Professional Assembly Language" by Richard Blum, and "Assembly Language Step-by-Step" by Jeff Duntemann.
- Forums and online communities: Joining forums and online communities (such as Stack Overflow and Reddit) dedicated to assembly language can provide invaluable support and insights from experienced programmers.