Exploring One-Pass Assemblers
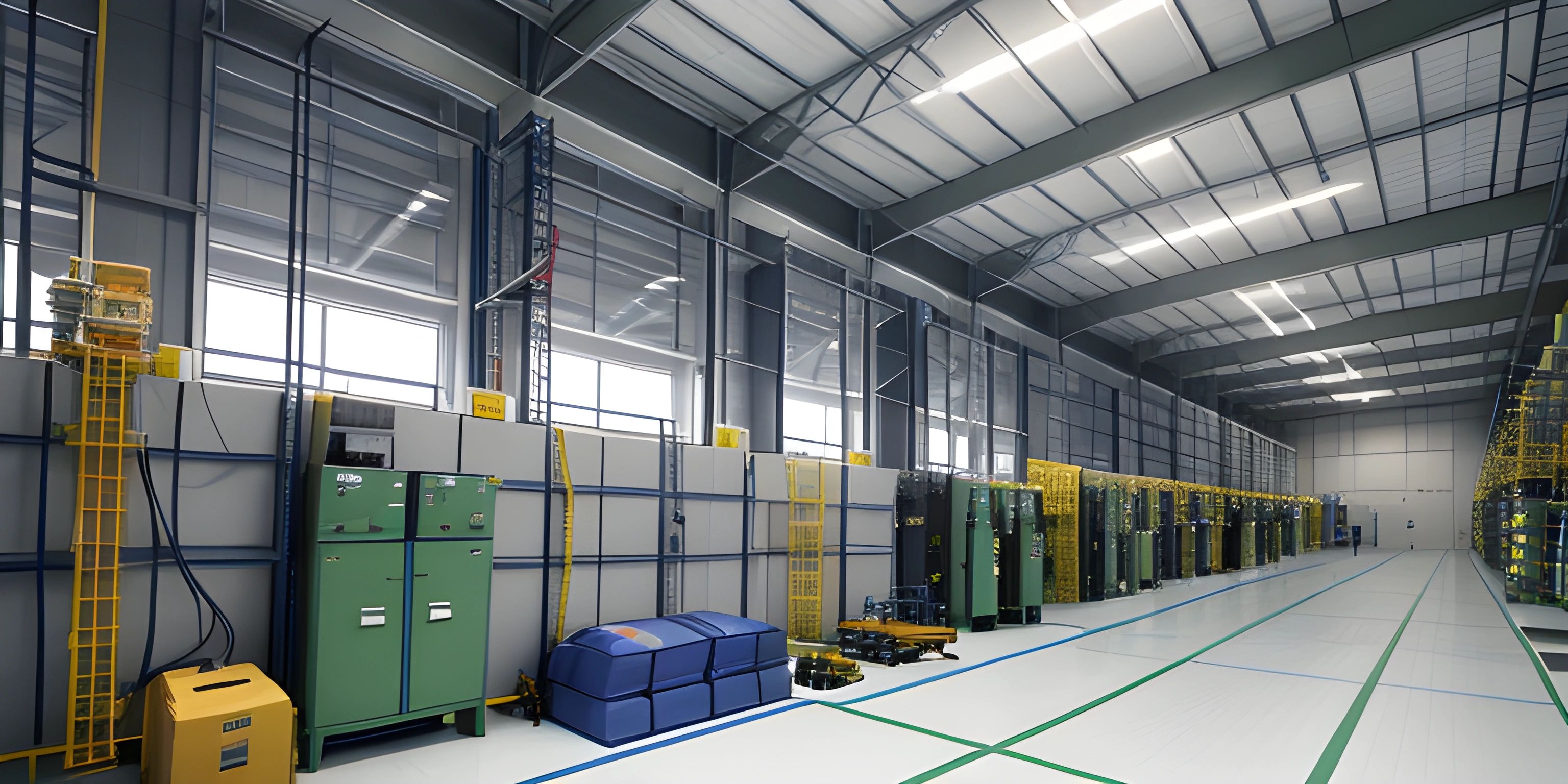
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine you are trying to bake a cake but you can only read the recipe once. You have to remember all the steps and ingredients as you go along, and there's no turning back. This is a bit like how one-pass assemblers work—they read the source code just once and generate the machine code in a single sweep. But is this approach always a piece of cake? Let's find out!
What is a One-Pass Assembler?
A one-pass assembler is a type of assembler that reads the source code in a single pass to produce the object code. Unlike its multi-pass counterparts, it doesn't revisit the source code. This means it has to resolve addresses, labels, and instructions on the fly without any second chances.
How One-Pass Assemblers Work
One-pass assemblers process the source code line by line, translating each line directly to its machine code equivalent. Here’s a simplified example in assembly language:
START: LOAD R1, VALUE ; Load VALUE into register R1 ADD R1, R2 ; Add the value in R2 to R1 STORE R1, RESULT; Store the result back into RESULT HALT ; Stop execution VALUE: .WORD 10 ; Define VALUE with an initial value of 10 RESULT: .WORD 0 ; Define RESULT with an initial value of 0
In a one-pass assembler, the instruction LOAD R1, VALUE
needs to resolve the address of VALUE
immediately. This can be tricky because VALUE
is defined later in the code.
Advantages of One-Pass Assemblers
Speed
Since one-pass assemblers only go through the source code once, they are generally faster than two-pass assemblers. This can be crucial in environments where time is of the essence, like in real-time systems.
Simplicity
One-pass assemblers are simpler to implement because they don't need to manage multiple passes over the code. This simplicity can lead to fewer bugs and easier maintenance.
Resource Efficiency
One-pass assemblers are more memory-efficient because they don't need to store intermediate representations of the source code. This can be important in resource-constrained systems.
Disadvantages of One-Pass Assemblers
Forward References
One of the biggest challenges with one-pass assemblers is handling forward references—references to symbols or addresses that haven't been defined yet. This often requires workarounds like backpatching, which can complicate the assembler.
Limited Optimization
Since one-pass assemblers process the code in a single sweep, they have limited opportunities for code optimization. Two-pass assemblers can collect more information about the code in the first pass, allowing for more sophisticated optimizations in the second pass.
Error Handling
Error handling can be more challenging in one-pass assemblers. Errors may only become apparent after several lines of code have been processed, making it harder to identify and fix the root cause.
One-Pass vs. Two-Pass Assemblers
Flexibility
Two-pass assemblers are more flexible because they can resolve forward references more easily. They can gather all symbol definitions in the first pass and resolve them in the second.
Optimization Opportunities
Two-pass assemblers can apply more advanced optimization techniques because they have a complete view of the code after the first pass. This can lead to more efficient machine code.
Error Detection
Two-pass assemblers often provide better error detection and reporting. Errors can be caught and reported more accurately because the assembler has more context.
When to Use a One-Pass Assembler
One-pass assemblers are a good fit for simple, straightforward programs where speed is critical, and resource constraints are a concern. They are commonly used in embedded systems, where memory and processing power are limited.
However, for more complex programs that require sophisticated optimizations and have numerous forward references, a two-pass assembler might be a better choice.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Getting Complex (psst, it's free!).