TypeScript Interfaces and Classes
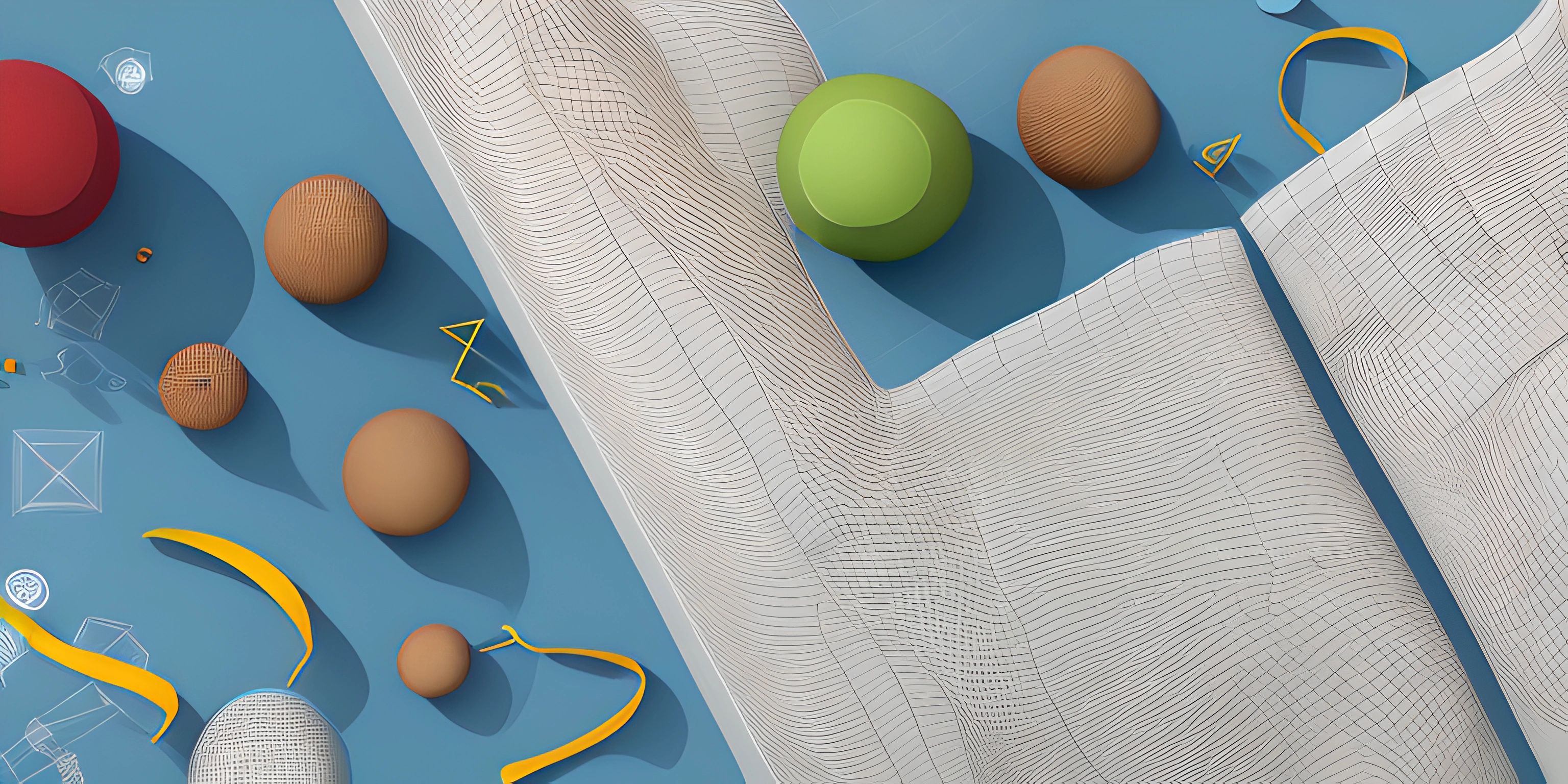
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
To fully harness the power of TypeScript, it's essential to understand two key concepts: interfaces and classes. These features help us write cleaner, more maintainable, and less error-prone code by giving us a way to define the structure and behavior of our data and objects.
Interfaces
In TypeScript, interfaces are a way to define the shape of an object. They provide a blueprint of the properties and types that an object must have. This allows us to enforce a consistent structure for our data and catch errors early, during development.
Here's an example of an interface:
interface Person { firstName: string; lastName: string; age: number; }
This Person
interface defines that any object implementing it must have firstName
, lastName
, and age
properties, with their respective types.
We can use this interface to create an object:
const john: Person = { firstName: "John", lastName: "Doe", age: 30, };
Now, if we try to create an object with missing or incorrect properties, TypeScript will throw an error:
const jane: Person = { firstName: "Jane", lastName: "Doe", // age is missing, TypeScript will complain! };
Interfaces can also define optional properties using the ?
symbol:
interface Employee { firstName: string; lastName: string; age: number; title?: string; }
In this case, title
is an optional property, and we won't get an error if it's not provided when creating an Employee
object.
Classes
Classes are a cornerstone of object-oriented programming (OOP). They define the blueprint for creating objects with specific properties and methods. In TypeScript, classes work together with interfaces to create a powerful and expressive way to create and manage objects.
Here's an example of a class in TypeScript:
class Animal { name: string; species: string; constructor(name: string, species: string) { this.name = name; this.species = species; } speak(): string { return `I'm a ${this.species} named ${this.name}!`; } }
In this example, we define an Animal
class with two properties, name
and species
, and a speak
method. The constructor
is a special method that initializes a new instance of the class.
To create an object of the Animal
class, we use the new
keyword:
const dog = new Animal("Fido", "Dog"); console.log(dog.speak()); // Output: I'm a Dog named Fido!
Classes can also implement interfaces, ensuring that they adhere to the specified structure:
interface Mammal { isWarmBlooded: boolean; } class Human implements Mammal { isWarmBlooded = true; name: string; constructor(name: string) { this.name = name; } }
In this example, the Human
class implements the Mammal
interface, ensuring that it has the isWarmBlooded
property.
By mastering interfaces and classes in TypeScript, you'll be well on your way to writing clean, maintainable, and robust code. These concepts are the foundation of object-oriented programming in TypeScript, and understanding them will open up a world of possibilities for structuring and organizing your code.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).