Creating 2D Animations in Unity
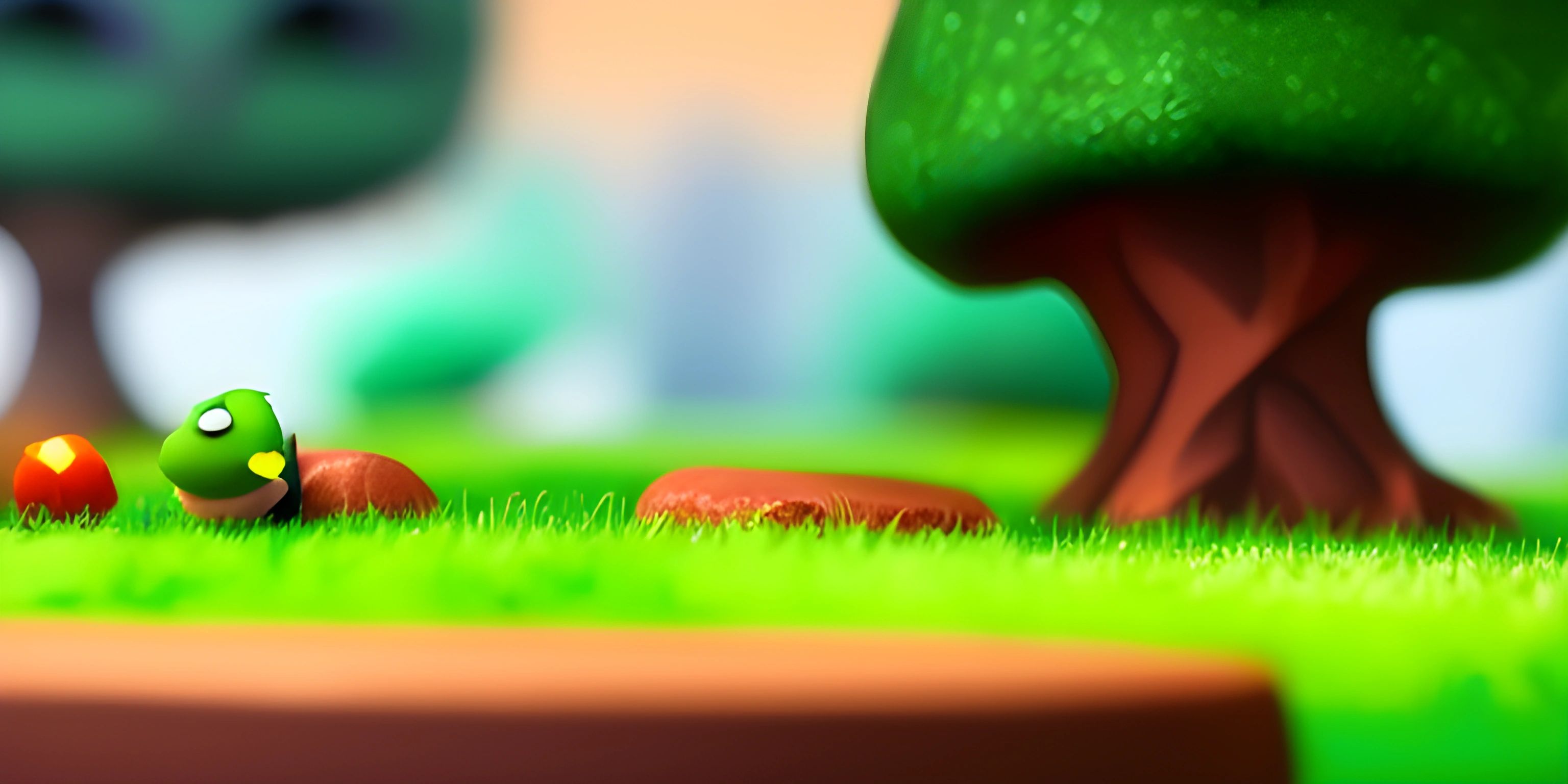
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ready to bring your 2D characters to life in Unity? You're in the right place! Animation in Unity is like giving your drawings a heartbeat. This guide will take you through the magical journey of creating and integrating 2D animations in Unity, so buckle up and let's dive into the enchanting world of moving sprites.
Setting Up Your Project
Before we dive into the fun part, let's set up our project. Open Unity and create a new 2D project. Name it something whimsical like "DancingSprites" to keep things lively!
Importing Sprites
Animations need sprites, and sprites need importing. Head over to your project window and create a new folder named "Sprites". Drag and drop your sprite images or sprite sheet into this folder. If you’re using a sprite sheet, Unity will help you slice it up like a pizza so each piece can be animated individually.
- Select your sprite sheet in the project window.
- In the inspector window, set the Sprite Mode to "Multiple".
- Click on the Sprite Editor button.
- Slice your sprite sheet using Slice > Automatic or manually slice it up as you see fit.
- Click Apply.
Creating Animation Clips
Animation clips are the building blocks of animation in Unity. They are like the individual frames in a flipbook.
- Select your sliced sprites from the project window.
- Drag them into the scene view. Unity will prompt you to create a new animation.
- Save your animation in a new folder named "Animations".
- Watch as Unity generates both an Animator Controller and an Animation Clip.
Open the Animation Window (Window > Animation > Animation) and make sure your new animation is selected. Here, you can tweak the timing of your animation to make it smoother or snappier, depending on your preference.
Animator Controller
The Animator Controller is the brain that controls which animations play and when. Think of it as the director of your animated movie.
- Double-click the Animator Controller generated earlier.
- In the Animator window, you'll see your animation clip as a state. You can add more states (animations) and use transitions to define how your character moves from one animation to another.
For example, you might have a "Walk" animation and an "Idle" animation. You can set up a transition so that when a certain condition is met (like pressing the arrow keys), your character transitions from idle to walking.
Triggering Animations with Code
Now, let's add some C# code to trigger our animations. Create a new C# script named "PlayerController" and attach it to your player object.
Here’s a simple example to get you started:
using UnityEngine; public class PlayerController : MonoBehaviour { private Animator animator; void Start() { // Get the Animator component attached to the player object animator = GetComponent<Animator>(); } void Update() { // Trigger the "Walk" animation when the right arrow key is pressed if (Input.GetKey(KeyCode.RightArrow)) { animator.SetBool("isWalking", true); } else { animator.SetBool("isWalking", false); } } }
This script checks for input and sets a boolean parameter in the Animator, which then triggers the appropriate animation.
Fine-Tuning Animations
Animations need to be fine-tuned to look their best. Use the Animation Window to adjust the timing of your frames. Add events to your animation clips to trigger functions at specific frames. For example, you could make your character play a sound effect every time their foot hits the ground.
Looping and Blending Animations
Looping is essential for animations like walking or running. In the Animation Window, select your animation clip, and in the inspector, check the "Loop Time" box.
Blending allows for smooth transitions between animations. For instance, blending between a walk and idle animation can make your character's movements look more natural. Use parameters in the Animator Controller to control the blend between different states.
Advanced Techniques
Here are some advanced techniques to take your animations to the next level:
- Blend Trees: Use blend trees for complex animations like running, walking, and sprinting.
- Inverse Kinematics (IK): IK allows for realistic limb movements. For example, you can make a character's hand stick to a wall as they walk.
- Animation Events: Trigger custom events at specific points in your animation timeline.
Summary
Creating 2D animations in Unity involves importing sprites, creating animation clips, and using the Animator Controller to manage your animations. By integrating C# scripts and fine-tuning your animations, you can bring your characters to life with smooth, dynamic movements.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making Things Stop (psst, it's free!).
FAQ
How do I import a sprite sheet into Unity?
Drag and drop your sprite sheet into the project window. Select it, set the Sprite Mode to "Multiple" in the inspector, click the Sprite Editor, and slice your sheet.
What is an Animator Controller in Unity?
The Animator Controller is the tool that manages which animations play and when. It allows you to create transitions between different states (animations) based on certain conditions.
How do I trigger animations using C# code?
Attach an Animator component to your game object and control it using C# scripts. Use methods like animator.SetBool
to change parameters in the Animator Controller, which will trigger different animations.
Can I loop animations in Unity?
Yes, you can loop animations by selecting the animation clip in the Animation Window and checking the "Loop Time" box in the inspector.
What are Blend Trees and how are they useful?
Blend Trees allow for smooth transitions between animations, useful for creating complex animations like running, walking, and sprinting. They help make character movements more natural.