P5.js Interactivity
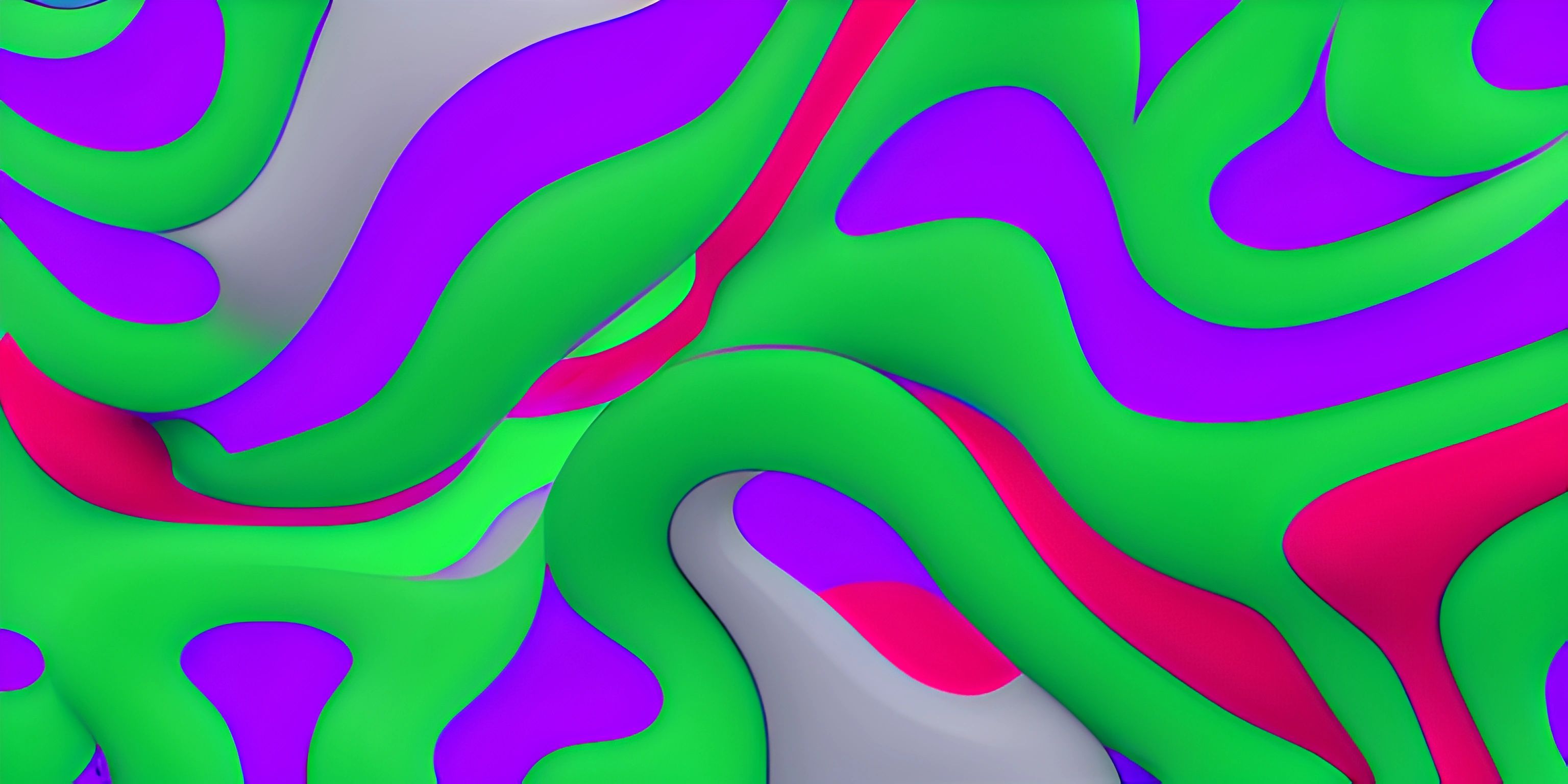
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
If you've ever seen colorful visualizations, interactive animations, or mind-bending art pieces on the web, chances are that some of them were made using p5.js. P5.js is a powerful JavaScript library that allows you to create graphics and interactive experiences on the web. In this article, we'll explore how to make your creations come to life by adding interactivity with p5.js.
Setting Up the Canvas
Before we dive into interactivity, let's set up our p5.js canvas. The canvas is the area on the page where our graphics will be drawn. To create a canvas, we need to define two p5.js functions: setup()
and draw()
.
function setup() { createCanvas(640, 480); } function draw() { background(220); }
In setup()
, we use the createCanvas()
function to specify the size of our canvas, and in draw()
, we set the background color using background()
.
Using Mouse and Keyboard Events
One of the most common ways to add interactivity to your p5.js projects is by using mouse and keyboard events. These events are triggered when the user interacts with the canvas using their mouse or keyboard.
Mouse Events
P5.js provides built-in mouse event functions such as mousePressed()
, mouseReleased()
, mouseClicked()
, mouseMoved()
, and mouseDragged()
.
For instance, let's create a simple example where a circle follows the mouse cursor:
function setup() { createCanvas(640, 480); } function draw() { background(220); fill(255, 0, 0); circle(mouseX, mouseY, 50); }
In this example, we use mouseX
and mouseY
variables to get the current mouse position and draw a circle at that position.
Keyboard Events
Similarly, p5.js provides built-in keyboard event functions such as keyPressed()
, keyReleased()
, and keyTyped()
.
Let's create an example where pressing the "R" key changes the background color to red:
let bgColor = 220; function setup() { createCanvas(640, 480); } function draw() { background(bgColor); } function keyPressed() { if (key == "r" || key == "R") { bgColor = "red"; } }
In this example, we use the keyPressed()
function to detect when the "R" key is pressed, and we change the bgColor
variable accordingly.
Using the p5.js map()
Function
The map()
function is incredibly useful when working with interactivity in p5.js. It allows you to scale a value from one range to another. For instance, if you want to change the size of a shape based on the mouse position, you can use map()
to accomplish this.
Let's create an example where the circle's size changes based on the mouse's X position:
function setup() { createCanvas(640, 480); } function draw() { background(220); let circleSize = map(mouseX, 0, width, 10, 200); circle(mouseX, mouseY, circleSize); }
In this example, we use map()
to scale the mouseX position from the range of 0 to the canvas width, to a new range of 10 to 200 for the circle's size.
Wrapping Up
Interactivity is a key ingredient in making your p5.js creations engaging and dynamic. By leveraging mouse and keyboard events, and using functions like map()
, you can create interactive experiences that respond to user input and keep your audience captivated. Now it's time to use these concepts and let your creativity run wild!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Drawing Basic Shapes (psst, it's free!).
FAQ
What is p5.js and why should I use it for interactivity?
p5.js is a JavaScript library designed to make it easy to create graphics and interactive experiences in a web browser. It is based on the popular Processing programming language, but with a focus on JavaScript and the web. P5.js simplifies the process of creating visual elements and interactivity, making it perfect for artists, designers, and beginners who want to create engaging web experiences without diving too deep into complex programming concepts.
How do I get started with p5.js and creating interactive elements?
To get started with p5.js, follow these steps:
- Download the p5.js library from the official website (https://p5js.org/download/).
- Create an HTML file and include the p5.js library using a script tag.
- Add a script tag for your custom p5.js code.
- Create a
setup()
function to define your canvas and initialize your sketch. - Create a
draw()
function to continuously update your sketch and handle interactivity. - Use p5.js built-in functions, such as
mousePressed()
andkeyPressed()
, to create interactive elements. Here's a simple example of a p5.js sketch:
<!DOCTYPE html> <html> <head> <script src="p5.js"></script> <script> function setup() { createCanvas(400, 400); } function draw() { background(220); if (mouseIsPressed) { fill(0); } else { fill(255); } ellipse(mouseX, mouseY, 80, 80); } </script> </head> <body> </body> </html>
Can I use p5.js with other JavaScript libraries for enhanced interactivity?
Absolutely! P5.js can be easily integrated with other JavaScript libraries to create even more engaging and interactive experiences. Some popular libraries that work well with p5.js include Three.js for 3D graphics, Tone.js for audio, and Socket.io for real-time communication between users. Just remember to include the additional libraries in your HTML file and make sure they are compatible with p5.js.
How can I detect user input, like mouse clicks and keyboard presses, in p5.js?
P5.js provides several built-in functions to handle user input, such as:
mousePressed()
: This function is called once when the user presses the mouse button.mouseReleased()
: This function is called once when the user releases the mouse button.mouseClicked()
: This function is called once after a mouse button has been pressed and released.keyPressed()
: This function is called once when a key is pressed.keyReleased()
: This function is called once when a key is released. You can define these functions in your p5.js sketch to detect and respond to user input. For example, you might change the color of a shape when the user clicks on it, or move an object based on arrow key presses.
How can I create animations and transitions in p5.js?
Animations and transitions in p5.js can be created using the draw()
function, which is called continuously at the frame rate set by the frameRate()
function (default is 60 frames per second). By changing the properties of visual elements, such as position, size, or color, you can create smooth animations and transitions.
For example, here's a simple animation of a circle moving across the canvas:
let x = 0; function setup() { createCanvas(400, 400); } function draw() { background(220); fill(255, 0, 0); ellipse(x, 200, 50, 50); x += 2; if (x > width) { x = 0; } }
You can also use built-in easing functions like lerp()
or external libraries like GSAP for more advanced animations and transitions.