Creating User Interfaces in Unity
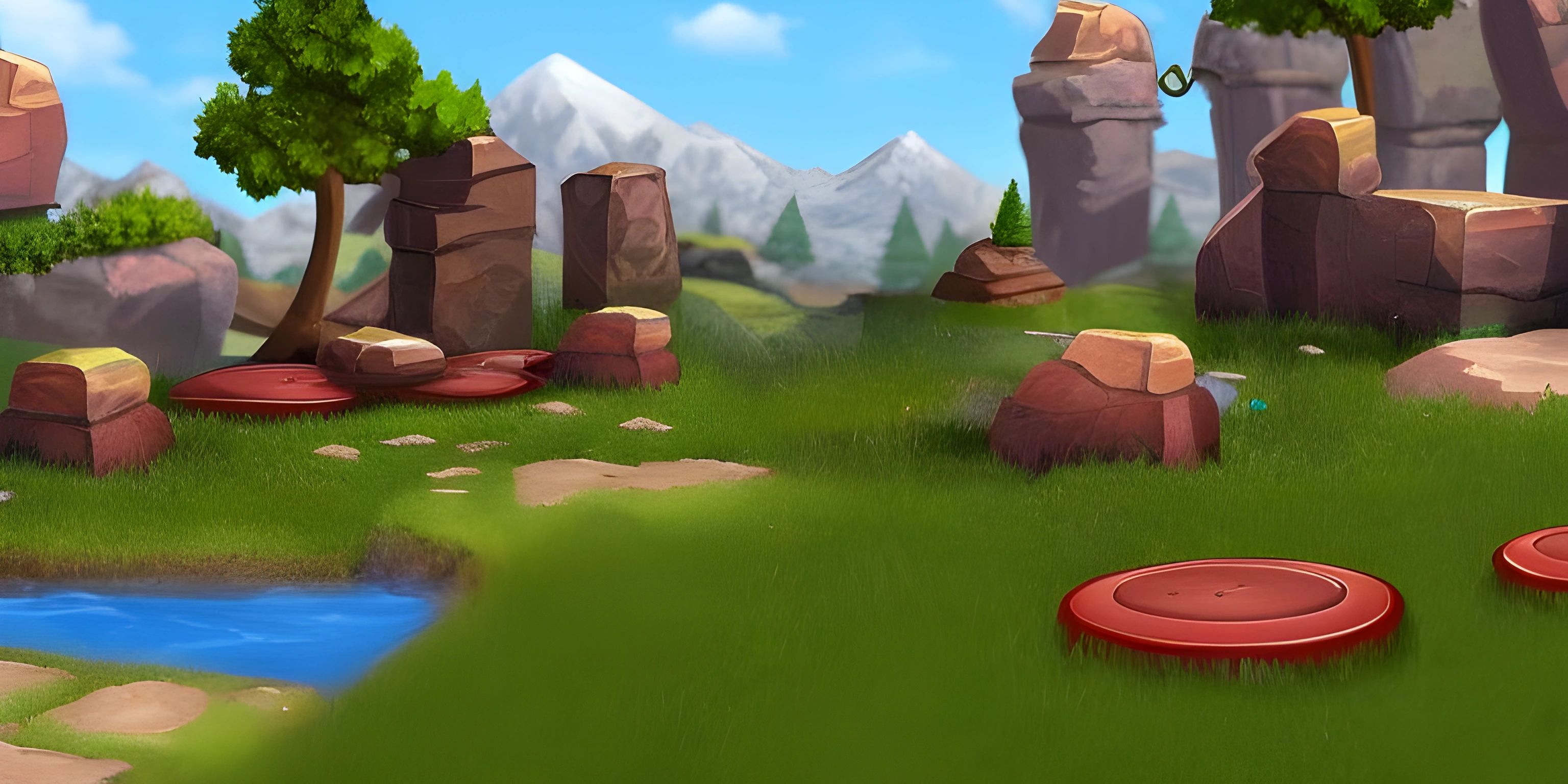
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
User interfaces (UI) are the unsung heroes of game development. They’re the friendly faces that guide players through menus, inform them of their health, score, and other vital stats, and generally make the game a more enjoyable experience. Imagine playing your favorite game without any menus or HUDs—it would be like trying to navigate a maze blindfolded! So let’s dive into the magical world of UI creation in Unity.
Getting Started with Unity UI
First things first, to create a UI in Unity, you need to understand the Canvas. The Canvas is like your blank slate, the stage on which all UI elements perform their roles.
When you create a new UI element, Unity automatically creates a Canvas for you if one doesn’t already exist. This is where all your UI elements will live—like an artist's canvas, but with fewer paint splatters and more code.
Adding UI Elements
Buttons
Let’s start with buttons. They’re the bread and butter of any UI. Here’s how to add a button to your Canvas:
- Right-click on the Canvas in the Hierarchy window.
- Select
UI > Button
.
You’ll see a new button appear on your Canvas, along with a Text child object. This text is what gets displayed on the button.
To change the button text, simply click on the Text object and modify the Text
property in the Inspector window.
using UnityEngine; using UnityEngine.UI; public class ButtonExample : MonoBehaviour { public Button myButton; void Start() { // Assign a method to the button click event myButton.onClick.AddListener(OnButtonClick); } void OnButtonClick() { Debug.Log("Button was clicked!"); } }
In this script, we have a button that logs a message when clicked. Simple, yet effective!
Text Elements
Text elements are equally important in conveying information. Adding text to your UI is as simple as adding a button:
- Right-click on the Canvas.
- Select
UI > Text
.
You can then adjust the text properties, such as font size, color, and alignment, in the Inspector window.
using UnityEngine; using UnityEngine.UI; public class TextExample : MonoBehaviour { public Text myText; void Start() { myText.text = "Hello, Unity UI!"; } }
This script sets the text to “Hello, Unity UI!” when the game starts. You can also dynamically update text elements based on in-game events, providing real-time feedback to the player.
Layouts
Layouts in Unity help organize your UI elements in a structured manner. Think of it as the Marie Kondo method for UI—everything has its place.
Horizontal and Vertical Layout Groups
Horizontal and Vertical Layout Groups are used to arrange UI elements in rows or columns. Here’s how to use a Vertical Layout Group:
- Create an empty GameObject as a child of the Canvas.
- Attach a
Vertical Layout Group
component to the GameObject. - Add UI elements (like buttons or text) as children of this GameObject.
The Vertical Layout Group will automatically arrange them in a vertical stack.
using UnityEngine; using UnityEngine.UI; public class LayoutExample : MonoBehaviour { public GameObject[] uiElements; void Start() { VerticalLayoutGroup layoutGroup = GetComponent<VerticalLayoutGroup>(); foreach (GameObject element in uiElements) { element.transform.SetParent(layoutGroup.transform, false); } } }
In this example, all UI elements in the uiElements
array are added to a Vertical Layout Group.
Grid Layout Group
The Grid Layout Group is perfect for creating grid-based layouts, such as inventories or level selectors:
- Create an empty GameObject under the Canvas.
- Attach a
Grid Layout Group
component to it. - Add UI elements as children.
The Grid Layout Group will organize them into a grid based on the settings you provide.
using UnityEngine; using UnityEngine.UI; public class GridLayoutExample : MonoBehaviour { public GameObject uiElementPrefab; public int columns = 3; public int rows = 3; void Start() { GridLayoutGroup gridLayout = GetComponent<GridLayoutGroup>(); for (int i = 0; i < columns * rows; i++) { GameObject element = Instantiate(uiElementPrefab, gridLayout.transform); } } }
This script dynamically creates a grid of UI elements based on the specified number of columns and rows.
Anchors and Pivots
Anchors and pivots are crucial for responsive UI design. They determine how UI elements scale and position relative to their parent Canvas.
- Anchors define the position and size of a UI element relative to its parent.
- Pivots determine the point around which a UI element scales and rotates.
To adjust anchors and pivots, select a UI element and modify its Rect Transform properties in the Inspector.
Conclusion
Creating a user interface in Unity is like orchestrating a symphony—each element has its role, but together they create a harmonious experience. From buttons to text elements, and layouts to anchors, understanding these components will elevate your game design skills.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Story Builder (psst, it's free!).
FAQ
How do I change the text on a Unity button?
To change the text on a Unity button, select the Text child object of the button and modify the Text
property in the Inspector window.
What is the Canvas in Unity?
The Canvas is a GameObject in Unity that acts as the stage for all UI elements. It ensures that UI elements are rendered correctly on the screen.
How do I create a responsive UI in Unity?
Creating a responsive UI involves using anchors and pivots to ensure UI elements scale and position correctly on different screen sizes. Adjust these properties in the Rect Transform component.
Can I dynamically update UI elements during gameplay?
Yes, you can dynamically update UI elements via scripts. For instance, you can change the text of a Text element or the color of a button based on in-game events.
What are Layout Groups in Unity?
Layout Groups, such as Horizontal, Vertical, and Grid Layout Groups, are used to arrange UI elements in a structured manner. They help organize elements into rows, columns, or grids.