Version Control with Git
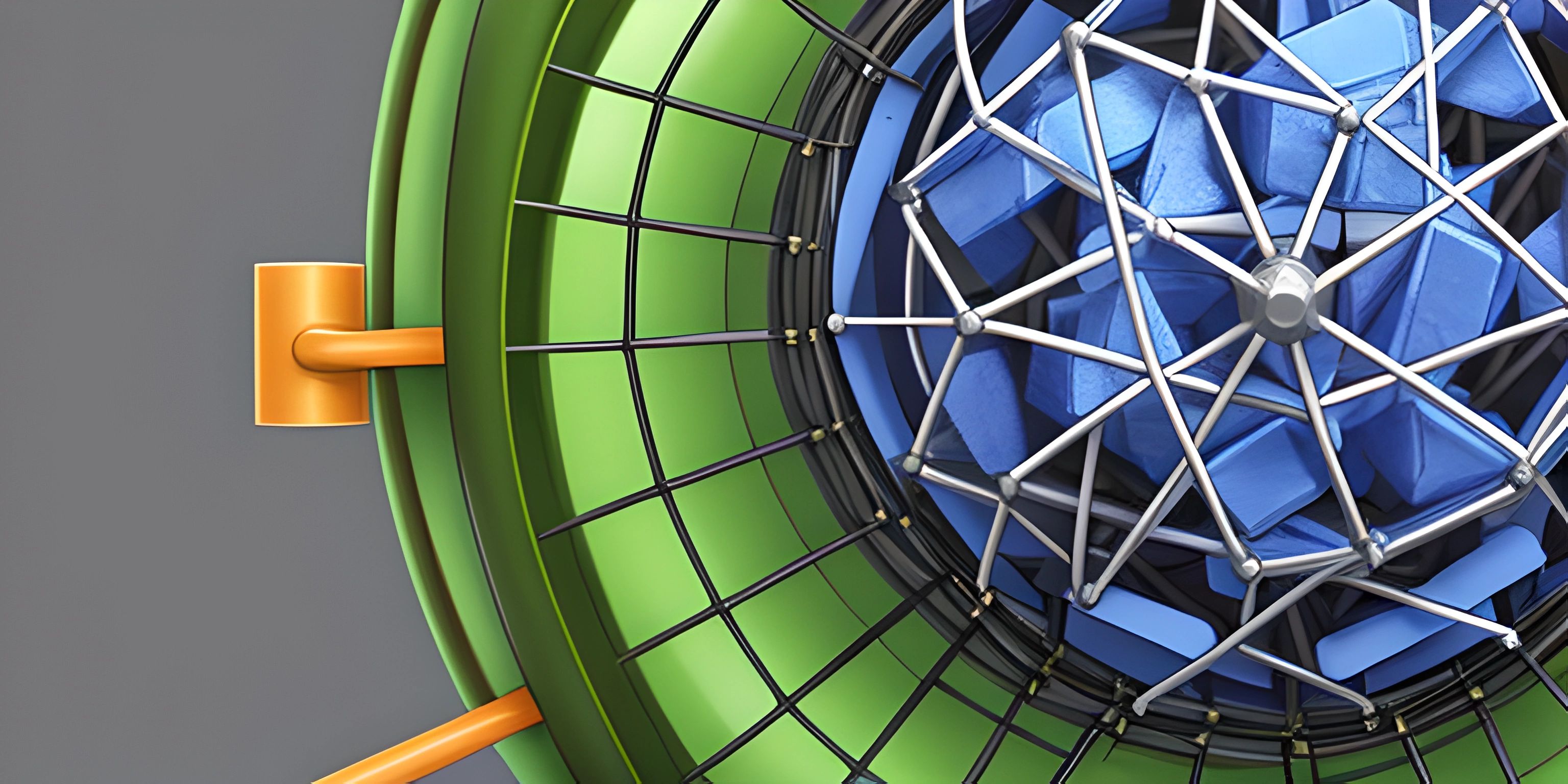
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Git is a widely-used version control system that helps developers manage and collaborate on code projects. It allows you to track changes, revert to previous versions, and work with others on the same project. In this article, we will cover the basics of Git and how to use it for version control.
What is Git?
Git is a distributed version control system, which means that each developer has a local copy of the entire project history. This allows for easy collaboration, as developers can work on their own branches and then merge their changes back into the main project.
Installing Git
To get started with Git, you need to install it on your computer. You can download the latest version from the official Git website.
For Linux, you can use the following command to install Git:
sudo apt-get install git
For macOS, you can use the following command to install Git using Homebrew:
brew install git
Basic Git Commands
Here are some basic Git commands to help you get started:
Initialize a Git repository
To create a new Git repository, navigate to your project folder in the terminal and run:
git init
Clone a Git repository
To create a local copy of an existing Git repository, run:
git clone <repository-url>
Replace <repository-url>
with the URL of the Git repository you want to clone.
Add files to the staging area
To start tracking changes to a file or add a new file to the repository, use the git add
command:
git add <file-name>
Commit changes
To save the changes you've made to the staging area, use the git commit
command:
git commit -m "Your commit message here"
Check the status of your repository
To see the current status of your repository, including any changes you've made, use the git status
command:
git status
Create a new branch
To create a new branch in your repository, use the git checkout
command:
git checkout -b <branch-name>
Switch between branches
To switch between branches, use the git checkout
command:
git checkout <branch-name>
Merge branches
To merge changes from one branch into another, first switch to the target branch using git checkout
, and then run:
git merge <source-branch-name>
Push changes to a remote repository
To push your local changes to a remote repository, use the git push
command:
git push origin <branch-name>
Pull changes from a remote repository
To update your local repository with the latest changes from a remote repository, use the git pull
command:
git pull origin <branch-name>
These are just the basics of Git. There's a lot more you can do with it, but this should be enough to get you started with managing and collaborating on code projects using Git for version control.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
What is Git and why is it important for version control?
Git is a widely-used distributed version control system designed to track changes in source code or files. It allows multiple developers to work on a project simultaneously, enabling collaboration, tracking changes, and resolving conflicts. Version control is important as it helps maintain a history of changes, allows reverting to a previous version, and improves overall project management.
How do I get started with Git for my code projects?
To get started with Git, follow these steps:
- Download and install Git from the official website (https://git-scm.com).
- Set up your Git account with your name and email address using the following commands:
git config --global user.name "Your Name" git config --global user.email "[email protected]"
- Create a new repository or clone an existing one.
- Make changes to your files, stage them, and commit your changes with proper commit messages.
- Push your changes to a remote repository, like GitHub or GitLab, to collaborate with others.
How do I create a new Git repository or clone an existing one?
To create a new repository, navigate to your project directory using the command line or terminal, then run git init
. This initializes a new Git repository in the current directory. To clone an existing repository, use the command git clone [repository_url]
, replacing [repository_url]
with the URL of the remote repository you want to clone.
What are the basic Git commands for managing changes in my project?
Here are some fundamental Git commands:
git status
: Shows the status of your working directory, including modified, staged, and untracked files.git add [file]
: Adds the specified file to the staging area, preparing it for a commit.git commit -m "Commit message"
: Commits the staged changes with a descriptive commit message.git log
: Shows a log of all the commits in the repository.git diff
: Displays the differences between the working directory and the latest commit.
How do I collaborate with others using Git?
To collaborate with others using Git, follow these steps:
- Create a remote repository on a platform like GitHub or GitLab and push your local repository to it.
- Share the repository URL with your collaborators, allowing them to clone and work on the project.
- Use branches to work on separate features or bug fixes without affecting the main branch.
- When a feature or fix is complete, create a pull request or merge request to propose merging the changes into the main branch.
- Review, discuss, and approve the changes before merging them.