x86 Assembly NASM User Input and Output
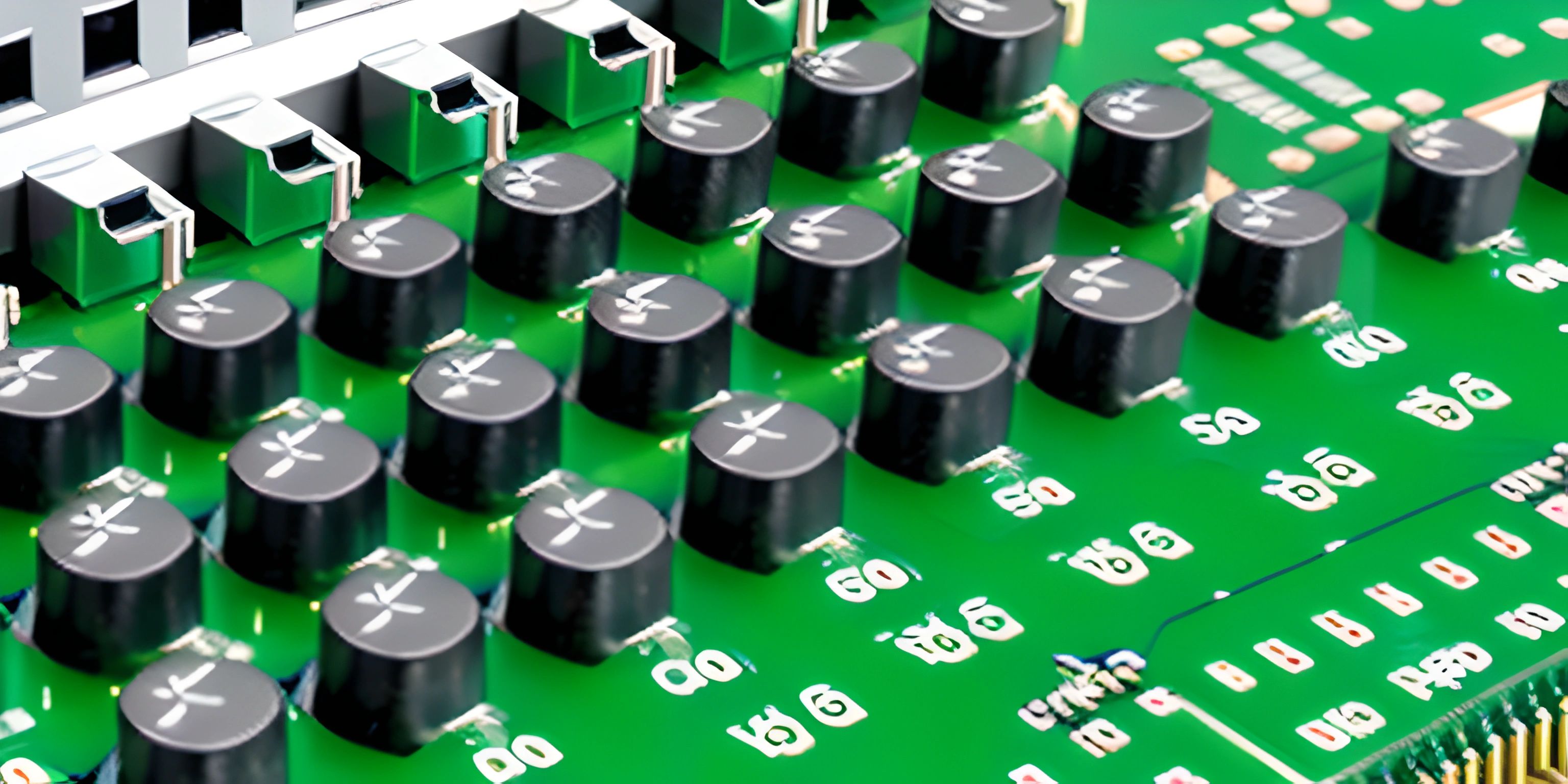
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When programming in x86 Assembly using the Netwide Assembler (NASM), handling user input and output can be a daunting task at first. But fear not! We're here to guide you through the process of reading and writing numbers like a pro.
System Calls
To interact with the operating system and perform input/output operations, we'll use system calls. These are functions provided by the kernel, allowing programs to request services such as reading from the keyboard or writing to the screen.
x86 System Call Conventions
Before diving into reading and writing numbers, it's crucial to understand the x86 system call conventions. On x86, the system call number is placed in the eax
register, while the function's arguments are stored in the ebx
, ecx
, edx
, esi
, and edi
registers, in that order.
To invoke a system call, we use the int 0x80
instruction. After the system call is executed, the result is stored in the eax
register.
Writing Numbers
For our first feat, let's see how to write an integer to the console. We'll use the write
system call (number 4) to accomplish this task.
Here's a step-by-step guide to writing an integer:
- Convert the integer to its string representation (e.g., from 42 to "42").
- Place the file descriptor for stdout (1) in the
ebx
register. - Put the memory address of the string in the
ecx
register. - Store the string length in the
edx
register. - Set the
eax
register to 4 (write syscall). - Call the
int 0x80
instruction.
; Write the number 42 to the console section .data num db 42 section .bss buffer resb 16 section .text global _start _start: ; Convert number to string mov eax, [num] lea edi, [buffer + 15] mov byte [edi], 0x0A ; newline character convert: dec edi xor edx, edx mov ecx, 10 div ecx add dl, '0' mov [edi], dl test eax, eax jnz convert ; Write to console mov eax, 4 mov ebx, 1 lea ecx, [edi] lea edx, [buffer + 16] sub edx, ecx int 0x80 ; Exit mov eax, 1 xor ebx, ebx int 0x80
Reading Numbers
Now that we've mastered writing numbers let's move on to reading them from the user. We'll use the read
system call (number 3) to do so.
Here's a step-by-step guide to reading an integer:
- Place the file descriptor for stdin (0) in the
ebx
register. - Put the memory address of a buffer to store the input in the
ecx
register. - Store the buffer size in the
edx
register. - Set the
eax
register to 3 (read syscall). - Call the
int 0x80
instruction. - Convert the received string into an integer (e.g., from "42" to 42).
; Read a number from the user and store it in the `eax` register section .bss buffer resb 16 section .text global _start _start: ; Read from console mov eax, 3 mov ebx, 0 lea ecx, [buffer] mov edx, 16 int 0x80 ; Convert string to number xor eax, eax lea esi, [buffer] convert: movzx edx, byte [esi] cmp dl, 0x0A ; newline character je done imul eax, 10 sub edx, '0' add eax, edx inc esi jmp convert done: ; Exit mov eax, 1 xor ebx, ebx int 0x80
With these examples, you're ready to read and write numbers in your x86 Assembly programs using NASM. As you practice, you'll find these operations are fundamental building blocks for more advanced tasks like arithmetic, sorting, and searching. So, go forth and conquer the world of x86 Assembly, one number at a time!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Basic Concepts (psst, it's free!).
FAQ
What is the basic method for reading and writing user input/output in x86 Assembly using NASM?
In x86 Assembly using NASM, you can utilize system calls to handle user input and output. The essential steps are:
- Load the appropriate system call number in the EAX register.
- Set up the function arguments in the corresponding registers.
- Execute the
int 0x80
instruction to make the system call. - Handle the return value, if necessary.
How can I read an integer from the user using x86 Assembly and NASM?
To read an integer from the user, follow these steps:
; Read integer system call mov eax, 3 ; sys_read mov ebx, 0 ; standard input (stdin) lea ecx, [buffer] ; memory location to store the input mov edx, 4 ; read 4 bytes (32-bit integer) int 0x80 ; make the system call
Make sure to define a buffer to store the input, like this:
section .bss buffer resd 1 ; reserve space for one dword (4 bytes)
How can I print an integer to the console using x86 Assembly and NASM?
To print an integer to the console, follow these steps:
; Write integer system call mov eax, 4 ; sys_write mov ebx, 1 ; standard output (stdout) lea ecx, [buffer] ; memory location of the integer to print mov edx, 4 ; print 4 bytes (32-bit integer) int 0x80 ; make the system call
Make sure the integer you want to print is stored in the buffer, like this:
section .data buffer dd 42 ; store the integer 42 in the buffer
How do I handle the return value of a system call in x86 Assembly using NASM?
After executing a system call with int 0x80
, the return value will be stored in the EAX register. You can then check and handle the return value as needed. For example, if a negative value is returned, it indicates an error:
int 0x80 ; make the system call cmp eax, 0 ; compare the return value with 0 jl error_handler ; jump to error_handler if return value is negative
What are some common system call numbers used in x86 Assembly and NASM?
A few common system call numbers are:
- 1: sys_exit - exit a program
- 3: sys_read - read data from a file descriptor (e.g., standard input)
- 4: sys_write - write data to a file descriptor (e.g., standard output)
- 5: sys_open - open a file You can find a more comprehensive list in the Linux system call table.