x86 Assembly Introduction
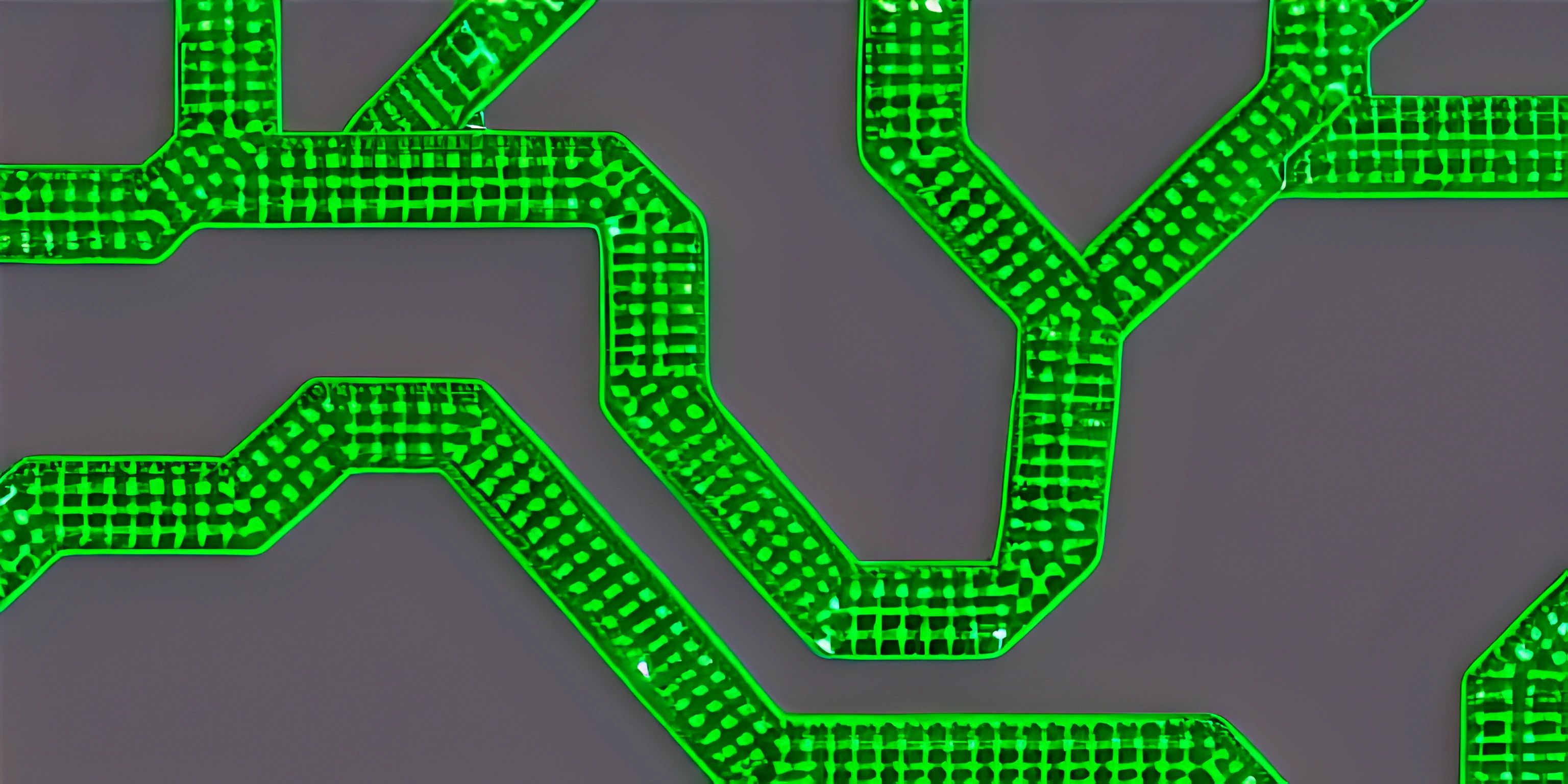
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Diving into the world of x86 assembly language might feel like you're being teleported to an alien planet, but fear not! We'll help you unravel the mysteries and understand the basic concepts of this low-level programming language. It's time to put on your space suit and explore the x86 universe!
What is x86 Assembly Language?
x86 assembly language is a low-level programming language used to communicate directly with computer hardware, specifically the x86 family of processors. It's like speaking the native tongue of your computer's processor. You know, like the secret code you and your childhood friend made up to keep your conversations private from your annoying siblings.
Unlike high-level languages like Python or Java, assembly languages are generally specific to a particular computer architecture. This means x86 assembly code written for an x86 processor won't work on, say, an ARM processor without some serious modifications.
Registers
In the x86 assembly world, registers are the superstars. They're small, super-fast storage locations within the processor that hold data and addresses. Imagine them as the processor's tiny personal assistants, always there to lend a helping hand.
There are several types of registers in x86 assembly, including:
- General-purpose registers: These are the multitaskers, handling a variety of tasks like holding data, addresses, and intermediate results. Some of the most common ones include EAX, EBX, ECX, and EDX.
- Segment registers: These help with memory management by keeping track of memory segments. Some examples are CS (Code Segment), DS (Data Segment), and SS (Stack Segment).
- Pointer and index registers: These registers are all about navigation. They help manage the stack (ESP and EBP) and assist with array and string operations (ESI and EDI).
Instructions
To communicate with the processor, we use instructions. These are the building blocks of x86 assembly code and tell the processor what to do. It's like giving your dog commands like "sit" or "fetch." Some basic x86 assembly instructions include:
- MOV: The most common instruction, MOV transfers data between registers or between a register and memory.
- ADD, SUB: As you might expect, these instructions add or subtract values in registers or memory.
- PUSH, POP: These instructions help manage the stack by pushing values onto it or popping them off.
- CMP, TEST: These instructions compare values and set flags accordingly.
Here's a simple example of x86 assembly code to add two numbers:
MOV EAX, 5 MOV EBX, 3 ADD EAX, EBX
In this example, we load the value 5 into the EAX register, 3 into the EBX register, and then add the values in EAX and EBX, storing the result (8) in the EAX register.
Assemblers and Disassemblers
To convert x86 assembly code into machine code (binary) that the processor can understand, we use an assembler. It's like a magical translator that turns your assembly code into a language the processor can speak. Some popular x86 assemblers include NASM and GAS.
On the flip side, disassemblers take machine code and turn it back into assembly code, like a reverse translator. This can be helpful for debugging and reverse engineering purposes.
Conclusion
While x86 assembly language might seem intimidating at first, understanding its basic concepts and structure can help you get started on your journey to becoming an assembly language guru. Remember, practice makes perfect. So, keep exploring and experimenting with x86 assembly code, and soon you'll be able to bend your computer's processor to your will. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is x86 assembly language?
x86 assembly language is a low-level programming language used to communicate directly with a computer's hardware. It is specific to the x86 architecture, which is a family of instruction set architectures initially developed by Intel. Assembly languages are more readable than machine code but still require a deep understanding of the underlying hardware.
Why should I learn x86 assembly language?
Learning x86 assembly language has several benefits, including:
- Gaining a deep understanding of computer hardware and how it operates.
- Writing efficient and highly optimized code, which is crucial in certain applications like device drivers, firmware, and performance-critical software.
- Debugging and reverse engineering software, since understanding assembly can help identify issues in compiled code.
- Expanding your programming skills and knowledge, which can be valuable in various areas of computer science and software engineering.
How do I get started with x86 assembly programming?
To get started with x86 assembly programming, you need to:
- Familiarize yourself with the basic concepts of assembly language and x86 architecture, such as registers, memory addressing, and instruction set.
- Choose an assembler, which is a program that translates assembly code into machine code. Popular assemblers for x86 include NASM and GAS.
- Set up a development environment that includes an assembler, a text editor or IDE for writing code, and a debugger to inspect and troubleshoot your programs.
- Learn the x86 assembly syntax and practice writing programs, starting with simple examples and gradually moving on to more complex tasks.
What are some fundamental concepts of x86 assembly language?
Some fundamental concepts of x86 assembly language include:
- Registers: Small, fast storage locations within the CPU that hold data and instructions.
- Memory addressing: Techniques to access and manipulate data stored in memory, such as direct, indirect, and indexed addressing.
- Instruction set: A collection of commands that the CPU can execute, including arithmetic, logical, control flow, and data movement operations.
- Assembly syntax: The rules and conventions for writing x86 assembly code, which may vary between different assemblers.
- Calling conventions: Standardized ways of passing parameters between functions and handling the call stack, which may differ depending on the operating system and programming language used.
Can I use x86 assembly language for modern 64-bit systems?
Yes, you can use x86 assembly language for modern 64-bit systems. In fact, x86-64 (also known as x64, AMD64, or Intel 64) is an extension of the x86 instruction set, specifically designed for 64-bit systems. While the overall principles remain the same, x86-64 introduces new registers, instructions, and addressing modes to handle the increased memory address space and processing capabilities. When programming for 64-bit systems, you'll need to use an assembler that supports the x86-64 instruction set and follow the appropriate calling conventions for your target operating system.