x86 NASM Introduction
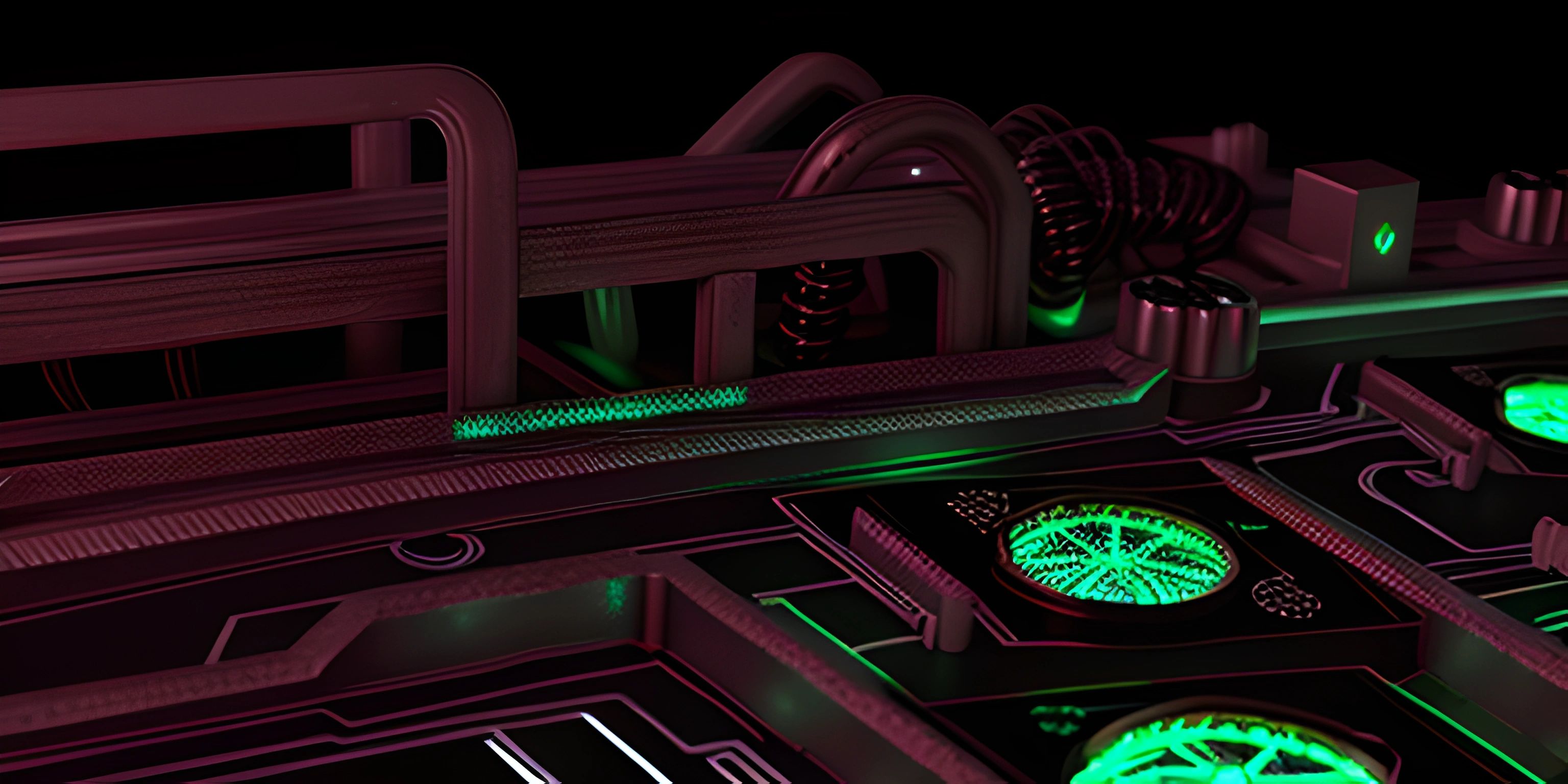
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
x86 NASM is an assembly language for the x86 family of processors. It's a low-level language that is closer to the hardware, allowing for finer control and optimization of your code. While most modern programming is done using higher-level languages, NASM still has its place in the world of programming for tasks that require maximum performance and control.
x86 Architecture
The x86 architecture is a family of processors developed by Intel, starting with the 8086 processor in 1978. This architecture has grown and evolved over time with additions like 32-bit (IA-32) and 64-bit (x86-64) versions. The x86 architecture is widely used in desktops, laptops, and servers, making a fundamental understanding of x86 NASM assembly valuable for programmers working with these systems.
NASM: The Netwide Assembler
NASM (Netwide Assembler) is an assembler and disassembler for the x86 architecture. An assembler is a program that translates human-readable assembly language into machine code, which can then be executed by the processor. NASM is widely used because of its simple syntax, rich feature set, and extensive documentation.
Why Learn x86 NASM Assembly?
While higher-level languages like Python and JavaScript offer great productivity and ease of use, there are situations where using assembly language can be beneficial:
- Performance: Writing code in assembly language allows you to optimize for speed and minimize memory usage, which can be critical in performance-critical applications.
- Hardware Control: Assembly provides direct access to hardware, enabling you to write code for device drivers, operating systems, and firmware.
- Education: Learning assembly language deepens your understanding of how processors work, making you a better programmer overall.
Basic x86 NASM Syntax
x86 NASM assembly uses a simple and easy-to-understand syntax. Here's a basic example:
section .data message db "Hello, world!", 0 section .text global _start _start: ; Write the message to stdout mov eax, 4 ; sys_write system call mov ebx, 1 ; file descriptor (stdout) lea ecx, [message] ; pointer to the message mov edx, 13 ; length of the message int 0x80 ; call the kernel ; Exit the program mov eax, 1 ; sys_exit system call xor ebx, ebx ; exit status (0) int 0x80 ; call the kernel
This code demonstrates how to write a simple "Hello, world!" program in x86 NASM assembly. It is divided into two sections: .data
for data storage and .text
for the actual code. The _start
label is the entry point of the program, and the code uses system calls to write the message to stdout and exit the program.
Conclusion
While x86 NASM assembly might not be the go-to language for everyday programming tasks, it holds a significant place in the world of programming. Understanding assembly language, especially for the widely used x86 architecture, can improve your programming skills and open up new opportunities in performance optimization, hardware control, and education. Happy assembling!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is x86 NASM assembly language?
x86 NASM assembly language is a low-level programming language used to write programs for x86-based processors. It is designed to be human-readable and is closely related to machine code. NASM (Netwide Assembler) is a popular assembler for x86 architecture that converts assembly language code into machine-readable object files.
Why would someone choose to use x86 NASM assembly language?
Using x86 NASM assembly language allows programmers to write highly optimized and efficient code, as it provides direct control over hardware resources. This can be beneficial for tasks like system programming, debugging, reverse engineering, and writing performance-critical code. However, it requires a good understanding of computer architecture and may be more challenging to learn compared to higher-level languages.
How do I write a simple "Hello, World!" program in x86 NASM assembly?
Here's a basic "Hello, World!" program in x86 NASM assembly:
; hello.asm section .data hello db 'Hello, World!',0 section .text global _start _start: ; Write 'Hello, World!' to the console mov eax, 4 ; sys_write mov ebx, 1 ; file descriptor (stdout) lea ecx, [hello] ; address of the string mov edx, 13 ; length of the string int 0x80 ; call kernel ; Exit the program mov eax, 1 ; sys_exit xor ebx, ebx ; exit code 0 int 0x80 ; call kernel
To assemble and link the program, use the following NASM and GCC commands:
nasm -f elf32 -o hello.o hello.asm gcc -m32 -o hello hello.o
What are some resources for learning x86 NASM assembly language?
There are numerous online resources and books available to help you learn x86 NASM assembly language. Some popular ones include:
- The NASM documentation: https://www.nasm.us/doc/
- Programming from the Ground Up by Jonathan Bartlett: http://download-mirror.savannah.gnu.org/releases/pgubook/
- x86 Assembly Guide by the University of Virginia Computer Science: https://www.cs.virginia.edu/~evans/cs216/guides/x86.html
Can I use x86 NASM assembly language to write programs for other processor architectures?
No, x86 NASM assembly language is specific to x86 processors. If you want to write assembly code for other processor architectures, you'll need to use a different assembly language and assembler tailored to that specific architecture. Examples include ARM assembly for ARM processors or MIPS assembly for MIPS processors.