Algorithm Basics
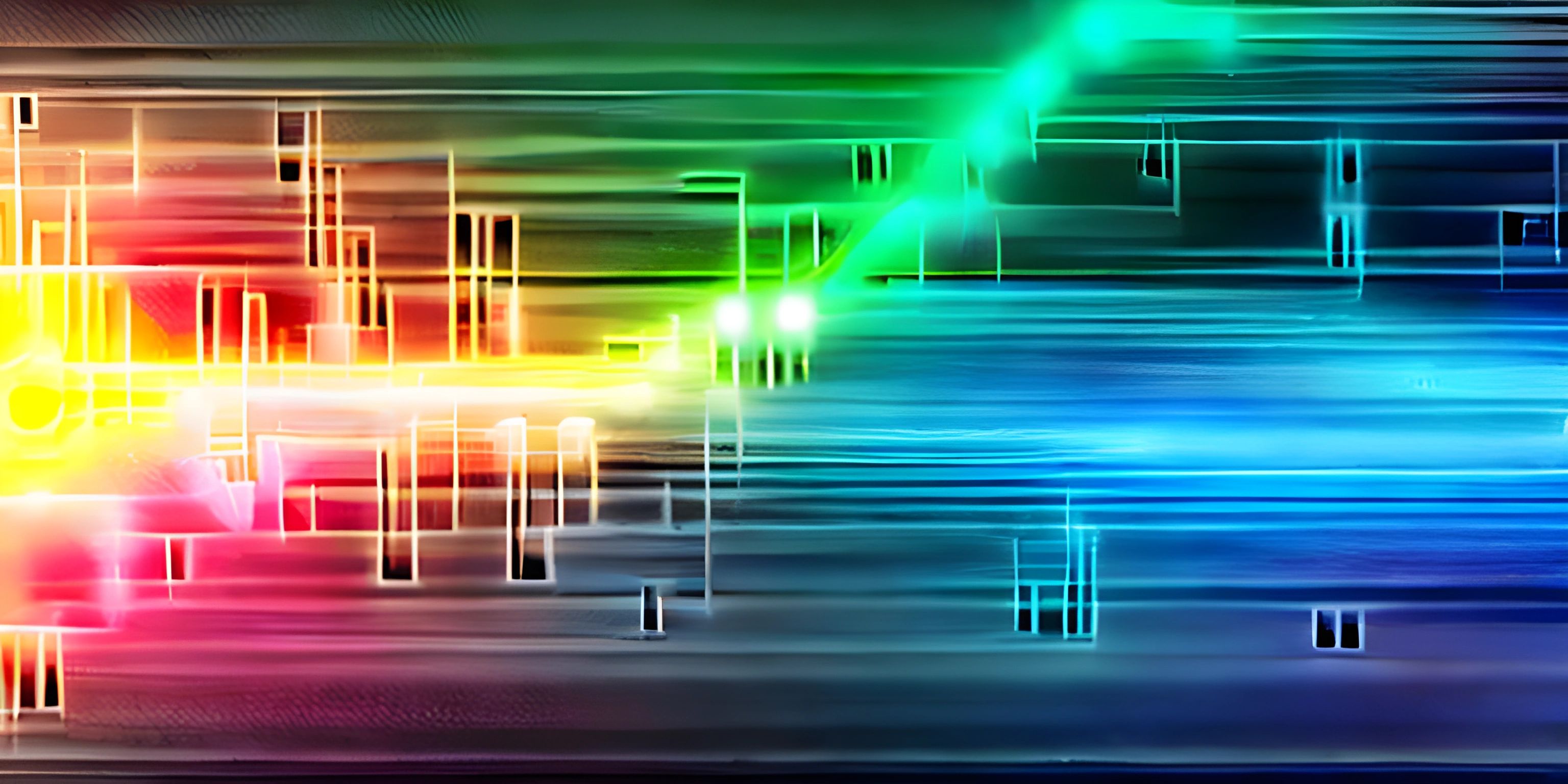
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Algorithms: the mysterious, behind-the-scenes magicians that make your favorite apps run smoothly, calculate your route home, or even help you decide what to binge-watch next on Netflix. But what exactly are they, and why should you care? Let’s dive in and demystify the magical world of algorithms!
What is an Algorithm?
An algorithm is essentially a step-by-step procedure or formula for solving a problem. Think of it as a recipe in a cookbook. Just like a recipe guides you through the process of cooking a delicious meal, an algorithm guides a computer to solve a specific problem or perform a particular task.
Everyday Algorithms
Before we get into the technical nitty-gritty, let's look at some everyday examples of algorithms:
-
Making a Cup of Coffee:
- Boil water.
- Add a spoonful of coffee to a cup.
- Pour boiling water into the cup.
- Stir well.
- Add sugar and milk if desired.
-
Getting Dressed:
- Pick a shirt.
- Pick pants.
- Put on shirt.
- Put on pants.
- Put on shoes and socks.
These examples illustrate the sequential nature of algorithms. Each step must be completed before moving on to the next.
Why Algorithms Matter
In the world of computer science, algorithms are the backbone of all software applications. They enable computers to perform tasks efficiently and effectively. Whether it's sorting a list of numbers, searching for information, or compressing data, there’s an algorithm at work behind the scenes.
Efficiency and Optimization
When we talk about algorithms, efficiency is key. An efficient algorithm performs its task using the least amount of resources possible, like time and memory. Imagine you have a huge list of names and you need to find a specific one. Would you rather:
- Go through the list name by name until you find it?
- Use a more sophisticated method that finds your name faster?
The latter is what efficient algorithms aim to do. They save time and computational power, making programs run faster and more effectively.
Types of Algorithms
Algorithms come in many shapes and sizes, each designed to solve different types of problems. Here are a few common categories:
Sorting Algorithms
Sorting algorithms arrange data in a particular order, such as ascending or descending. Examples include:
- Bubble Sort: Repeatedly swaps adjacent elements if they are in the wrong order.
- Merge Sort: Divides the array into halves, sorts them, and then merges them back together.
- Quick Sort: Picks a pivot element and partitions the array around the pivot.
Search Algorithms
Search algorithms are used to find specific data within a structure. Examples include:
- Linear Search: Goes through each element in the list until it finds the target.
- Binary Search: Divides the sorted list in half to find the target faster.
Graph Algorithms
Graph algorithms deal with graphs (a set of nodes connected by edges). Examples include:
- Dijkstra's Algorithm: Finds the shortest path between nodes in a graph.
- Breadth-First Search (BFS): Explores all nodes at the present depth level before moving on to the nodes at the next depth level.
Cryptographic Algorithms
These algorithms focus on securing data through encryption and decryption. Examples include:
- RSA: A public-key cryptosystem used for secure data transmission.
- AES: A symmetric encryption algorithm used for securing sensitive data.
How Algorithms are Represented
Algorithms can be represented in various ways, including:
Pseudocode
Pseudocode is a high-level description of an algorithm using the conventions of programming languages but designed for human reading. Here’s an example of pseudocode for a simple linear search algorithm:
Algorithm LinearSearch(A, n, x) for i from 0 to n-1 if A[i] = x return i return -1
Flowcharts
Flowcharts are visual diagrams that represent the steps of an algorithm. They use different shapes to denote different types of actions or steps and arrows to show the flow of the process.
Code
Finally, algorithms are often implemented in actual code written in programming languages like Python, Java, or C++. Here’s the linear search algorithm implemented in Python:
def linear_search(arr, target): """ Search for the target in the array. :param arr: list of elements :param target: element to find :return: index of target if found, else -1 """ for i in range(len(arr)): if arr[i] == target: return i return -1 # Example usage array = [1, 2, 3, 4, 5] print(linear_search(array, 3)) # Output: 2
Analyzing Algorithms
When we analyze algorithms, we typically focus on two main aspects:
Time Complexity
Time complexity measures the amount of time an algorithm takes to complete as a function of the input size. It is often expressed using Big O notation, such as O(n), O(log n), or O(n^2). This helps us understand how the algorithm’s performance scales with larger inputs.
Space Complexity
Space complexity measures the amount of memory an algorithm uses in relation to the input size. Like time complexity, it is expressed using Big O notation. Algorithms with lower space complexity are generally preferred, especially when dealing with large datasets.
Practical Applications of Algorithms
Algorithms are everywhere! Here are some practical applications you might encounter daily:
- Search Engines: Use search algorithms to find the most relevant information based on your query.
- Social Media: Use recommendation algorithms to suggest friends, posts, or ads based on your activity.
- Navigation Systems: Use pathfinding algorithms to calculate the shortest or fastest route to your destination.
- E-commerce: Use sorting and filtering algorithms to display products based on your preferences.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).
FAQ
What is an algorithm?
An algorithm is a step-by-step procedure or formula for solving a problem. It acts like a recipe, guiding a computer through a series of actions to perform a specific task or solve a particular problem.
Why are algorithms important in computer science?
Algorithms are the backbone of all software applications. They enable computers to perform tasks efficiently and effectively, saving time and computational power. They are crucial for everything from sorting data to securing information.
What are some common types of algorithms?
Some common types of algorithms include sorting algorithms (like Bubble Sort and Quick Sort), search algorithms (like Linear Search and Binary Search), graph algorithms (like Dijkstra's Algorithm), and cryptographic algorithms (like RSA and AES).
How can algorithms be represented?
Algorithms can be represented in various ways, including pseudocode, flowcharts, and actual code written in programming languages like Python or Java. Each representation helps in understanding and implementing the algorithm.
What is time complexity?
Time complexity measures the amount of time an algorithm takes to complete as a function of the input size. It is often expressed using Big O notation (e.g., O(n), O(log n)), which helps understand how the algorithm’s performance scales with larger inputs.