Exploring Popular Third-Party Libraries for Angular Development
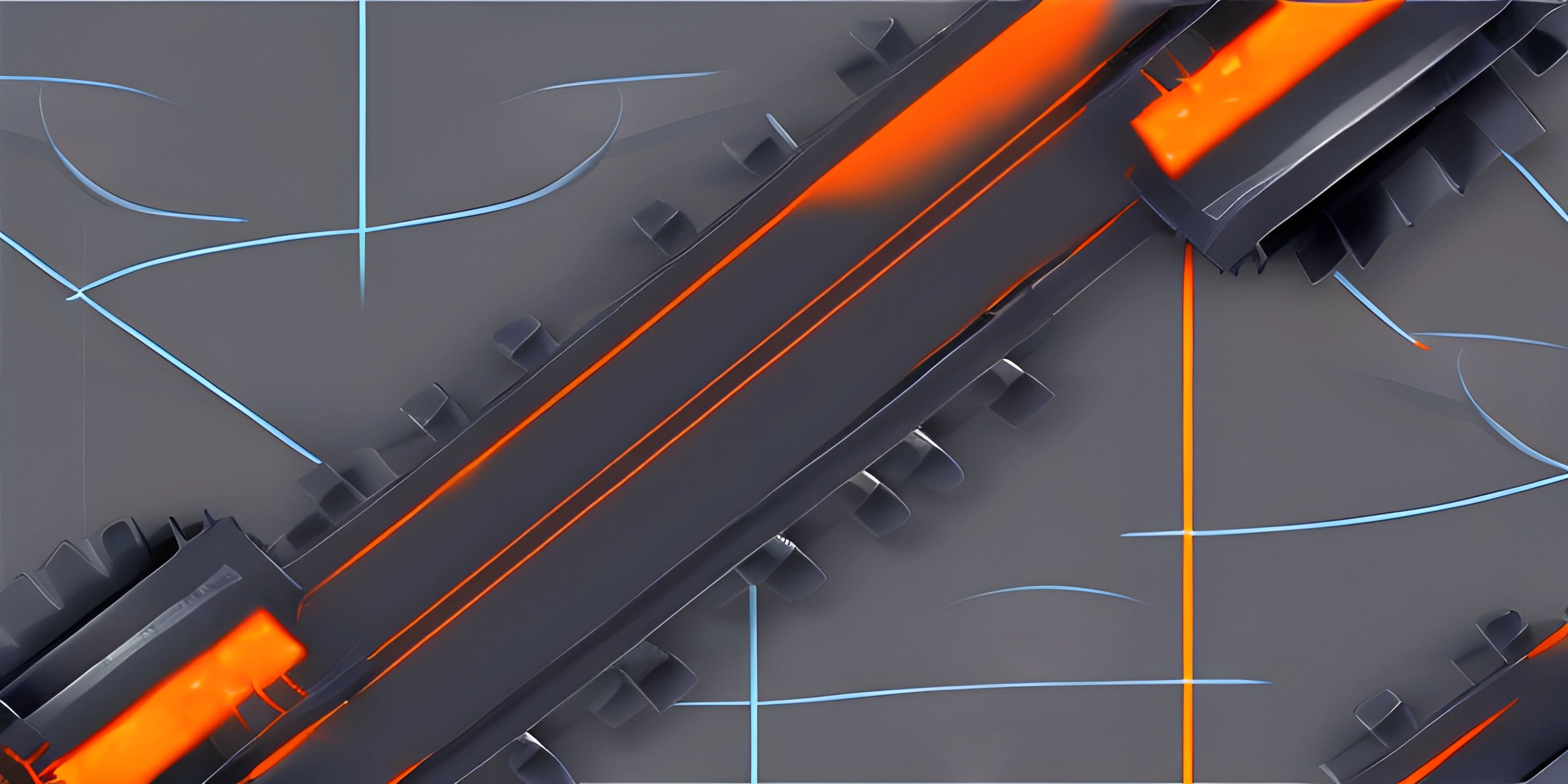
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Angular is a fantastic framework for building web applications, but to make the most of it, developers often turn to third-party libraries. These libraries can help you save time, write cleaner code, and bring additional functionality to your projects. In this article, we'll explore some popular third-party libraries that you can use to supercharge your Angular development.
1. Angular Material
Angular Material is a popular library developed by the Angular team. It provides a collection of Material Design components that are easy to implement and customize. The library includes components for forms, navigation, layout, buttons, and more, allowing you to create a consistent and modern-looking UI for your applications.
Example of importing Angular Material components:
import { MatButtonModule } from "@angular/material/button"; @NgModule({ imports: [MatButtonModule], }) export class AppModule {}
2. NgRx
NgRx is a powerful state management library for Angular applications. It's based on Redux, a popular state management pattern used in many JavaScript applications. NgRx provides a robust and scalable solution to manage your application's state, making it easier to handle complex data flows and asynchronous operations.
Example of creating an NgRx store:
import { StoreModule } from "@ngrx/store"; import { counterReducer } from "./counter.reducer"; @NgModule({ imports: [StoreModule.forRoot({ count: counterReducer })], }) export class AppModule {}
3. NGX-Bootstrap
NGX-Bootstrap is a comprehensive library that provides a set of Bootstrap components for Angular applications. With NGX-Bootstrap, you can quickly build responsive and mobile-first web applications without relying on jQuery. The library includes components for navigation, pagination, modals, tooltips, and more.
Example of importing NGX-Bootstrap components:
import { BsDropdownModule } from "ngx-bootstrap/dropdown"; @NgModule({ imports: [BsDropdownModule.forRoot()], }) export class AppModule {}
4. Angular CLI
While not a library per se, the Angular CLI is an essential tool for Angular developers. It helps you quickly scaffold new projects, generate components, services, and more, as well as run tests and build your applications for production. By automating repetitive tasks, Angular CLI allows you to focus on writing your application logic.
Example of creating a new Angular application using Angular CLI:
ng new my-app
5. PrimeNG
PrimeNG is a rich set of UI components for Angular applications. With over 80 components, from basic input fields to advanced data tables and charts, PrimeNG offers a wide range of building blocks for your applications. The library also comes with a selection of pre-built themes and customization options.
Example of importing PrimeNG components:
import { ButtonModule } from "primeng/button"; @NgModule({ imports: [ButtonModule], }) export class AppModule {}
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Web Frameworks (React) (psst, it's free!).
FAQ
What are some popular third-party libraries for Angular development?
Some popular third-party libraries for Angular development include Angular Material, NgRx, NGX-Bootstrap, Angular CLI, and PrimeNG. These libraries help developers save time, write cleaner code, and bring additional functionality to their projects.
Why should I use third-party libraries in my Angular projects?
Third-party libraries can help you save time and effort by providing pre-built components, patterns, and tools that can be easily integrated into your Angular projects. This allows you to focus on writing your application logic and enhances the overall development experience.
Can you give an example of a third-party library for UI components in Angular?
Angular Material and PrimeNG are examples of third-party libraries that provide a wide range of UI components for Angular applications. These libraries help you create modern and visually appealing user interfaces with ease, without having to write all the code from scratch.