Arrays Explained
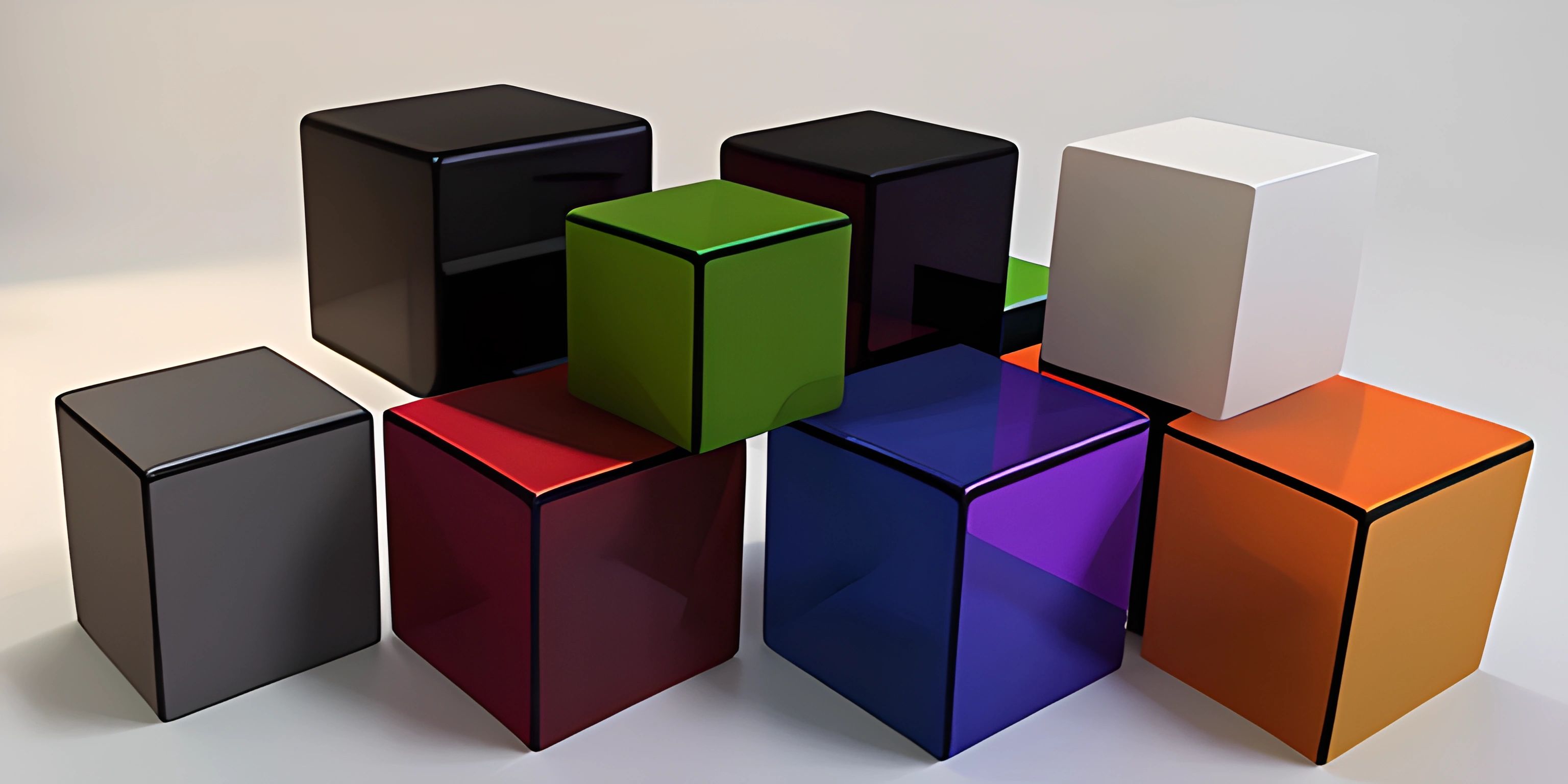
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Arrays are like the Swiss Army knives of data structures: versatile, widely-used, and found in almost every programming language. They provide an efficient way to store and manipulate collections of data. Let's dive into the world of arrays, their properties, and how they're used in different programming languages.
What is an Array?
An array is a collection of elements, where each element can be of any data type (e.g., integer, string, object). The elements are stored in contiguous memory locations, making arrays not only easy to work with but also very efficient. One of the key features of an array is that you can access any element within it using an index (a numerical value that represents the position of the element).
Imagine an array as a row of lockers in a hallway. Each locker has a number (index) and can store an item (element). You can easily access any locker by its number and retrieve or update the item inside.
Creating and Using Arrays
Let's see how to create and use arrays in some popular programming languages.
Python
In Python, arrays are known as lists. Here's how to create a list and access its elements:
my_list = ["apple", "banana", "cherry"] print(my_list[0]) # Output: apple
JavaScript
JavaScript has its own version of arrays, simply called arrays:
const myArray = ["apple", "banana", "cherry"]; console.log(myArray[0]); // Output: apple
Java
Java has a more rigid array structure, with fixed size and data type:
String[] myArray = {"apple", "banana", "cherry"}; System.out.println(myArray[0]); // Output: apple
In each example, we created an array holding three strings and printed the first element using index 0
.
Common Array Operations
Arrays are versatile, and you can perform a variety of operations on them, such as adding, removing, or updating elements. Here are some common operations:
- Accessing elements: Use the index to retrieve or update an element.
- Adding elements: Append new elements to the end, or insert them at a specific position.
- Removing elements: Remove elements by index or by value.
- Searching: Find the index or existence of an element.
- Sorting: Arrange the elements in a specific order (e.g., ascending, descending).
Let's see a quick example in Python:
my_list = [1, 2, 3] my_list.append(4) # Adds 4 to the end => [1, 2, 3, 4] my_list.insert(1, 1.5) # Inserts 1.5 at index 1 => [1, 1.5, 2, 3, 4] my_list.remove(1) # Removes the first occurrence of 1 => [1.5, 2, 3, 4] print(my_list.index(2)) # Output: 1 (Index of element 2)
Array Limitations and Alternatives
While arrays are incredibly useful, they have some limitations:
- Fixed size: In many languages, arrays have a fixed size, making them inflexible.
- Contiguous memory: Arrays require contiguous memory, which can cause issues when dealing with large data sets.
To overcome these limitations, you can use alternative data structures like linked lists, dictionaries, or sets.
Wrapping Up
Arrays are fundamental data structures found in virtually every programming language. They offer an efficient way to store and manipulate collections of data. With their wide range of operations and use cases, arrays are an essential tool in any programmer's toolkit. Now that you're well-acquainted with arrays, you can confidently wield this powerful data structure in your coding adventures!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Palindromes (psst, it's free!).